
Microduino
Fork of Io_moon by
Embed:
(wiki syntax)
Show/hide line numbers
BlynkTemplates.h
00001 class BlynkStackOnly 00002 { 00003 protected: 00004 BlynkStackOnly() {} 00005 ~BlynkStackOnly() {} 00006 private: 00007 void* operator new(size_t size); 00008 void operator delete(void *p); 00009 }; 00010 00011 class BlynkNonCopyable 00012 { 00013 protected: 00014 BlynkNonCopyable(){} 00015 ~BlynkNonCopyable(){} 00016 00017 private: 00018 /// @brief Declared as private to prevent usage of copy constructor 00019 BlynkNonCopyable(const BlynkNonCopyable&); 00020 /// @brief Declared as private to prevent usage of assignment operator 00021 BlynkNonCopyable& operator=(const BlynkNonCopyable&); 00022 }; 00023 00024 template<typename T> 00025 class BlynkSingleton: public BlynkNonCopyable 00026 { 00027 public: 00028 /** @brief Returns the instance of the singleton type 00029 When called for the first time, the singleton instance will be 00030 created. All subsequent calls will return a reference to the 00031 previously created instance. 00032 @return The singleton instance 00033 */ 00034 static T* instance() 00035 { 00036 static T instance; 00037 return &instance; 00038 } 00039 protected: 00040 BlynkSingleton() {} 00041 ~BlynkSingleton() {} 00042 }; 00043 00044
Generated on Tue Jul 19 2022 01:01:49 by
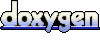