
working code of cdms with i2c
Fork of rtos_basic by
Embed:
(wiki syntax)
Show/hide line numbers
master.cpp
00001 #include "master.h" 00002 00003 I2C master (p28,p27); //configure pins p27,p28 as I2C master 00004 Serial pc (USBTX,USBRX); 00005 00006 struct SensorData //HK_data_structure 00007 { 00008 char voltage[10]; 00009 char current[10]; 00010 char temp[10]; 00011 } Sensor; 00012 00013 void FUNC_I2C_MASTER_MAIN(char command, int slave_address, int iterations) 00014 { 00015 wait(0.5); 00016 printf("1\n"); 00017 bool acknowledge1; 00018 bool acknowledge2; 00019 uint8_t loopvariable2=0; 00020 bool loopvariable1 = true; 00021 bool loopvariable3 = true; 00022 while(loopvariable1) 00023 { 00024 //-------------writing the command to slave-------------------------------------------------------------- 00025 printf("2\n"); 00026 master.frequency(100000); //set clock frequency 00027 master.start(); //initiating the data transfer 00028 acknowledge2 = (bool) master.write(slave_address|0x00); //addressing the slave to write 00029 if(acknowledge2) //proceeding further only if slave is addressed 00030 { 00031 printf("3\n"); 00032 acknowledge1 = (bool) master.write(command); //sending the command to slave 00033 if(acknowledge1) //proceeding further only if sent data is acknowledged 00034 { 00035 printf("acknowledge1=%d\n",acknowledge1); 00036 loopvariable1=false; //if acknowledged, breaking loop in next iteration 00037 00038 //--------------reading data from slave--------------------------------------------------------------- 00039 while(loopvariable3) 00040 { 00041 master.frequency(100000); //set clock frequency 00042 master.start(); //initiate data transfer 00043 acknowledge1 = (bool) master.write(slave_address | 0x01); //addressing the slave to read 00044 00045 if(acknowledge1) //proceedong only if slae is addressed 00046 { 00047 loopvariable3 = false; 00048 while(loopvariable2<8) 00049 { 00050 Sensor.voltage[loopvariable2] = receive_byte(); //receiving data if acknowledged 00051 printf(" voltage%d = %x\n",loopvariable2,Sensor.voltage[loopvariable2]); 00052 00053 Sensor.current[loopvariable2] = receive_byte(); //receiving data if acknowledged 00054 printf(" current%d = %x\n",loopvariable2, Sensor.current[loopvariable2]); 00055 00056 Sensor.temp[loopvariable2] = receive_byte(); //receiving data if acknowledged 00057 printf(" temperature%d = %x\n",loopvariable2,Sensor.temp[loopvariable2]); 00058 00059 loopvariable2++; 00060 00061 } //while(loopvariable2<30) 00062 }//if(acknowledge1==1) 00063 00064 master.stop(); 00065 }//while(loopvariable3) 00066 }//if(acknowledge1==1) 00067 }//if(acknowledge2==1) 00068 }//while(loopvariable1) 00069 printf("done"); 00070 }//main 00071 00072 00073 //----------------function to read and return the data received----------------------------------- 00074 char receive_byte() 00075 { 00076 00077 char value; 00078 value = master.read(1); 00079 return(value); //returning the 4 byte floating point number 00080 } 00081
Generated on Sun Jul 24 2022 21:13:36 by
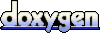