
Fork of my original MQTTGateway
Embed:
(wiki syntax)
Show/hide line numbers
MQTTSubscribeServer.c
00001 /******************************************************************************* 00002 * Copyright (c) 2014 IBM Corp. 00003 * 00004 * All rights reserved. This program and the accompanying materials 00005 * are made available under the terms of the Eclipse Public License v1.0 00006 * and Eclipse Distribution License v1.0 which accompany this distribution. 00007 * 00008 * The Eclipse Public License is available at 00009 * http://www.eclipse.org/legal/epl-v10.html 00010 * and the Eclipse Distribution License is available at 00011 * http://www.eclipse.org/org/documents/edl-v10.php. 00012 * 00013 * Contributors: 00014 * Ian Craggs - initial API and implementation and/or initial documentation 00015 *******************************************************************************/ 00016 00017 #include "MQTTPacket.h" 00018 #include "StackTrace.h" 00019 00020 #include <string.h> 00021 00022 00023 /** 00024 * Deserializes the supplied (wire) buffer into subscribe data 00025 * @param dup integer returned - the MQTT dup flag 00026 * @param packetid integer returned - the MQTT packet identifier 00027 * @param maxcount - the maximum number of members allowed in the topicFilters and requestedQoSs arrays 00028 * @param count - number of members in the topicFilters and requestedQoSs arrays 00029 * @param topicFilters - array of topic filter names 00030 * @param requestedQoSs - array of requested QoS 00031 * @param buf the raw buffer data, of the correct length determined by the remaining length field 00032 * @param buflen the length in bytes of the data in the supplied buffer 00033 * @return the length of the serialized data. <= 0 indicates error 00034 */ 00035 int MQTTDeserialize_subscribe(unsigned char* dup, unsigned short* packetid, int maxcount, int* count, MQTTString topicFilters[], 00036 int requestedQoSs[], unsigned char* buf, int buflen) 00037 { 00038 MQTTHeader header = {0}; 00039 unsigned char* curdata = buf; 00040 unsigned char* enddata = NULL; 00041 int rc = -1; 00042 int mylen = 0; 00043 00044 FUNC_ENTRY; 00045 header.byte = readChar(&curdata); 00046 if (header.bits.type != SUBSCRIBE) 00047 goto exit; 00048 *dup = header.bits.dup; 00049 00050 curdata += (rc = MQTTPacket_decodeBuf(curdata, &mylen)); /* read remaining length */ 00051 enddata = curdata + mylen; 00052 00053 *packetid = readInt(&curdata); 00054 00055 *count = 0; 00056 while (curdata < enddata) 00057 { 00058 if (!readMQTTLenString(&topicFilters[*count], &curdata, enddata)) 00059 goto exit; 00060 if (curdata >= enddata) /* do we have enough data to read the req_qos version byte? */ 00061 goto exit; 00062 requestedQoSs[*count] = readChar(&curdata); 00063 (*count)++; 00064 } 00065 00066 rc = 1; 00067 exit: 00068 FUNC_EXIT_RC(rc); 00069 return rc; 00070 } 00071 00072 00073 /** 00074 * Serializes the supplied suback data into the supplied buffer, ready for sending 00075 * @param buf the buffer into which the packet will be serialized 00076 * @param buflen the length in bytes of the supplied buffer 00077 * @param packetid integer - the MQTT packet identifier 00078 * @param count - number of members in the grantedQoSs array 00079 * @param grantedQoSs - array of granted QoS 00080 * @return the length of the serialized data. <= 0 indicates error 00081 */ 00082 int MQTTSerialize_suback(unsigned char* buf, int buflen, unsigned short packetid, int count, int* grantedQoSs) 00083 { 00084 MQTTHeader header = {0}; 00085 int rc = -1; 00086 unsigned char *ptr = buf; 00087 int i; 00088 00089 FUNC_ENTRY; 00090 if (buflen < 2 + count) 00091 { 00092 rc = MQTTPACKET_BUFFER_TOO_SHORT; 00093 goto exit; 00094 } 00095 header.byte = 0; 00096 header.bits.type = SUBACK; 00097 writeChar(&ptr, header.byte); /* write header */ 00098 00099 ptr += MQTTPacket_encode(ptr, 2 + count); /* write remaining length */ 00100 00101 writeInt(&ptr, packetid); 00102 00103 for (i = 0; i < count; ++i) 00104 writeChar(&ptr, grantedQoSs[i]); 00105 00106 rc = ptr - buf; 00107 exit: 00108 FUNC_EXIT_RC(rc); 00109 return rc; 00110 } 00111 00112
Generated on Tue Jul 12 2022 18:06:46 by
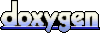