
Phase-shifted PWM demo program
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 // Phase-shifted PWM Test Code 00002 // 00003 // Attempt to run two PWM outputs with 180° phase shifting 00004 // Version x.x 00005 // Created by Wayne Chin 00006 // October 15, 2010 00007 00008 #include "mbed.h" 00009 00010 #define TRUE 1 00011 #define FALSE 0 00012 00013 DigitalOut led1(LED1); 00014 DigitalOut led2(LED2); 00015 DigitalOut led3(LED3); 00016 DigitalOut led4(LED4); 00017 00018 Ticker pwmramp1; 00019 Ticker pwmramp2; 00020 int duty1; // Duty cycle in integer % 00021 int duty2; // Duty cycle in integer % 00022 int PWMclock; // PWM clock = system clock / 4 00023 int PWMrate; // Use to calculate PWM rate (period) 00024 00025 Serial pc(USBTX, USBRX); // tx, rx 00026 00027 void pwmupdate1() 00028 { 00029 int PWMwidth, PWMhalf, PWMrising, PWMfalling; 00030 if (duty1++ > 100) duty1 = 0; // Clamp at 100% pulsewidth 00031 PWMwidth = (duty1 * PWMrate) / 100; 00032 PWMhalf = PWMwidth / 2; 00033 PWMrising = PWMrate - PWMhalf; 00034 PWMfalling = PWMhalf; 00035 LPC_PWM1->MR3 = PWMrising; // Set rising edge 00036 LPC_PWM1->MR4 = PWMfalling; // Set falling edge 00037 LPC_PWM1->LER |= 0x18; // Enable PWM Match 3 & 4 latch 00038 led4 = !led4; 00039 } 00040 00041 void pwmupdate2() 00042 { 00043 int PWMcenter, PWMwidth, PWMhalf, PWMrising, PWMfalling; 00044 if (duty2++ > 100) duty2 = 0; // Clamp at 100% pulsewidth 00045 PWMcenter = PWMrate / 2; 00046 PWMwidth = (duty2 * PWMrate) / 100; 00047 PWMhalf = PWMwidth / 2; 00048 PWMrising = PWMcenter - PWMhalf; 00049 PWMfalling = PWMcenter + PWMhalf; 00050 LPC_PWM1->MR5 = PWMrising; 00051 LPC_PWM1->MR6 = PWMfalling; 00052 LPC_PWM1->LER |= 0x60; // Enable PWM Match 5 & 6 latch 00053 led2 = !led2; 00054 } 00055 00056 int main() 00057 { 00058 /****** Program Starts Here *******/ 00059 pc.baud(19200); 00060 pc.printf("\n\rConnected to mBed...\r\n"); 00061 // Initialize variables 00062 duty1 = 0; 00063 duty2 = 0; 00064 00065 printf("SystemCoreClock = %d Hz\r\n", SystemCoreClock); 00066 // Verify power control for peripherals register 00067 printf("PCLKSEL0: %x\n\r", LPC_SC->PCLKSEL0); // Leave at CCLK/4 00068 PWMclock = SystemCoreClock / 4; 00069 PWMrate = PWMclock / 15000; // 15kHz 00070 printf("PWMrate: %x\n\r", PWMrate); 00071 //printf("PINSEL4: %x\n\r", LPC_PINCON->PINSEL4); // Comes up as GPIO 00072 LPC_PINCON->PINSEL4 |= 0x00000040; // Set P2.3 for PWM1.4 00073 LPC_PINCON->PINSEL4 |= 0x00000400; // Set P2.5 for PWM1.6 00074 printf("PINSEL4: %x\n\r", LPC_PINCON->PINSEL4); 00075 // Set up count control register 00076 printf("PWM1CTCR: %x\n\r", LPC_PWM1->CTCR); // Should already come up in timer mode 00077 // Set up match control register 00078 printf("PWM1MCR: %x\n\r", LPC_PWM1->MCR); 00079 //LPC_PWM1->MCR |= 0x00002000; // Reset PWM4 on match - do not use 00080 //LPC_PWM1->MCR |= 0x00080000; // Reset PWM6 on match - do not use 00081 LPC_PWM1->MCR |= 0x00000002; // Reset PWM0 on match 00082 printf("PWM1MCR: %x\n\r", LPC_PWM1->MCR); 00083 // Set up match registers 00084 LPC_PWM1->MR0 = PWMrate; // Set MR0 (PWM rate) 00085 LPC_PWM1->MR3 = 0x000; // Reset MR3 00086 LPC_PWM1->MR4 = 0x320; // Reset MR4 00087 LPC_PWM1->MR5 = 0x160; // Reset MR5 00088 LPC_PWM1->MR6 = 0x480; // Reset MR6 00089 printf("PWM1MR0,3,4,5,6: %x %x %x %x %x\n\r", LPC_PWM1->MR0, LPC_PWM1->MR3, LPC_PWM1->MR4, LPC_PWM1->MR5, LPC_PWM1->MR6); 00090 // Set up latch enable register 00091 printf("PWM1LER: %x\n\r", LPC_PWM1->LER); 00092 LPC_PWM1->LER |= 0x08; // Enable PWM Match 3 latch 00093 LPC_PWM1->LER |= 0x10; // Enable PWM Match 4 latch 00094 LPC_PWM1->LER |= 0x20; // Enable PWM Match 5 latch 00095 LPC_PWM1->LER |= 0x40; // Enable PWM Match 6 latch 00096 printf("PWM1LER: %x\n\r", LPC_PWM1->LER); 00097 // Set up PWM control register 00098 printf("PWM1PCR: %x\n\r", LPC_PWM1->PCR); 00099 LPC_PWM1->PCR |= 0x00000010; // Select double edge PWM for PWM4 00100 LPC_PWM1->PCR |= 0x00000040; // Select double edge PWM for PWM6 00101 LPC_PWM1->PCR |= 0x00001000; // Enable PWM4 00102 LPC_PWM1->PCR |= 0x00004000; // Enable PWM6 00103 printf("PWM1PCR: %x\n\r", LPC_PWM1->PCR); 00104 // Set up timer control register 00105 printf("PWM1TCR: %x\n\r", LPC_PWM1->TCR); 00106 LPC_PWM1->TCR |= 0x08; // Enable PWM mode 00107 LPC_PWM1->TCR |= 0x01; // Enable counter 00108 printf("PWM1TCR: %x\n\r", LPC_PWM1->TCR); 00109 00110 // Set up timeout calls 00111 pwmramp1.attach_us(&pwmupdate1, 250000); // setup pwmramp1 to call pwmupdate after 250 ms 00112 pwmramp2.attach_us(&pwmupdate2, 500000); // setup pwmramp2 to call pwmupdate after 500 ms 00113 00114 while (1) 00115 { 00116 00117 }//while 00118 }//main
Generated on Sat Jul 16 2022 05:06:36 by
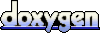