
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
func.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __FUNC_H__ 00010 #define __FUNC_H__ 00011 00012 /** 00013 * \file 00014 * \brief Function Object Type 00015 * 00016 * Function object type header. 00017 */ 00018 00019 /** 00020 * Function obj 00021 * 00022 * A function is like an instance of a code obj. 00023 * Contains ptr to its code obj and has its own attributes dict. 00024 * 00025 * The first (__main__) module that is executed has a function obj 00026 * created for it to execute the bytecode which builds the module. 00027 */ 00028 typedef struct PmFunc_s 00029 { 00030 /** Object descriptor */ 00031 PmObjDesc_t od; 00032 00033 /** Ptr to code obj */ 00034 pPmCo_t f_co; 00035 00036 /** Ptr to attribute dict */ 00037 pPmDict_t f_attrs; 00038 00039 /** Ptr to globals dict */ 00040 pPmDict_t f_globals; 00041 00042 #ifdef HAVE_DEFAULTARGS 00043 /** Ptr to tuple holding default args */ 00044 pPmTuple_t f_defaultargs; 00045 #endif /* HAVE_DEFAULTARGS */ 00046 00047 #ifdef HAVE_CLOSURES 00048 /** Ptr to tuple of cell values */ 00049 pPmTuple_t f_closure; 00050 #endif /* HAVE_CLOSURES */ 00051 00052 } PmFunc_t, 00053 *pPmFunc_t; 00054 00055 00056 /** 00057 * Creates a Function Obj for the given Code Obj. 00058 * Allocate space for a Func obj and fill the fields. 00059 * 00060 * @param pco ptr to code obj 00061 * @param pglobals ptr to globals dict (from containing func/module) 00062 * @param r_pfunc Return by reference; pointer to new function 00063 * @return Return status 00064 */ 00065 PmReturn_t func_new(pPmObj_t pco, pPmObj_t pglobals, pPmObj_t *r_pfunc); 00066 00067 #endif /* __FUNC_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
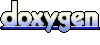