
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
heap.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __HEAP_H__ 00010 #define __HEAP_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief VM Heap 00016 * 00017 * VM heap header. 00018 */ 00019 00020 00021 /** 00022 * The threshold of heap.avail under which the interpreter will run the GC 00023 * just before starting a native session. 00024 */ 00025 #define HEAP_GC_NF_THRESHOLD (512) 00026 00027 00028 #ifdef __DEBUG__ 00029 #define DEBUG_PRINT_HEAP_AVAIL(s) \ 00030 do { uint16_t n; heap_getAvail(&n); printf(s "heap avail = %d\n", n); } \ 00031 while (0) 00032 #else 00033 #define DEBUG_PRINT_HEAP_AVAIL(s) 00034 #endif 00035 00036 00037 /** 00038 * Initializes the heap for use. 00039 * 00040 * @param base The address where the contiguous heap begins 00041 * @param size The size in bytes (octets) of the given heap. 00042 * @return Return code. 00043 */ 00044 PmReturn_t heap_init(uint8_t *base, uint32_t size); 00045 00046 /** 00047 * Returns a free chunk from the heap. 00048 * 00049 * The chunk will be at least the requested size. 00050 * The actual size can be found in the return chunk's od.od_size. 00051 * 00052 * @param requestedsize Requested size of the chunk in bytes. 00053 * @param r_pchunk Addr of ptr to chunk (return). 00054 * @return Return code 00055 */ 00056 PmReturn_t heap_getChunk(uint16_t requestedsize, uint8_t **r_pchunk); 00057 00058 /** 00059 * Places the chunk back in the heap. 00060 * 00061 * @param ptr Pointer to object to free. 00062 */ 00063 PmReturn_t heap_freeChunk(pPmObj_t ptr); 00064 00065 /** @return Return number of bytes available in the heap */ 00066 uint32_t heap_getAvail (void); 00067 00068 /** @return Return the size of the heap in bytes */ 00069 uint32_t heap_getSize (void); 00070 00071 #ifdef HAVE_GC 00072 /** 00073 * Runs the mark-sweep garbage collector 00074 * 00075 * @return Return code 00076 */ 00077 PmReturn_t heap_gcRun(void); 00078 00079 /** 00080 * Enables (if true) or disables automatic garbage collection 00081 * 00082 * @param bool Value to enable or disable auto GC 00083 * @return Return code 00084 */ 00085 PmReturn_t heap_gcSetAuto(uint8_t auto_gc); 00086 00087 #endif /* HAVE_GC */ 00088 00089 /** 00090 * Pushes an object onto the temporary roots stack if there is room 00091 * to protect the objects from a potential garbage collection 00092 * 00093 * @param pobj Object to push onto the roots stack 00094 * @param r_objid By reference; ID to use when popping the object from the stack 00095 */ 00096 void heap_gcPushTempRoot(pPmObj_t pobj, uint8_t *r_objid); 00097 00098 /** 00099 * Pops from the temporary roots stack all objects upto and including the one 00100 * denoted by the given ID 00101 * 00102 * @param objid ID of object to pop 00103 */ 00104 void heap_gcPopTempRoot(uint8_t objid); 00105 00106 #endif /* __HEAP_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
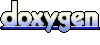