Emulation of LocalFileSystem with virtual COM.
Dependents: KL46Z-lpc81isp lpcterm2
Storage.cpp
00001 #include "Storage.h" 00002 #include "FATFileSystem.h" 00003 #include <ctype.h> 00004 00005 #if (DEBUG2 > 3) 00006 #define STORAGE_DBG(...) do{fprintf(stderr,"[%s@%d] ",__PRETTY_FUNCTION__,__LINE__);fprintf(stderr,__VA_ARGS__);fprintf(stderr,"\r\n");} while(0); 00007 #else 00008 #define STORAGE_DBG(...) 00009 #endif 00010 00011 LocalStorage::LocalStorage(StorageInterface* storage, const char* name) 00012 : FATFileSystem(name),_storage(storage) 00013 { 00014 _name = name; 00015 } 00016 00017 static bool match1(const char* name, const char* pat) { 00018 while(1) { 00019 char c = *pat++; 00020 char d = *name++; 00021 if (c == '\0' && d == '\0') { 00022 return true; 00023 } else if (c == '\0' || d == '\0') { 00024 return false; 00025 } 00026 switch(c) { 00027 case '?': 00028 break; 00029 case '*': 00030 name--; 00031 while(*name) { 00032 if (*name == '.') { 00033 break; 00034 } 00035 name++; 00036 } 00037 break; 00038 default: 00039 if (toupper(d) != toupper(c)) { 00040 return false; 00041 } 00042 break; 00043 } 00044 } 00045 } 00046 00047 extern FILINFO FATDirHandle_finfo; // fat/FATDirHandle.cpp 00048 /* static */ bool LocalStorage::find(char* name, size_t size, const char* dirname, const char* pat) 00049 { 00050 char dirpath[32]; 00051 strcpy(dirpath, "/"); 00052 if (strlen(dirname) >= sizeof(dirpath)-2) { 00053 return false; 00054 } 00055 strcat(dirpath, dirname); 00056 DIR *dir = ::opendir(dirpath); 00057 if (dir == NULL) { 00058 return false; 00059 } 00060 uint32_t fdatetime = 0; 00061 bool found = false; 00062 struct dirent *entry; 00063 while ((entry = readdir(dir)) != NULL) { 00064 if (match1(entry->d_name, pat)) { 00065 FILINFO* fi = &FATDirHandle_finfo; 00066 uint32_t datetime = fi->ftime | (fi->fdate<<16); 00067 STORAGE_DBG("datetime=%08x [%s]", datetime, entry->d_name); 00068 if (datetime > fdatetime) { 00069 fdatetime = datetime; 00070 if (strlen(dirpath) + 1 + strlen(entry->d_name) < size) { 00071 strcpy(name, dirpath); 00072 strcat(name, "/"); 00073 strcat(name, entry->d_name); 00074 found = true; 00075 } 00076 } 00077 } 00078 } 00079 closedir(dir); 00080 return found; 00081 } 00082
Generated on Tue Jul 12 2022 19:39:32 by
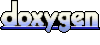