
python-on-a-chip online compiler
Embed:
(wiki syntax)
Show/hide line numbers
codeobj.h
Go to the documentation of this file.
00001 /* 00002 # This file is Copyright 2002 Dean Hall. 00003 # This file is part of the PyMite VM. 00004 # This file is licensed under the MIT License. 00005 # See the LICENSE file for details. 00006 */ 00007 00008 00009 #ifndef __CODEOBJ_H__ 00010 #define __CODEOBJ_H__ 00011 00012 00013 /** 00014 * \file 00015 * \brief CodeObj Type 00016 * 00017 * CodeObj type header. 00018 */ 00019 00020 00021 /** Code image field offset consts */ 00022 #define CI_TYPE_FIELD 0 00023 #define CI_SIZE_FIELD 1 00024 #define CI_ARGCOUNT_FIELD 3 00025 #define CI_FLAGS_FIELD 4 00026 #define CI_STACKSIZE_FIELD 5 00027 #define CI_NLOCALS_FIELD 6 00028 00029 #ifdef HAVE_CLOSURES 00030 # define CI_FREEVARS_FIELD 7 00031 # ifdef HAVE_DEBUG_INFO 00032 # define CI_FIRST_LINE_NO 8 00033 # define CI_NAMES_FIELD 10 00034 # else 00035 # define CI_NAMES_FIELD 8 00036 # endif /* HAVE_DEBUG_INFO */ 00037 #else 00038 # ifdef HAVE_DEBUG_INFO 00039 # define CI_FIRST_LINE_NO 7 00040 # define CI_NAMES_FIELD 9 00041 # else 00042 # define CI_NAMES_FIELD 7 00043 # endif /* HAVE_DEBUG_INFO */ 00044 #endif /* HAVE_CLOSURES */ 00045 00046 00047 /** Native code image size */ 00048 #define NATIVE_IMAGE_SIZE 4 00049 00050 /* Masks for co_flags (from Python's code.h) */ 00051 #define CO_OPTIMIZED 0x01 00052 #define CO_NEWLOCALS 0x02 00053 #define CO_VARARGS 0x04 00054 #define CO_VARKEYWORDS 0x08 00055 #define CO_NESTED 0x10 00056 #define CO_GENERATOR 0x20 00057 #define CO_NOFREE 0x40 00058 00059 /** 00060 * Code Object 00061 * 00062 * An extended object that holds only the most frequently used parts 00063 * of the static code image. Other parts can be obtained by 00064 * inspecting the code image itself. 00065 */ 00066 typedef struct PmCo_s 00067 { 00068 /** Object descriptor */ 00069 PmObjDesc_t od; 00070 /** Address in progmem of the code image, or of code img obj in heap */ 00071 uint8_t const *co_codeimgaddr; 00072 /** Address in RAM of names tuple */ 00073 pPmTuple_t co_names; 00074 /** Address in RAM of constants tuple */ 00075 pPmTuple_t co_consts; 00076 /** Address in memspace of bytecode (or native function) */ 00077 uint8_t const *co_codeaddr; 00078 00079 #ifdef HAVE_DEBUG_INFO 00080 /** Address in memspace of the line number table */ 00081 uint8_t const *co_lnotab; 00082 /** Address in memspace of the filename */ 00083 uint8_t const *co_filename; 00084 /** Line number of first source line of lnotab */ 00085 uint16_t co_firstlineno; 00086 #endif /* HAVE_DEBUG_INFO */ 00087 00088 #ifdef HAVE_CLOSURES 00089 /** Address in RAM of cellvars tuple */ 00090 pPmTuple_t co_cellvars; 00091 /** Number of freevars */ 00092 uint8_t co_nfreevars; 00093 #endif /* HAVE_CLOSURES */ 00094 00095 /** Memory space selector */ 00096 PmMemSpace_t co_memspace:8; 00097 /** Number of positional arguments the function expects */ 00098 uint8_t co_argcount; 00099 /** Compiler flags */ 00100 uint8_t co_flags; 00101 /** Stack size */ 00102 uint8_t co_stacksize; 00103 /** Number of local variables */ 00104 uint8_t co_nlocals; 00105 } PmCo_t, 00106 *pPmCo_t; 00107 00108 /** 00109 * Native Code Object 00110 * 00111 * An extended object that holds only the most frequently used parts 00112 * of the static native image. Other parts can be obtained by 00113 * inspecting the native image itself. 00114 */ 00115 typedef struct PmNo_s 00116 { 00117 /** object descriptor */ 00118 PmObjDesc_t od; 00119 /** expected num args to the func */ 00120 int8_t no_argcount; 00121 /** index into native function table */ 00122 int16_t no_funcindx; 00123 } PmNo_t, 00124 *pPmNo_t; 00125 00126 00127 /** 00128 * Creates a CodeObj by loading info from a code image in memory. 