
Start van regelaar
Dependencies: Encoder MODSERIAL mbed
Dependents: K9motoraansturing_copy lichtpoortjes
main.cpp
00001 #include "mbed.h" 00002 #include "encoder.h" 00003 #include "MODSERIAL.h" 00004 00005 /******************************************************************************* 00006 * * 00007 * Code can be found at http://mbed.org/users/vsluiter/code/BMT-K9-Regelaar/ * 00008 * * 00009 ********************************************************************************/ 00010 00011 /** keep_in_range -> float in, and keep_in_range if less than min, or larger than max **/ 00012 void keep_in_range(float * in, float min, float max); 00013 00014 /** variable to show when a new loop can be started*/ 00015 /** volatile means that it can be changed in an */ 00016 /** interrupt routine, and that that change is vis-*/ 00017 /** ible in the main loop. */ 00018 00019 volatile bool looptimerflag; 00020 00021 /** function called by Ticker "looptimer" */ 00022 /** variable 'looptimerflag' is set to 'true' */ 00023 /** each time the looptimer expires. */ 00024 void setlooptimerflag(void) 00025 { 00026 looptimerflag = true; 00027 } 00028 00029 00030 int main() { 00031 //LOCAL VARIABLES 00032 /*Potmeter input*/ 00033 AnalogIn potmeter(PTC2); 00034 /* Encoder, using my encoder library */ 00035 /* First pin should be PTDx or PTAx */ 00036 /* because those pins can be used as */ 00037 /* InterruptIn */ 00038 Encoder motor1(PTD0,PTC9); 00039 /* MODSERIAL to get non-blocking Serial*/ 00040 MODSERIAL pc(USBTX,USBRX); 00041 /* PWM control to motor */ 00042 PwmOut pwm_motor(PTA12); 00043 /* Direction pin */ 00044 DigitalOut motordir(PTD3); 00045 /* variable to store setpoint in */ 00046 float setpoint; 00047 /* variable to store pwm value in*/ 00048 float pwm_to_motor; 00049 //START OF CODE 00050 00051 /*Set the baudrate (use this number in RealTerm too! */ 00052 pc.baud(230400); 00053 00054 /*Create a ticker, and let it call the */ 00055 /*function 'setlooptimerflag' every 0.01s */ 00056 Ticker looptimer; 00057 looptimer.attach(setlooptimerflag,0.01); 00058 /* Oops... I left some test code in my program */ 00059 pc.printf("bla"); 00060 00061 //INFINITE LOOP 00062 while(1) { 00063 /* Wait until looptimer flag is true. */ 00064 /* '!=' means not-is-equal */ 00065 while(looptimerflag != true); 00066 /* Clear the looptimerflag, otherwise */ 00067 /* the program would simply continue */ 00068 /* without waitingin the next iteration*/ 00069 looptimerflag = false; 00070 00071 /* Read potmeter value, apply some math */ 00072 /* to get useful setpoint value */ 00073 setpoint = (potmeter.read()-0.5)*2000; 00074 00075 /* Print setpoint and current position to serial terminal*/ 00076 pc.printf("s: %f, %d \n\r", setpoint, motor1.getPosition()); 00077 00078 /* This is a P-action! calculate error, multiply with gain, and store in pwm_to_motor */ 00079 pwm_to_motor = (setpoint - motor1.getPosition())*.001; 00080 /* Coerce pwm value if outside range */ 00081 /* Not strictly needed here, but useful */ 00082 /* if doing other calculations with pwm value */ 00083 keep_in_range(&pwm_to_motor, -1,1); 00084 00085 /* Control the motor direction pin. based on */ 00086 /* the sign of your pwm value. If your */ 00087 /* motor keeps spinning when running this code */ 00088 /* you probably need to swap the motor wires, */ 00089 /* or swap the 'write(1)' and 'write(0)' below */ 00090 if(pwm_to_motor > 0) 00091 motordir.write(1); 00092 else 00093 motordir.write(0); 00094 //WRITE VALUE TO MOTOR 00095 /* Take the absolute value of the PWM to send */ 00096 /* to the motor. */ 00097 pwm_motor.write(abs(pwm_to_motor)); 00098 } 00099 } 00100 00101 00102 //coerces value 'in' to min or max when exceeding those values 00103 //if you'd like to understand the statement below take a google for 00104 //'ternary operators'. 00105 void keep_in_range(float * in, float min, float max) 00106 { 00107 *in > min ? *in < max? : *in = max: *in = min; 00108 } 00109 00110 00111
Generated on Tue Jul 12 2022 23:21:09 by
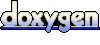