
LPC800-MAX RGB demo using SCT and MRT
Embed:
(wiki syntax)
Show/hide line numbers
MRT.cpp
00001 #include "MRT.h" 00002 00003 MRT::MRT(int channel) 00004 { 00005 static bool insted = false; 00006 00007 if (!insted) { 00008 inst(); 00009 insted = true; 00010 } 00011 00012 _ch = &LPC_MRT->Channel[channel]; 00013 _ch->CTRL |= (1<<1); // one-shot 00014 } 00015 00016 void MRT::inst() 00017 { 00018 LPC_SYSCON->SYSAHBCLKCTRL |= (1<<10); // enable MRT 00019 LPC_SYSCON->PRESETCTRL |= (1<<7); // reset MRT 00020 us_clk = SystemCoreClock / 1000000; 00021 } 00022 00023 void MRT::write(uint32_t interval) 00024 { 00025 _ch->INTVAL = interval | (1<<31); // and LOAD 00026 _ch->STAT |= 0x01; 00027 } 00028 00029 int MRT::status() 00030 { 00031 return (_ch->STAT & 1) ? IDLE : RUNNING; 00032 } 00033 00034 void MRT::wait_ms(uint32_t timeout_ms) 00035 { 00036 wait_raw(us_clk * 1000 * timeout_ms); 00037 } 00038 00039 void MRT::wait_us(uint32_t timeout_us) 00040 { 00041 wait_raw(us_clk * timeout_us); 00042 } 00043 00044 void MRT::wait_raw(uint32_t timeout) 00045 { 00046 write(timeout); 00047 while(status() == RUNNING); 00048 } 00049 00050 uint32_t MRT::read() 00051 { 00052 return _ch->TIMER; 00053 }
Generated on Fri Jul 15 2022 08:12:42 by
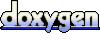