
Fork of my original MQTTGateway
Embed:
(wiki syntax)
Show/hide line numbers
DownloadFile.h
00001 #ifndef _DOWNLOAD_FILE_H_ 00002 #define _DOWNLOAD_FILE_H_ 00003 00004 #include <stdio.h> 00005 #include <string> 00006 #include "mbed.h" 00007 #include "rtos.h" 00008 #include "NetworkInterface.h" 00009 #include "https_request.h" 00010 #include "http_request.h" 00011 00012 class DownloadFile 00013 { 00014 public: 00015 DownloadFile(NetworkInterface* nw, const char * file = NULL, const char * capem = NULL) 00016 :network(nw), 00017 filename(file), 00018 pem(capem), 00019 useSSL(capem != NULL), 00020 fp(NULL), 00021 size_written(0), 00022 get_req_ssl(NULL), 00023 get_req(NULL) 00024 { 00025 if (filename) 00026 { 00027 fp = fopen(file, "w+"); 00028 if (fp != NULL) 00029 printf("File open successfull!\r\n"); 00030 } 00031 } 00032 00033 virtual ~DownloadFile() 00034 { 00035 if (fp != NULL) 00036 fclose(fp); 00037 00038 // HttpsRequest destructor also free's up 00039 // the HttpsResult ... so it must be consumed 00040 // before this class goes out of scope 00041 if(get_req) 00042 delete get_req; 00043 if(get_req_ssl) 00044 delete get_req_ssl; 00045 } 00046 00047 HttpResponse* get_file(const char * url); 00048 00049 std::string get_file_content(); 00050 00051 const char * get_filename() 00052 { 00053 return filename; 00054 } 00055 00056 size_t get_written_size() { 00057 return size_written; 00058 } 00059 00060 void basic_auth(const char * user, const char * password); 00061 00062 00063 protected: 00064 void body_callback(const char* data, size_t data_len); 00065 00066 private: 00067 NetworkInterface* network; 00068 const char * filename; 00069 const char * pem; 00070 bool useSSL; 00071 FILE * fp; 00072 size_t size_written; 00073 std::string authstr; 00074 00075 HttpsRequest* get_req_ssl; 00076 HttpRequest* get_req; 00077 }; 00078 00079 #endif 00080
Generated on Tue Jul 12 2022 18:06:45 by
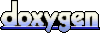