
Change LED brightness brightness from 0 to 100%
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //Change the brightness of onboard LED using PWM 00002 // Tested with FRDM-KL25Z board 00003 #include "mbed.h" 00004 00005 PwmOut led(LED1); 00006 00007 00008 int main() 00009 { 00010 float duty_cycle = 1; //PWM output is inverted for LED. Therefore duty_cycle = 1 means LED off 00011 00012 led.period_ms(1); // PWM freequency = 1/period = 1 kHz 00013 00014 while(1) 00015 { 00016 led = duty_cycle; // also "led.write(duty_cycle)" will do the same thing 00017 duty_cycle = duty_cycle - 0.01; 00018 wait(0.05); // delay for visually see the effect 00019 00020 if(duty_cycle <= 0) 00021 duty_cycle = 1; 00022 00023 } 00024 }
Generated on Thu Jul 14 2022 06:49:11 by
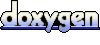