
Fork of my original MQTTGateway
Embed:
(wiki syntax)
Show/hide line numbers
fsfat_test.h
Go to the documentation of this file.
00001 /** @file fsfat_test.h 00002 * 00003 * mbed Microcontroller Library 00004 * Copyright (c) 2006-2016 ARM Limited 00005 * 00006 * Licensed under the Apache License, Version 2.0 (the "License"); 00007 * you may not use this file except in compliance with the License. 00008 * You may obtain a copy of the License at 00009 * 00010 * http://www.apache.org/licenses/LICENSE-2.0 00011 * 00012 * Unless required by applicable law or agreed to in writing, software 00013 * distributed under the License is distributed on an "AS IS" BASIS, 00014 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00015 * See the License for the specific language governing permissions and 00016 * limitations under the License. 00017 * 00018 * Header file for test support data structures and function API. 00019 */ 00020 #ifndef __FSFAT_TEST_H 00021 #define __FSFAT_TEST_H 00022 00023 #include <stdint.h> 00024 #include <stdlib.h> 00025 #include <stdbool.h> 00026 00027 #ifdef __cplusplus 00028 extern "C" { 00029 #endif 00030 00031 /* Defines */ 00032 //#define FSFAT_INIT_1_TABLE_HEAD { "a", ""} 00033 #define FSFAT_INIT_1_TABLE_MID_NODE { "/sd/01234567.txt", "abcdefghijklmnopqrstuvwxyz"} 00034 //#define FSFAT_INIT_1_TABLE_TAIL { "/sd/fopentst/hello/world/animal/wobbly/dog/foot/backrght.txt", "present"} 00035 #define FSFAT_TEST_RW_TABLE_SENTINEL 0xffffffff 00036 #define FSFAT_TEST_BYTE_DATA_TABLE_SIZE 256 00037 #define FSFAT_UTEST_MSG_BUF_SIZE 256 00038 #define FSFAT_UTEST_DEFAULT_TIMEOUT_MS 10000 00039 #define FSFAT_MBED_HOSTTEST_TIMEOUT 60 00040 #define FSFAT_MAX_FILE_BASENAME 8 00041 #define FSFAT_MAX_FILE_EXTNAME 3 00042 #define FSFAT_BUF_MAX_LENGTH 64 00043 #define FSFAT_FILENAME_MAX_LENGTH 255 00044 00045 00046 /* support macro for make string for utest _MESSAGE macros, which dont support formatted output */ 00047 #define FSFAT_TEST_UTEST_MESSAGE(_buf, _max_len, _fmt, ...) \ 00048 do \ 00049 { \ 00050 snprintf((_buf), (_max_len), (_fmt), __VA_ARGS__); \ 00051 }while(0); 00052 00053 00054 /* 00055 * Structures 00056 */ 00057 00058 /* kv data for test */ 00059 typedef struct fsfat_kv_data_t { 00060 const char* filename; 00061 const char* value; 00062 } fsfat_kv_data_t; 00063 00064 00065 extern const uint8_t fsfat_test_byte_data_table[FSFAT_TEST_BYTE_DATA_TABLE_SIZE]; 00066 00067 int32_t fsfat_test_create(const char* filename, const char* data, size_t len); 00068 int32_t fsfat_test_delete(const char* key_name); 00069 int32_t fsfat_test_filename_gen(char* name, const size_t len); 00070 #ifdef __cplusplus 00071 } 00072 #endif 00073 00074 #endif /* __FSFAT_TEST_H */
Generated on Tue Jul 12 2022 18:06:46 by
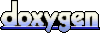