
Fork of my original MQTTGateway
Embed:
(wiki syntax)
Show/hide line numbers
RadioCfg.cpp
00001 #include "RadioCfg.h" 00002 #include <string.h> 00003 #include <stdlib.h> 00004 00005 static char tempstr[100]; 00006 00007 static int jsoneq(const char * json, jsmntok_t * tok, const char * s) 00008 { 00009 if (tok->type == JSMN_STRING && (int) strlen(s) == tok->end - tok->start && 00010 strncmp(json + tok->start, s, tok->end - tok->start) == 0) { 00011 return 0; 00012 } 00013 return -1; 00014 } 00015 00016 int parseradioconfig(const char * jsonstring, RadioCfg & radiocfg) 00017 { 00018 int i, r; 00019 00020 jsmn_parser p; 00021 jsmntok_t t[128]; 00022 00023 jsmn_init(&p); 00024 r = jsmn_parse(&p, jsonstring, strlen(jsonstring), t, sizeof(t)/sizeof(t[0])); 00025 if ( r < 0 ) 00026 return -1; 00027 00028 /* Top level element is an object */ 00029 if (r < 1 || t[0].type != JSMN_OBJECT) 00030 { 00031 return -1; 00032 } 00033 00034 /* Loop over all tokens */ 00035 for (i = 1; i < r; i++) 00036 { 00037 if (jsoneq(jsonstring, &t[i], "error") == 0) 00038 { 00039 // Query returned an error ... 00040 return -1; 00041 } 00042 else if (jsoneq(jsonstring, &t[i], "id") == 0) 00043 { 00044 memset(&tempstr[0], 0, sizeof(tempstr)); 00045 strncpy(&tempstr[0], jsonstring + t[i+1].start, (t[i+1].end - t[i+1].start)); 00046 // Store the radio id ... 00047 radiocfg.radioID = strtoull(tempstr, NULL, 0); 00048 i++; 00049 } 00050 else if (jsoneq(jsonstring, &t[i], "name") == 0) 00051 { 00052 memset(&radiocfg.name[0], 0, RADIO_NAME_LEN); 00053 strncpy(&radiocfg.name[0], jsonstring + t[i+1].start, (t[i+1].end - t[i+1].start)); 00054 i++; 00055 } 00056 else if (jsoneq(jsonstring, &t[i], "country") == 0) 00057 { 00058 memset(&radiocfg.country[0], 0, RADIO_NAME_LEN); 00059 strncpy(&radiocfg.country[0], jsonstring + t[i+1].start, (t[i+1].end - t[i+1].start)); 00060 i++; 00061 } 00062 else if (jsoneq(jsonstring, &t[i], "utc_offset") == 0) 00063 { 00064 memset(&tempstr[0], 0, sizeof(tempstr)); 00065 strncpy(&tempstr[0], jsonstring + t[i+1].start, (t[i+1].end - t[i+1].start)); 00066 // Store the radio id ... 00067 radiocfg.utc_offset = strtoul(&tempstr[0], NULL, 0); 00068 i++; 00069 } 00070 else if (jsoneq(jsonstring, &t[i], "radios") == 0) 00071 { 00072 int j; 00073 // Radios is an array of radio objects 00074 if (t[i+1].type != JSMN_ARRAY){ 00075 continue; 00076 } 00077 00078 int arrsize = t[i+1].size; 00079 // Move to the start of the array 00080 i += 2; 00081 for (j = 0; j < arrsize; j++) 00082 { 00083 RadioSlave slv; 00084 int r = t[i + j].size; 00085 //jsmntok_t * g = &t[i + j]; 00086 //printf(" * %.*s\n", g->end - g->start, jsonstring + g->start); 00087 // Enumerate objects within the array 00088 for (int z = 0; z < r ; z++) 00089 { 00090 00091 if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "id") == 0) 00092 { 00093 jsmntok_t * o = &t[i+j+(z*2)+2]; 00094 memset(&tempstr[0], 0, sizeof(tempstr)); 00095 strncpy(&tempstr[0], jsonstring + o->start, o->end - o->start); 00096 slv.radioID = strtoull(&tempstr[0], NULL, 0); 00097 } 00098 else if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "name") == 0) 00099 { 00100 jsmntok_t * o = &t[i+j+(z*2)+2]; 00101 memset(&slv.name[0], 0, RADIO_NAME_LEN); 00102 strncpy(&slv.name[0], jsonstring + o->start, o->end - o->start); 00103 } 00104 if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "temp_pin") == 0) 00105 { 00106 jsmntok_t * o = &t[i+j+(z*2)+2]; 00107 memset(&tempstr[0], 0, sizeof(tempstr)); 00108 strncpy(&tempstr[0], jsonstring + o->start, o->end - o->start); 00109 slv.temp_pin = strtoul(&tempstr[0], NULL, 0); 00110 } 00111 if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "humid_pin") == 0) 00112 { 00113 jsmntok_t * o = &t[i+j+(z*2)+2]; 00114 memset(&tempstr[0], 0, sizeof(tempstr)); 00115 strncpy(&tempstr[0], jsonstring + o->start, o->end - o->start); 00116 slv.humid_pin = strtoul(&tempstr[0], NULL, 0); 00117 } 00118 if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "lumin_pin") == 0) 00119 { 00120 jsmntok_t * o = &t[i+j+(z*2)+2]; 00121 memset(&tempstr[0], 0, sizeof(tempstr)); 00122 strncpy(&tempstr[0], jsonstring + o->start, o->end - o->start); 00123 slv.lumin_pin = strtoul(&tempstr[0], NULL, 0); 00124 } 00125 if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "spinkler_pin") == 0) 00126 { 00127 jsmntok_t * o = &t[i+j+(z*2)+2]; 00128 memset(&tempstr[0], 0, sizeof(tempstr)); 00129 strncpy(&tempstr[0], jsonstring + o->start, o->end - o->start); 00130 slv.sprinkler_pin = strtoul(&tempstr[0], NULL, 0); 00131 } 00132 if (jsoneq(jsonstring, &t[i+j+(z*2)+1], "hthreshold") == 0) 00133 { 00134 jsmntok_t * o = &t[i+j+(z*2)+2]; 00135 memset(&tempstr[0], 0, sizeof(tempstr)); 00136 strncpy(&tempstr[0], jsonstring + o->start, o->end - o->start); 00137 slv.hthreshold = strtoul(&tempstr[0], NULL, 0); 00138 } 00139 else 00140 { 00141 // TODO: 00142 } 00143 } 00144 // Add the radio slave to the vector 00145 radiocfg.radios.push_back(slv); 00146 i += (t[i+j].size) * 2; 00147 } 00148 // Move to the end of the object 00149 i += 4; 00150 }else 00151 { 00152 00153 } 00154 } 00155 00156 return 0; 00157 }
Generated on Tue Jul 12 2022 18:06:46 by
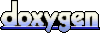