Emulation of LocalFileSystem with virtual COM.
Dependents: KL46Z-lpc81isp lpcterm2
mystring.h
00001 #include <stdlib.h> 00002 #include <string.h> 00003 #pragma once 00004 class mystring { 00005 public: 00006 mystring(){ 00007 _init(); 00008 } 00009 mystring(const char* s) { 00010 _init(); 00011 append(s); 00012 } 00013 00014 ~mystring() { 00015 if (_buf) { 00016 free(_buf); 00017 } 00018 } 00019 void clear() { 00020 if (_buf) { 00021 free(_buf); 00022 } 00023 _init(); 00024 } 00025 bool empty() { 00026 return _len == 0; 00027 } 00028 size_t size() { 00029 return _len; 00030 } 00031 void append(const char* s, int len) { 00032 if (_buf == NULL) { 00033 return; 00034 } 00035 int new_len = _len + len; 00036 char* new_buf = (char*)malloc(new_len + 1); 00037 if (new_buf == NULL) { 00038 return; 00039 } 00040 memcpy(new_buf, _buf, _len); 00041 memcpy(new_buf+_len, s, len); 00042 new_buf[_len+len] = '\0'; 00043 free(_buf); 00044 _buf = new_buf; 00045 _len += new_len; 00046 } 00047 void append(const char* s) { 00048 append(s, strlen(s)); 00049 } 00050 void append(int c) { 00051 char buf[1]; 00052 buf[0] = c; 00053 append(buf, sizeof(buf)); 00054 } 00055 char* c_str() { 00056 if (_buf) { 00057 return _buf; 00058 } 00059 return ""; 00060 } 00061 mystring& operator= (const char* s) { 00062 _init(); 00063 append(s); 00064 return *this; 00065 } 00066 mystring& operator+= (const char* s) { 00067 append(s); 00068 return *this; 00069 } 00070 char& operator[] (size_t pos) { 00071 return _buf[pos]; 00072 } 00073 private: 00074 void _init() { 00075 _len = 0; 00076 _buf = (char*)malloc(1); 00077 if (_buf) { 00078 _buf[0] = '\0'; 00079 } 00080 } 00081 char* _buf; 00082 int _len; 00083 };
Generated on Tue Jul 12 2022 19:39:32 by
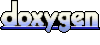