
Samples acceleration at 100 Hz for 10 seconds. At the end of the 10 seconds, turns on: - LED1 if the board stayed horizontal for most time - LED2 if the board stayed on the long edge for most time - LED3 if the board stayed on the short edge for most time
Dependencies: BSP_B-L475E-IOT01
main.cpp
00001 #include "mbed.h" 00002 00003 #include "stm32l475e_iot01_accelero.h" 00004 00005 #include <stdio.h> 00006 #include <errno.h> 00007 00008 // Block devices 00009 #if COMPONENT_SPIF 00010 #include "SPIFBlockDevice.h" 00011 #endif 00012 00013 #if COMPONENT_DATAFLASH 00014 #include "DataFlashBlockDevice.h" 00015 #endif 00016 00017 #if COMPONENT_SD 00018 #include "SDBlockDevice.h" 00019 #endif 00020 00021 #include "HeapBlockDevice.h" 00022 00023 // File systems 00024 #include "LittleFileSystem.h" 00025 #include "FATFileSystem.h" 00026 00027 // Physical block device, can be any device that supports the BlockDevice API 00028 /*SPIFBlockDevice bd( 00029 MBED_CONF_SPIF_DRIVER_SPI_MOSI, 00030 MBED_CONF_SPIF_DRIVER_SPI_MISO, 00031 MBED_CONF_SPIF_DRIVER_SPI_CLK, 00032 MBED_CONF_SPIF_DRIVER_SPI_CS);*/ 00033 00034 #define BLOCK_SIZE 512 00035 HeapBlockDevice bd(16384, BLOCK_SIZE); 00036 00037 // File system declaration 00038 LittleFileSystem fs("fs"); 00039 00040 DigitalOut led1(LED1); 00041 DigitalOut led2(LED2); 00042 DigitalOut led3(LED3); 00043 00044 Ticker timeout_ticker; 00045 Thread t; 00046 00047 static FILE *f; 00048 volatile int seconds_passed = 0; 00049 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00050 00051 InterruptIn button(USER_BUTTON); 00052 int16_t pDataXYZ[3] = {0}; 00053 00054 void toggle_led(int led1_status, int led2_status, int led3_status){ 00055 led1 = led1_status; 00056 led2 = led2_status; 00057 led3 = led3_status; 00058 } 00059 00060 bool is_board_horizontal(int16_t pDataXYZ[3]) { 00061 return (pDataXYZ[2] < 1030 && pDataXYZ[2] > 950) || (pDataXYZ[2] < -950 && pDataXYZ[2] > -1030); 00062 } 00063 00064 bool is_board_vertical_short(int16_t pDataXYZ[3]) { 00065 return (pDataXYZ[0] < -950 && pDataXYZ[0] > -1030) || (pDataXYZ[0] < 1030 && pDataXYZ[0] > 950); 00066 } 00067 00068 bool is_board_vertical_long(int16_t pDataXYZ[3]) { 00069 return (pDataXYZ[1] < 1030 && pDataXYZ[1] > 950) || (pDataXYZ[1] < -950 && pDataXYZ[1] > -1030); 00070 } 00071 00072 void write_positions_on_file() { 00073 int16_t pDataXYZ[3] = {0}; 00074 BSP_ACCELERO_AccGetXYZ(pDataXYZ); 00075 if (is_board_vertical_short(pDataXYZ)) { 00076 fprintf(f, "%d\n", 0); 00077 } else if (is_board_vertical_long(pDataXYZ)) { 00078 fprintf(f, "%d\n", 1); 00079 } else if (is_board_horizontal(pDataXYZ)) { 00080 fprintf(f, "%d\n", 2); 00081 } else { 00082 fprintf(f, "%d\n", -1); 00083 } 00084 fflush(f); 00085 fflush(stdout); 00086 } 00087 00088 void toggle_led_based_on_position() { 00089 int horizontal_occurrencies = 0; 00090 int long_vertical_occurrencies = 0; 00091 int short_vertical_occurrencies = 0; 00092 fflush(stdout); 00093 fflush(f); 00094 00095 fseek(f, 0, SEEK_SET); 00096 int position; 00097 while (!feof(f)) { 00098 fscanf(f, "%d", &position); 00099 if (position == 0) { 00100 short_vertical_occurrencies +=1; 00101 } else if(position == 1) { 00102 long_vertical_occurrencies +=1; 00103 } else if(position == 2) { 00104 horizontal_occurrencies +=1; 00105 } 00106 } 00107 printf("horizontal occ: %d \n",horizontal_occurrencies); 00108 printf("long vert occ: %d \n",long_vertical_occurrencies); 00109 printf("short vert occ: %d \n",short_vertical_occurrencies); 00110 if (horizontal_occurrencies >= long_vertical_occurrencies && horizontal_occurrencies >= short_vertical_occurrencies) { 00111 toggle_led(1,0,0); 00112 } else if (long_vertical_occurrencies >= horizontal_occurrencies && long_vertical_occurrencies >= short_vertical_occurrencies ) { 00113 toggle_led(0,1,0); 00114 } else if (short_vertical_occurrencies >= horizontal_occurrencies && short_vertical_occurrencies >= long_vertical_occurrencies) { 00115 toggle_led(0,0,1); 00116 } 00117 00118 printf("Press the restart button to sample again.\n"); 00119 fflush(stdout); 00120 int err = fclose(f); 00121 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00122 if (err < 0) { 00123 error("error: %s (%d)\n", strerror(err), -err); 00124 } 00125 err = fs.unmount(); 00126 printf("%s\n", (err < 0 ? "Fail :(" : "OK")); 00127 if (err < 0) { 00128 error("error: %s (%d)\n", strerror(-err), err); 00129 } 00130 00131 } 00132 00133 void toggle() { 00134 int16_t pDataXYZ[3] = {0}; 00135 BSP_ACCELERO_AccGetXYZ(pDataXYZ); 00136 printf("ACCELERO_X = %d\n", pDataXYZ[0]); 00137 printf("ACCELERO_Y = %d\n", pDataXYZ[1]); 00138 printf("ACCELERO_Z = %d\n\n", pDataXYZ[2]); 00139 } 00140 00141 void ticker_attach() { 00142 queue.call(write_positions_on_file); 00143 seconds_passed++; 00144 // Blink correct led after 10 seconds 00145 if (seconds_passed == 1000) { 00146 timeout_ticker.detach(); 00147 queue.call(toggle_led_based_on_position); 00148 seconds_passed = 0; 00149 } 00150 } 00151 00152 int main(){ 00153 t.start(callback(&queue, &EventQueue::dispatch_forever)); 00154 BSP_ACCELERO_Init(); 00155 00156 // Try to mount the filesystem 00157 printf("Mounting the filesystem... "); 00158 fflush(stdout); 00159 int err = fs.mount(&bd); 00160 printf("%s\n", (err ? "Fail :(" : "OK")); 00161 if (err) { 00162 // Reformat if we can't mount the filesystem 00163 // this should only happen on the first boot 00164 printf("No filesystem found, formatting... "); 00165 fflush(stdout); 00166 err = fs.reformat(&bd); 00167 printf("%s\n", (err ? "Fail :(" : "OK")); 00168 if (err) { 00169 error("error: %s (%d)\n", strerror(-err), err); 00170 } 00171 } 00172 00173 // Open the numbers file 00174 printf("Opening \"/fs/numbers.txt\"... "); 00175 fflush(stdout); 00176 f = fopen("/fs/numbers.txt", "r+"); 00177 printf("%s\n", (!f ? "Fail :(" : "OK")); 00178 if (!f) { 00179 // Create the numbers file if it doesn't exist 00180 printf("No file found, creating a new file... "); 00181 fflush(stdout); 00182 f = fopen("/fs/numbers.txt", "w+"); 00183 printf("%s\n", (!f ? "Fail :(" : "OK")); 00184 if (!f) { 00185 error("error: %s (%d)\n", strerror(errno), -errno); 00186 } 00187 } 00188 00189 timeout_ticker.attach(&ticker_attach, 0.01); 00190 }
Generated on Fri Jul 15 2022 21:49:37 by
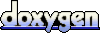