
Test of the accelerometer, digital I/O, on-board LCD screen. Looking at vector product of the x-y components of the accelerometer. Works pretty well. Still rough, program wise - sc 140710
Dependencies: MMA8451Q SLCD mbed
Fork of ACC_LCD_341_MID by
acc_341_VB.cpp
00001 #include "mbed.h" 00002 #include "MMA8451Q.h" 00003 #include "SLCD.h" 00004 00005 /* 00006 Test of the accelerometer, digital I/O, on-board LCD screen. 00007 Looing at vector product of the x-y components of the accelerometer. 00008 Works pretty well. Still rough, program wise - sc 140710 00009 00010 Author: Vishal Bharam 00011 Date: 9/29/2014 00012 */ 00013 00014 #define DATATIME 0.150 00015 #define PROGNAME "ACCLCD341VB\r/n" 00016 #define PRINTDBUG 00017 00018 00019 #if defined (TARGET_KL25Z) || defined (TARGET_KL46Z) 00020 PinName const SDA = PTE25; // Data pins for the accelerometer/magnetometer. 00021 PinName const SCL = PTE24; // DO NOT CHANGE 00022 #elif defined (TARGET_KL05Z) 00023 PinName const SDA = PTB4; 00024 PinName const SCL = PTB3; 00025 #else 00026 #error TARGET NOT DEFINED 00027 #endif 00028 00029 #define MMA8451_I2C_ADDRESS (0x1d<<1) 00030 00031 SLCD slcd; //define LCD display 00032 00033 MMA8451Q acc(SDA, SCL, MMA8451_I2C_ADDRESS); 00034 Serial pc(USBTX, USBRX); 00035 00036 float sqrt_newt(float argument) { 00037 return (sqrt(argument)); 00038 } 00039 00040 00041 void LCDMess(char *lMess, float dWait){ 00042 slcd.Home(); 00043 slcd.clear(); 00044 slcd.printf(lMess); 00045 wait(dWait); 00046 } 00047 00048 00049 int main() { 00050 float xAcc; 00051 float yAcc; 00052 float vector; 00053 char lcdData[10]; //buffer needs places dor decimal pt and colon 00054 00055 #ifdef PRINTDBUG 00056 pc.printf(PROGNAME); 00057 #endif 00058 // main loop forever 00059 while(true) { 00060 00061 //Get accelerometer data - tilt angles minus offset for zero mark. 00062 xAcc = abs(acc.getAccX()); 00063 yAcc = abs(acc.getAccY()); 00064 // Calulate vector sum of x and y reading. 00065 vector = sqrt_newt(pow(xAcc,2) + pow(yAcc,2)); 00066 00067 00068 #ifdef PRINTDBUG 00069 pc.printf("xAcc = %f\r\n", xAcc); 00070 pc.printf("yAcc = %f\r\n", yAcc); 00071 pc.printf("vector = %f\r\n", vector); 00072 #endif 00073 00074 sprintf (lcdData,"%4.3f",vector); 00075 LCDMess(lcdData, DATATIME); 00076 // Wait then do the whole thing again. 00077 wait(DATATIME); 00078 } 00079 }
Generated on Mon Jul 25 2022 00:55:24 by
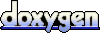