
Modify the previous example to use NVStore for keeping track of how many times every different LED turned on This information must persist across system reboots! Print this information on STDout at every boot before running the application
Dependencies: BSP_B-L475E-IOT01
main.cpp
00001 #include "mbed.h" 00002 00003 #include "stm32l475e_iot01_accelero.h" 00004 00005 #include <stdio.h> 00006 #include <errno.h> 00007 00008 // Block devices 00009 #if COMPONENT_SPIF 00010 #include "SPIFBlockDevice.h" 00011 #endif 00012 00013 #if COMPONENT_DATAFLASH 00014 #include "DataFlashBlockDevice.h" 00015 #endif 00016 00017 #if COMPONENT_SD 00018 #include "SDBlockDevice.h" 00019 #endif 00020 00021 #include "HeapBlockDevice.h" 00022 00023 // File systems 00024 #include "LittleFileSystem.h" 00025 #include "FATFileSystem.h" 00026 #include "nvstore.h" 00027 00028 00029 // Physical block device, can be any device that supports the BlockDevice API 00030 /*SPIFBlockDevice bd( 00031 MBED_CONF_SPIF_DRIVER_SPI_MOSI, 00032 MBED_CONF_SPIF_DRIVER_SPI_MISO, 00033 MBED_CONF_SPIF_DRIVER_SPI_CLK, 00034 MBED_CONF_SPIF_DRIVER_SPI_CS);*/ 00035 00036 #define BLOCK_SIZE 512 00037 HeapBlockDevice bd(16384, BLOCK_SIZE); 00038 00039 // File system declaration 00040 LittleFileSystem fs("fs"); 00041 00042 DigitalOut led1(LED1); 00043 DigitalOut led2(LED2); 00044 DigitalOut led3(LED3); 00045 00046 Ticker timeout_ticker; 00047 Thread t; 00048 00049 static FILE *f; 00050 volatile int seconds_passed = 0; 00051 uint16_t key_1 = 1; 00052 uint32_t value_1; 00053 uint16_t key_2 = 2; 00054 uint32_t value_2; 00055 uint16_t key_3 = 3; 00056 uint32_t value_3; 00057 00058 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00059 00060 InterruptIn button(USER_BUTTON); 00061 int16_t pDataXYZ[3] = {0}; 00062 00063 void toggle_led(int led1_status, int led2_status, int led3_status){ 00064 led1 = led1_status; 00065 led2 = led2_status; 00066 led3 = led3_status; 00067 } 00068 00069 00070 bool is_board_horizontal(int16_t pDataXYZ[3]) { 00071 return (pDataXYZ[2] < 1030 && pDataXYZ[2] > 950) || (pDataXYZ[2] < -950 && pDataXYZ[2] > -1030); 00072 } 00073 00074 bool is_board_vertical_short(int16_t pDataXYZ[3]) { 00075 return (pDataXYZ[0] < -950 && pDataXYZ[0] > -1030) || (pDataXYZ[0] < 1030 && pDataXYZ[0] > 950); 00076 } 00077 00078 bool is_board_vertical_long(int16_t pDataXYZ[3]) { 00079 return (pDataXYZ[1] < 1030 && pDataXYZ[1] > 950) || (pDataXYZ[1] < -950 && pDataXYZ[1] > -1030); 00080 } 00081 00082 void write_positions_on_file() { 00083 int16_t pDataXYZ[3] = {0}; 00084 BSP_ACCELERO_AccGetXYZ(pDataXYZ); 00085 if (is_board_vertical_short(pDataXYZ)) { 00086 fprintf(f, "%d\n", 0); 00087 } else if (is_board_vertical_long(pDataXYZ)) { 00088 fprintf(f, "%d\n", 1); 00089 } else if (is_board_horizontal(pDataXYZ)) { 00090 fprintf(f, "%d\n", 2); 00091 } else { 00092 fprintf(f, "%d\n", -1); 00093 } 00094 fflush(f); 00095 fflush(stdout); 00096 } 00097 00098 void toggle_led_based_on_position() { 00099 int horizontal_occurrencies = 0; 00100 int long_vertical_occurrencies = 0; 00101 int short_vertical_occurrencies = 0; 00102 fflush(stdout); 00103 fflush(f); 00104 00105 fseek(f, 0, SEEK_SET); 00106 int position; 00107 while (!feof(f)) { 00108 fscanf(f, "%d", &position); 00109 if (position == 0) { 00110 short_vertical_occurrencies +=1; 00111 } else if(position == 1) { 00112 long_vertical_occurrencies +=1; 00113 } else if(position == 2) { 00114 horizontal_occurrencies +=1; 00115 } 00116 } 00117 printf("horizontal occ: %d \n",horizontal_occurrencies); 00118 printf("long vert occ: %d \n",long_vertical_occurrencies); 00119 printf("short vert occ: %d \n",short_vertical_occurrencies); 00120 NVStore &nvstore = NVStore::get_instance(); 00121 if (horizontal_occurrencies >= long_vertical_occurrencies && horizontal_occurrencies >= short_vertical_occurrencies) { 00122 toggle_led(1,0,0); 00123 value_1+=1; 00124 nvstore.set(key_1, sizeof(value_1), &value_1); 00125 } else if (long_vertical_occurrencies >= horizontal_occurrencies && long_vertical_occurrencies >= short_vertical_occurrencies ) { 00126 toggle_led(0,1,0); 00127 value_2+=1; 00128 nvstore.set(key_2, sizeof(value_2), &value_2); 00129 } else if (short_vertical_occurrencies >= horizontal_occurrencies && short_vertical_occurrencies >= long_vertical_occurrencies) { 00130 toggle_led(0,0,1); 00131 value_3+=1; 00132 nvstore.set(key_3, sizeof(value_3), &value_3); 00133 } 00134 printf("Press the restart button to sample again.\n"); 00135 00136 fflush(stdout); 00137 int err = fclose(f); 00138 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00139 if (err < 0) { 00140 error("error: %s (%d)\n", strerror(err), -err); 00141 } 00142 err = fs.unmount(); 00143 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00144 if (err < 0) { 00145 error("error: %s (%d)\n", strerror(-err), err); 00146 } 00147 00148 } 00149 00150 void toggle() { 00151 int16_t pDataXYZ[3] = {0}; 00152 BSP_ACCELERO_AccGetXYZ(pDataXYZ); 00153 printf("ACCELERO_X = %d\n", pDataXYZ[0]); 00154 printf("ACCELERO_Y = %d\n", pDataXYZ[1]); 00155 printf("ACCELERO_Z = %d\n\n", pDataXYZ[2]); 00156 } 00157 00158 00159 00160 void ticker_attach() { 00161 queue.call(write_positions_on_file); 00162 seconds_passed++; 00163 // Blink correct led after 10 seconds 00164 if (seconds_passed == 1000) { 00165 timeout_ticker.detach(); 00166 queue.call(toggle_led_based_on_position); 00167 seconds_passed = 0; 00168 } 00169 } 00170 00171 int main(){ 00172 t.start(callback(&queue, &EventQueue::dispatch_forever)); 00173 BSP_ACCELERO_Init(); 00174 00175 NVStore &nvstore = NVStore::get_instance(); 00176 00177 uint16_t actual_len_bytes = 0; 00178 int rc; 00179 00180 rc = nvstore.init(); 00181 printf("Init NVStore. \n"); 00182 00183 rc = nvstore.get(key_1, sizeof(value_1), &value_1, actual_len_bytes); 00184 if (rc == NVSTORE_NOT_FOUND) { 00185 value_1 = 0; 00186 value_2 = 0; 00187 value_3 = 0; 00188 nvstore.set(key_1, sizeof(value_1), &value_1); 00189 nvstore.set(key_2, sizeof(value_2), &value_2); 00190 nvstore.set(key_3, sizeof(value_3), &value_3); 00191 } else { 00192 nvstore.get(key_2, sizeof(value_2), &value_2, actual_len_bytes); 00193 nvstore.get(key_3, sizeof(value_3), &value_3, actual_len_bytes); 00194 } 00195 00196 printf("LED_1: %d\nLED_2: %d\nLED_3: %d\n", value_1, value_2, value_3); 00197 00198 // Try to mount the filesystem 00199 printf("Mounting the filesystem... "); 00200 fflush(stdout); 00201 int err = fs.mount(&bd); 00202 printf("%s\n", (err ? "Fail :(" : "OK")); 00203 if (err) { 00204 // Reformat if we can't mount the filesystem 00205 // this should only happen on the first boot 00206 printf("No filesystem found, formatting... "); 00207 fflush(stdout); 00208 err = fs.reformat(&bd); 00209 printf("%s\n", (err ? "Fail :(" : "OK")); 00210 if (err) { 00211 error("error: %s (%d)\n", strerror(-err), err); 00212 } 00213 } 00214 00215 // Open the numbers file 00216 printf("Opening \"/fs/numbers.txt\"... "); 00217 fflush(stdout); 00218 f = fopen("/fs/numbers.txt", "r+"); 00219 printf("%s\n", (!f ? "Fail :(" : "OK")); 00220 if (!f) { 00221 // Create the numbers file if it doesn't exist 00222 printf("No file found, creating a new file... "); 00223 fflush(stdout); 00224 f = fopen("/fs/numbers.txt", "w+"); 00225 printf("%s\n", (!f ? "Fail :(" : "OK")); 00226 if (!f) { 00227 error("error: %s (%d)\n", strerror(errno), -errno); 00228 } 00229 } 00230 00231 timeout_ticker.attach(&ticker_attach, 0.01); 00232 }
Generated on Sun Jul 24 2022 11:43:35 by
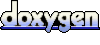