
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
plat_interface.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2010 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __PLAT_H__ 00010 #define __PLAT_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief PyMite's Porting Interface 00016 */ 00017 00018 00019 /** 00020 * Initializes the platform as needed by the routines 00021 * in the platform implementation file. 00022 */ 00023 PmReturn_t plat_init(void); 00024 00025 /** De-initializes the platform after the VM is done running. */ 00026 PmReturn_t plat_deinit(void); 00027 00028 /** 00029 * Returns the byte at the given address in memspace. 00030 * 00031 * Increments the address (just like getc and read(1)) 00032 * to make image loading work (recursively). 00033 * 00034 * PORT: fill in getByte for each memspace in the system; 00035 * call sys_error for invalid memspaces. 00036 * 00037 * @param memspace memory space/type 00038 * @param paddr ptr to address 00039 * @return byte from memory. 00040 * paddr - points to the next byte 00041 */ 00042 uint8_t plat_memGetByte(PmMemSpace_t memspace, uint8_t const **paddr); 00043 00044 /** 00045 * Receives one byte from the default connection, 00046 * usually UART0 on a target device or stdio on the desktop 00047 */ 00048 PmReturn_t plat_getByte(uint8_t *b); 00049 00050 00051 /** 00052 * Sends one byte out on the default connection, 00053 * usually UART0 on a target device or stdio on the desktop 00054 */ 00055 PmReturn_t plat_putByte(uint8_t b); 00056 00057 00058 /** 00059 * Gets the number of timer ticks that have passed since system start. 00060 */ 00061 PmReturn_t plat_getMsTicks(uint32_t *r_ticks); 00062 00063 00064 /** 00065 * Reports an exception or other error that caused the thread to quit 00066 */ 00067 void plat_reportError(PmReturn_t result); 00068 00069 #endif /* __PLAT_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
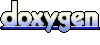