
Fork of my original MQTTGateway
Embed:
(wiki syntax)
Show/hide line numbers
FP.h
Go to the documentation of this file.
00001 /** 00002 * @file FP.h 00003 * @brief Core Utility - Templated Function Pointer Class 00004 * @author sam grove 00005 * @version 1.1 00006 * @see http://mbed.org/users/sam_grove/code/FP/ 00007 * 00008 * Copyright (c) 2013 00009 * 00010 * Licensed under the Apache License, Version 2.0 (the "License"); 00011 * you may not use this file except in compliance with the License. 00012 * You may obtain a copy of the License at 00013 * 00014 * http://www.apache.org/licenses/LICENSE-2.0 00015 * 00016 * Unless required by applicable law or agreed to in writing, software 00017 * distributed under the License is distributed on an "AS IS" BASIS, 00018 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00019 * See the License for the specific language governing permissions and 00020 * limitations under the License. 00021 */ 00022 00023 #ifndef FP_H 00024 #define FP_H 00025 00026 /** Example using the FP Class with global functions 00027 * @code 00028 * #include "mbed.h" 00029 * #include "FP.h" 00030 * 00031 * FP<void,bool>fp; 00032 * DigitalOut myled(LED1); 00033 * 00034 * void handler(bool value) 00035 * { 00036 * myled = value; 00037 * return; 00038 * } 00039 * 00040 * int main() 00041 * { 00042 * fp.attach(&handler); 00043 * 00044 * while(1) 00045 * { 00046 * fp(1); 00047 * wait(0.2); 00048 * fp(0); 00049 * wait(0.2); 00050 * } 00051 * } 00052 * @endcode 00053 */ 00054 00055 /** Example using the FP Class with different class member functions 00056 * @code 00057 * #include "mbed.h" 00058 * #include "FP.h" 00059 * 00060 * FP<void,bool>fp; 00061 * DigitalOut myled(LED4); 00062 * 00063 * class Wrapper 00064 * { 00065 * public: 00066 * Wrapper(){} 00067 * 00068 * void handler(bool value) 00069 * { 00070 * myled = value; 00071 * return; 00072 * } 00073 * }; 00074 * 00075 * int main() 00076 * { 00077 * Wrapper wrapped; 00078 * fp.attach(&wrapped, &Wrapper::handler); 00079 * 00080 * while(1) 00081 * { 00082 * fp(1); 00083 * wait(0.2); 00084 * fp(0); 00085 * wait(0.2); 00086 * } 00087 * } 00088 * @endcode 00089 */ 00090 00091 /** Example using the FP Class with member FP and member function 00092 * @code 00093 * #include "mbed.h" 00094 * #include "FP.h" 00095 * 00096 * DigitalOut myled(LED2); 00097 * 00098 * class Wrapper 00099 * { 00100 * public: 00101 * Wrapper() 00102 * { 00103 * fp.attach(this, &Wrapper::handler); 00104 * } 00105 * 00106 * void handler(bool value) 00107 * { 00108 * myled = value; 00109 * return; 00110 * } 00111 * 00112 * FP<void,bool>fp; 00113 * }; 00114 * 00115 * int main() 00116 * { 00117 * Wrapper wrapped; 00118 * 00119 * while(1) 00120 * { 00121 * wrapped.fp(1); 00122 * wait(0.2); 00123 * wrapped.fp(0); 00124 * wait(0.2); 00125 * } 00126 * } 00127 * @endcode 00128 */ 00129 00130 /** 00131 * @class FP 00132 * @brief API for managing Function Pointers 00133 */ 00134 template<class retT, class argT> 00135 class FP 00136 { 00137 public: 00138 /** Create the FP object - only one callback can be attached to the object, that is 00139 * a member function or a global function, not both at the same time 00140 */ 00141 FP() 00142 { 00143 obj_callback = 0; 00144 c_callback = 0; 00145 } 00146 00147 /** Add a callback function to the object 00148 * @param item - Address of the initialized object 00149 * @param member - Address of the member function (dont forget the scope that the function is defined in) 00150 */ 00151 template<class T> 00152 void attach(T *item, retT (T::*method)(argT)) 00153 { 00154 obj_callback = (FPtrDummy *)(item); 00155 method_callback = (retT (FPtrDummy::*)(argT))(method); 00156 return; 00157 } 00158 00159 /** Add a callback function to the object 00160 * @param function - The address of a globally defined function 00161 */ 00162 void attach(retT (*function)(argT)) 00163 { 00164 c_callback = function; 00165 } 00166 00167 /** Invoke the function attached to the class 00168 * @param arg - An argument that is passed into the function handler that is called 00169 * @return The return from the function hanlder called by this class 00170 */ 00171 retT operator()(argT arg) const 00172 { 00173 if( 0 != c_callback ) { 00174 return obj_callback ? (obj_callback->*method_callback )(arg) : (*c_callback)(arg); 00175 } 00176 return (retT)0; 00177 } 00178 00179 /** Determine if an callback is currently hooked 00180 * @return 1 if a method is hooked, 0 otherwise 00181 */ 00182 bool attached() 00183 { 00184 return obj_callback || c_callback ; 00185 } 00186 00187 /** Release a function from the callback hook 00188 */ 00189 void detach() 00190 { 00191 obj_callback = 0; 00192 c_callback = 0; 00193 } 00194 00195 private: 00196 00197 // empty type used for casting 00198 class FPtrDummy; 00199 00200 FPtrDummy *obj_callback; 00201 00202 /** 00203 * @union Funciton 00204 * @brief Member or global callback function 00205 */ 00206 union { 00207 retT (*c_callback )(argT); /*!< Footprint for a global function */ 00208 retT (FPtrDummy::*method_callback )(argT); /*!< Footprint for a member function */ 00209 }; 00210 }; 00211 00212 #endif
Generated on Tue Jul 12 2022 18:06:46 by
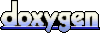