
changed name
Fork of mbed-os-example-ble-BatteryLevel by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2014 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include <events/mbed_events.h> 00018 #include <mbed.h> 00019 #include "ble/BLE.h" 00020 #include "ble/Gap.h" 00021 #include "ble/services/BatteryService.h" 00022 #include "ble/services/HeartRateService.h" 00023 00024 DigitalOut led1(LED1, 1); 00025 00026 const static char DEVICE_NAME[] = "ProVaida"; 00027 static const uint16_t uuid16_list[] = {GattService::UUID_HEART_RATE_SERVICE}; 00028 //static const uint16_t uuid16_list[] = {GattService::UUID_BATTERY_SERVICE}; 00029 00030 uint8_t hrmCounter = 100; 00031 static HeartRateService* hrService; 00032 00033 static EventQueue eventQueue(/* event count */ 16 * EVENTS_EVENT_SIZE); 00034 00035 void disconnectionCallback(const Gap::DisconnectionCallbackParams_t *params) 00036 { 00037 BLE::Instance().gap().startAdvertising(); 00038 } 00039 00040 void updateSensorValue() { 00041 hrmCounter++; 00042 if (hrmCounter > 175) { 00043 hrmCounter = 100; 00044 } 00045 hrService->updateHeartRate(hrmCounter); 00046 //batteryServicePtr->updateBatteryLevel(batteryLevel); 00047 } 00048 00049 void blinkCallback(void) 00050 { 00051 led1 = !led1; /* Do blinky on LED1 while we're waiting for BLE events */ 00052 00053 BLE &ble = BLE::Instance(); 00054 if (ble.gap().getState().connected) { 00055 eventQueue.call(updateSensorValue); 00056 } 00057 } 00058 00059 /** 00060 * This function is called when the ble initialization process has failed 00061 */ 00062 void onBleInitError(BLE &ble, ble_error_t error) 00063 { 00064 /* Initialization error handling should go here */ 00065 } 00066 00067 void printMacAddress() 00068 { 00069 /* Print out device MAC address to the console*/ 00070 Gap::AddressType_t addr_type; 00071 Gap::Address_t address; 00072 BLE::Instance().gap().getAddress(&addr_type, address); 00073 printf("DEVICE MAC ADDRESS: "); 00074 for (int i = 5; i >= 1; i--){ 00075 printf("%02x:", address[i]); 00076 } 00077 printf("%02x\r\n", address[0]); 00078 } 00079 00080 /** 00081 * Callback triggered when the ble initialization process has finished 00082 */ 00083 void bleInitComplete(BLE::InitializationCompleteCallbackContext *params) 00084 { 00085 BLE& ble = params->ble; 00086 ble_error_t error = params->error; 00087 00088 if (error != BLE_ERROR_NONE) { 00089 /* In case of error, forward the error handling to onBleInitError */ 00090 onBleInitError(ble, error); 00091 return; 00092 } 00093 00094 /* Ensure that it is the default instance of BLE */ 00095 if(ble.getInstanceID() != BLE::DEFAULT_INSTANCE) { 00096 return; 00097 } 00098 00099 ble.gap().onDisconnection(disconnectionCallback); 00100 00101 /* Setup primary service */ 00102 hrService = new HeartRateService(ble, hrmCounter, HeartRateService::LOCATION_FINGER); 00103 00104 /* Setup advertising */ 00105 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::BREDR_NOT_SUPPORTED | GapAdvertisingData::LE_GENERAL_DISCOVERABLE); 00106 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LIST_16BIT_SERVICE_IDS, (uint8_t *) uuid16_list, sizeof(uuid16_list)); 00107 ble.gap().accumulateAdvertisingPayload(GapAdvertisingData::COMPLETE_LOCAL_NAME, (uint8_t *) DEVICE_NAME, sizeof(DEVICE_NAME)); 00108 ble.gap().setAdvertisingType(GapAdvertisingParams::ADV_CONNECTABLE_UNDIRECTED); 00109 ble.gap().setAdvertisingInterval(1000); /* 1000ms */ 00110 ble.gap().startAdvertising(); 00111 00112 printMacAddress(); 00113 } 00114 00115 void scheduleBleEventsProcessing(BLE::OnEventsToProcessCallbackContext* context) { 00116 BLE &ble = BLE::Instance(); 00117 eventQueue.call(Callback<void()>(&ble, &BLE::processEvents)); 00118 } 00119 00120 int main() 00121 { 00122 eventQueue.call_every(500, blinkCallback); 00123 00124 BLE &ble = BLE::Instance(); 00125 ble.onEventsToProcess(scheduleBleEventsProcessing); 00126 ble.init(bleInitComplete); 00127 00128 eventQueue.dispatch_forever(); 00129 00130 return 0; 00131 }
Generated on Sun Jul 17 2022 21:48:39 by
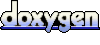