Blynk example, for all boards using plain Serial/USB connection
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /************************************************************* 00002 Blynk is a platform with iOS and Android apps to control 00003 Arduino, Raspberry Pi and the likes over the Internet. 00004 You can easily build graphic interfaces for all your 00005 projects by simply dragging and dropping widgets. 00006 00007 Downloads, docs, tutorials: http://www.blynk.cc 00008 Sketch generator: http://examples.blynk.cc 00009 Blynk community: http://community.blynk.cc 00010 Follow us: http://www.fb.com/blynkapp 00011 http://twitter.com/blynk_app 00012 00013 Blynk library is licensed under MIT license 00014 This example code is in public domain. 00015 00016 ************************************************************* 00017 This example shows how to use Serial/USB 00018 to connect your project to Blynk. 00019 00020 *************************************************************/ 00021 #include "mbed.h" 00022 00023 // Define your serial for console logs: 00024 Serial pc(p9, p10); 00025 00026 // Define your serial for data: 00027 Serial serial(USBTX, USBRX); 00028 00029 //#define BLYNK_DEBUG 00030 #define BLYNK_PRINT pc 00031 #include <Blynk/BlynkSimpleSerial.h> 00032 00033 // You should get Auth Token in the Blynk App. 00034 // Go to the Project Settings (nut icon). 00035 char auth[] = "YourAuthToken"; 00036 00037 DigitalOut led(LED1); 00038 00039 BLYNK_WRITE(V1) { 00040 led = !led; 00041 Blynk.virtualWrite(V2, BlynkMillis()/1000); 00042 } 00043 00044 void setup() 00045 { 00046 pc.baud(115200); 00047 00048 serial.baud(115200); 00049 00050 Blynk.begin(serial, auth); 00051 } 00052 00053 void loop() 00054 { 00055 Blynk.run(); 00056 } 00057 00058 int main() { 00059 setup(); 00060 while(1) { 00061 loop(); 00062 } 00063 }
Generated on Fri Jul 22 2022 22:12:30 by
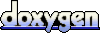