Example for BBC Micro:bit
Fork of microbit-samples by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /************************************************************* 00002 Blynk is a platform with iOS and Android apps to control 00003 Arduino, Raspberry Pi and the likes over the Internet. 00004 You can easily build graphic interfaces for all your 00005 projects by simply dragging and dropping widgets. 00006 00007 Downloads, docs, tutorials: http://www.blynk.cc 00008 Sketch generator: http://examples.blynk.cc 00009 Blynk community: http://community.blynk.cc 00010 Follow us: http://www.fb.com/blynkapp 00011 http://twitter.com/blynk_app 00012 00013 Blynk library is licensed under MIT license 00014 This example code is in public domain. 00015 00016 ************************************************************* 00017 NOTE: Please set this config in your MicroBitConfig.h: 00018 #define MICROBIT_BLE_ENABLED 1 00019 #define MICROBIT_BLE_PAIRING_MODE 0 00020 #define MICROBIT_BLE_OPEN 1 00021 00022 Optionally, you can disable unused services: 00023 #define MICROBIT_BLE_DFU_SERVICE 0 00024 #define MICROBIT_BLE_EVENT_SERVICE 0 00025 00026 Warning: Bluetooth support is in beta! 00027 00028 *************************************************************/ 00029 #include <MicroBit.h> 00030 MicroBit uBit; 00031 00032 //#define BLYNK_DEBUG 00033 #define BLYNK_PRINT uBit.serial 00034 00035 #include <BlynkSimpleMicroBit.h> 00036 00037 // You should get Auth Token in the Blynk App. 00038 // Go to the Project Settings (nut icon). 00039 char auth[] = "YourAuthToken"; 00040 00041 00042 void onConnected(MicroBitEvent e) 00043 { 00044 uBit.display.printChar('+', 500); 00045 Blynk.startSession(); 00046 } 00047 00048 void onDisconnected(MicroBitEvent e) 00049 { 00050 uBit.display.print("- - -", 100); 00051 Blynk.disconnect(); 00052 } 00053 00054 int counter = 0; 00055 00056 void onPressed(MicroBitEvent e) 00057 { 00058 char buf[8]; 00059 sprintf(buf, "%d", counter); 00060 00061 Blynk.virtualWrite(V1, buf); 00062 uBit.serial.printf("%s\n", buf); 00063 uBit.display.scroll(buf); 00064 00065 counter++; 00066 } 00067 00068 void setup() { 00069 // Initialise the micro:bit runtime. 00070 uBit.init(); 00071 uBit.display.scrollAsync("BLYNK", 100); 00072 00073 // listen for Bluetooth connection state changes 00074 uBit.messageBus.listen(MICROBIT_ID_BLE, MICROBIT_BLE_EVT_CONNECTED, onConnected); 00075 uBit.messageBus.listen(MICROBIT_ID_BLE, MICROBIT_BLE_EVT_DISCONNECTED, onDisconnected); 00076 00077 // 00078 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_CLICK, onPressed); 00079 00080 // Add Blynk service 00081 Blynk.begin(*uBit.ble, auth); 00082 } 00083 00084 void loop() { 00085 Blynk.run(); 00086 } 00087 00088 int main(void) { 00089 setup(); 00090 while(1) { 00091 loop(); 00092 uBit.sleep(10); 00093 } 00094 }
Generated on Sat Jul 16 2022 17:49:15 by
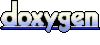