00129 * 00130 * An image is a static representation of a Python object. 00131 * The process of converting an object to and from an image 00132 * is also called marshalling. 00133 * In PyMite, code images are the equivalent of .pyc files. 00134 * Code images can only contain a select subset of object types 00135 * (None, Int, Float, String, Slice?, Tuple, and CodeImg). 00136 * All other types (Lists, Dicts, CodeObjs, Modules, Classes, 00137 * Functions, ClassInstances) are built at runtime. 00138 * 00139 * All multibyte values are in Little Endian order 00140 * (least significant byte comes first in the byte stream). 00141 * 00142 * memspace and *paddr determine the start of the code image. 00143 * Load the code object with values from the code image, 00144 * including the names and consts tuples. 00145 * Leave contents of paddr pointing one byte past end of 00146 * code img. 00147 * 00148 * The code image has the following structure: 00149 * -type: 8b - OBJ_TYPE_CIM 00150 * -size: 16b - number of bytes 00151 * the code image occupies. 00152 * -argcount: 8b - number of arguments to this code obj. 00153 * -stacksz: 8b - the maximum arg-stack size needed. 00154 * -nlocals: 8b - number of local vars in the code obj. 00155 * -names: Tuple - tuple of string objs. 00156 * -consts: Tuple - tuple of objs. 00157 * -code: 8b[] - bytecode array. 00158 * 00159 * @param memspace memory space containing image 00160 * @param paddr ptr to ptr to code img in memspace 00161 * return by reference: paddr points one byte 00162 * past end of code img 00163 * @param r_pco Return arg. New code object with fields 00164 * filled in. 00165 * @return Return status 00166 */ 00167 PmReturn_t 00168 co_loadFromImg(PmMemSpace_t memspace, uint8_t const **paddr, pPmObj_t *r_pco); 00169 00170 /** 00171 * Recursively sets image address of the CO and all its nested COs 00172 * in its constant pool. This is done so that an image that was 00173 * received during an interactive session will persist as long as any 00174 * of its COs/funcs/objects is still alive. 00175 * 00176 * @param pco Pointer to root code object whose images are set 00177 * @param pimg Pointer to very top of code image (PmodeImgObj) 00178 */ 00179 void co_rSetCodeImgAddr(pPmCo_t pco, uint8_t const *pimg); 00180 00181 /** 00182 * Creates a Native code object by loading a native image. 00183 * 00184 * An image is a static representation of a Python object. 00185 * A native image is much smaller than a regular image 00186 * because only two items of data are needed after the type: 00187 * the number of args the func expects and the index into 00188 * the native function table. 00189 * A reference to the image is not needed since it is 00190 * just as efficient to store the info in RAM as it is to 00191 * store a pointer and memspace value. 00192 * 00193 * memspace and *paddr determine the start of the native image. 00194 * Loads the argcount and the func index from the native object. 00195 * Leaves contents of paddr pointing one byte past end of 00196 * code img. 00197 * 00198 * The native image has the following structure: 00199 * -type: 8b - OBJ_TYPE_CIM 00200 * -argcount: 8b - number of arguments to this code obj. 00201 * -code: 16b - index into native function table. 00202 * 00203 * @param memspace memory space containing image 00204 * @param paddr ptr to ptr to code img in memspace (return) 00205 * @param r_pno Return by reference, new code object 00206 * @return Return status 00207 */ 00208 PmReturn_t no_loadFromImg(PmMemSpace_t memspace, 00209 uint8_t const **paddr, pPmObj_t *r_pno); 00210 00211 #endif /* __CODEOBJ_H__ */
Generated on Tue Jul 12 2022 23:13:47 by
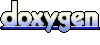