
for checking multiple SOLID T sensors
Dependencies: DevInterfaces AT30TSE75x I2Cinterfaces
Fork of AT30TSE752TST by
main.cpp
00001 /** example program for the use of the AT30TSE7xx class 00002 * 00003 * check addresses connected, read them 00004 * (C) Wim Beaumont Universiteit Antwerpen 2017 00005 00006 * ver 0.22 updated to the last mbed , see if mbed studio accepts now 00007 */ 00008 00009 #define AT30TSE753EXAMPLEVER "0.22" 00010 00011 #include "mbed.h" 00012 00013 #if defined (TARGET_KL25Z) || defined (TARGET_KL46Z) 00014 PinName const SDA = PTE0; 00015 PinName const SCL = PTE1; 00016 #elif defined (TARGET_KL05Z) 00017 PinName const SDA = PTB4; 00018 PinName const SCL = PTB3; 00019 #elif defined (TARGET_K20D50M) 00020 PinName const SDA = PTB1; 00021 PinName const SCL = PTB0; 00022 #else 00023 #error TARGET NOT DEFINED 00024 #endif 00025 00026 00027 #include "I2C.h" 00028 #include "I2CInterface.h" 00029 #include "MBEDI2CInterface.h" 00030 #include "dev_interface_def.h" 00031 #include "AT30TSE75x.h" 00032 00033 00034 MBEDI2CInterface mbedi2c( SDA, SCL); 00035 MBEDI2CInterface* mbedi2cp= &mbedi2c ; 00036 I2CInterface* i2cdev= mbedi2cp; 00037 00038 Serial pc(USBTX, USBRX); 00039 00040 void print_buf_hex( char *data, int length){ 00041 int nr; 00042 char *ptr=data; 00043 for ( int lc=0; lc < length ; lc++){ 00044 nr= (int) *(ptr++); 00045 printf( "%02x ",nr); 00046 } 00047 printf("\n\r"); 00048 } 00049 00050 00051 int main(void) { 00052 00053 // get the version of getVersion 00054 getVersion gv; 00055 int addr=0; 00056 int i2cerr; 00057 00058 printf("AT30TSE752 example program version %s, compile date %s time %s\n\r",AT30TSE753EXAMPLEVER,__DATE__,__TIME__); 00059 printf("getVersion :%s\n\r ",gv.getversioninfo()); 00060 00061 00062 00063 AT30TSE75x tid[8] ={ AT30TSE75x( i2cdev ,0), AT30TSE75x( i2cdev ,1),AT30TSE75x( i2cdev ,2) ,AT30TSE75x( i2cdev ,3), 00064 AT30TSE75x( i2cdev ,4), AT30TSE75x( i2cdev ,5),AT30TSE75x( i2cdev ,6) ,AT30TSE75x( i2cdev ,7)}; 00065 bool addrfound[8]; 00066 for (int lc=0; lc <7 ;lc++) { 00067 printf ( "AT30SE75x version :%s\n\r ",tid[lc].getversioninfo()); 00068 printf( "Taddr %x , Eaddr %x subaddr %d\n\r ", tid[lc].getTaddr(),tid[lc].getEaddr(), addr); 00069 if( tid[lc].getInitStatus() ){ printf("reading config registers failed \n\r");addrfound[lc]=false; } 00070 else { 00071 addrfound[lc]=true; 00072 tid[lc].set_resolution(12 , i2cerr ); 00073 tid[lc].set_FaultTollerantQueue('6', i2cerr ); 00074 tid[lc].set_AlertPinPolarity(0,i2cerr); 00075 tid[lc].set_AlarmThermostateMode(0,i2cerr); 00076 tid[lc].set_config(i2cerr,0); 00077 int configrd= tid[lc].read_config( i2cerr, 0); 00078 printf( " config %x I2cerr %d \n\r", configrd,i2cerr ); 00079 } 00080 } 00081 int pagenr=0; 00082 const int nrstrs=4; 00083 char str[nrstrs][16]; 00084 00085 while(1) { 00086 for (int lc=0; lc<7 ;lc++) { 00087 if( addrfound[lc]) { 00088 pagenr=0; 00089 for ( int sc=0 ; sc <nrstrs;sc++){ 00090 i2cerr=tid[lc].read_eeprompage(str[sc], 16, 0, (uint8_t) pagenr++); 00091 if(i2cerr) printf("eeprom read error %d addr %d \n\r",i2cerr,lc); 00092 } 00093 00094 for (int sc=0 ; sc < nrstrs;sc ++) { 00095 str[sc][15]='\0'; //make sure it ends 00096 printf("%d:%15s ",sc,str[sc]); 00097 } 00098 // temperature correction 00099 str[3][7]='\0'; 00100 float tempcor=atof(str[3]); 00101 if ( tempcor < -2.5 || tempcor > 2.5) tempcor=0.0; 00102 float Tmp= tid[lc].get_temperature(i2cerr); 00103 printf ("T=%f %f I2cerr %d addr %d\n\r", Tmp,Tmp+tempcor, i2cerr,lc); 00104 } // if 00105 } // for 00106 printf("\n\r"); 00107 wait_ms(500); 00108 } //while 00109 00110 }
Generated on Sun Jul 24 2022 18:18:01 by
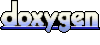