
Modify the BlinkThreadCallback example so you run two threads that blink different LEDs once per second by waiting for each other
main.cpp
00001 #include "mbed.h" 00002 00003 DigitalOut led1(LED1); 00004 DigitalOut led2(LED2); 00005 InterruptIn button(USER_BUTTON); 00006 00007 //____________________________ 00008 00009 Thread thread1; 00010 Thread thread2; 00011 00012 //____________________________ 00013 00014 bool is_debug_enabled = false; 00015 bool is_t2_done = true; 00016 bool is_t1_done = false; 00017 00018 void toggle_debug_mode() { 00019 is_debug_enabled = !is_debug_enabled; 00020 } 00021 00022 void led1_thread(DigitalOut *led) { 00023 if(is_debug_enabled) 00024 printf("T1 STARTED\n"); 00025 while (true) { 00026 if(is_t2_done){ 00027 if(is_debug_enabled) 00028 printf("T1 EXECUTING\n"); 00029 is_t1_done = false; 00030 is_t2_done = false; 00031 *led = !*led; 00032 wait(1); 00033 *led = !*led; 00034 is_t1_done = true; 00035 if(is_debug_enabled) 00036 printf("T1 DONE\n\n"); 00037 } 00038 } 00039 } 00040 00041 void led2_thread(DigitalOut *led) { 00042 if(is_debug_enabled) 00043 printf("T2 STARTED\n"); 00044 while (true) { 00045 if(is_t1_done){ 00046 if(is_debug_enabled) 00047 printf("T2 EXECUTING\n"); 00048 is_t1_done = false; 00049 is_t2_done = false; 00050 *led = !*led; 00051 wait(1); 00052 *led = !*led; 00053 is_t2_done = true; 00054 if(is_debug_enabled) 00055 printf("T2 DONE\n\n"); 00056 } 00057 } 00058 } 00059 00060 int main() { 00061 button.rise(&toggle_debug_mode); 00062 thread1.start(callback(led1_thread, &led1)); 00063 thread2.start(callback(led2_thread, &led2)); 00064 thread1.join(); 00065 thread2.join(); 00066 }
Generated on Wed Aug 31 2022 20:29:07 by
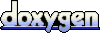