Emulation of LocalFileSystem with virtual COM.
Dependents: KL46Z-lpc81isp lpcterm2
SWSPI.h
00001 /* SWSPI, Software SPI library 00002 * Copyright (c) 2012-2014, David R. Van Wagner, http://techwithdave.blogspot.com 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #ifndef SWSPI_H 00024 #define SWSPI_H 00025 00026 /** A software implemented SPI that can use any digital pins 00027 * 00028 * Useful when don't want to share a single SPI hardware among attached devices 00029 * or when pinout doesn't match exactly to the target's SPI pins 00030 * 00031 * @code 00032 * #include "mbed.h" 00033 * #include "SWSPI.h" 00034 * 00035 * SWSPI spi(p5, p6, p7); // mosi, miso, sclk 00036 * 00037 * int main() 00038 * { 00039 * DigitalOut cs(p8); 00040 * spi.format(8, 0); 00041 * spi.frequency(10000000); 00042 * cs.write(0); 00043 * spi.write(0x9f); 00044 * int jedecid = (spi.write(0) << 16) | (spi.write(0) << 8) | spi.write(0); 00045 * cs.write(1); 00046 * } 00047 * @endcode 00048 */ 00049 class SWSPI 00050 { 00051 private: 00052 uint8_t fast_write(uint8_t value); 00053 DigitalOut* mosi; 00054 DigitalIn* miso; 00055 DigitalOut* sclk; 00056 int port; 00057 int bits; 00058 int mode; 00059 int polarity; // idle clock value 00060 int phase; // 0=sample on leading (first) clock edge, 1=trailing (second) 00061 int freq; 00062 bool _fast; 00063 00064 public: 00065 /** Create SWSPI object 00066 * 00067 * @param mosi_pin 00068 * @param miso_pin 00069 * @param sclk_pin 00070 */ 00071 SWSPI(PinName mosi_pin, PinName miso_pin, PinName sclk_pin); 00072 00073 /** Destructor */ 00074 ~SWSPI(); 00075 00076 /** Specify SPI format 00077 * 00078 * @param bits 8 or 16 are typical values 00079 * @param mode 0, 1, 2, or 3 phase (bit1) and idle clock (bit0) 00080 */ 00081 void format(int bits, int mode = 0); 00082 00083 /** Specify SPI clock frequency 00084 * 00085 * @param hz frequency (optional, defaults to 10000000) 00086 */ 00087 void frequency(int hz = 10000000); 00088 00089 /** Write data and read result 00090 * 00091 * @param value data to write (see format for bit size) 00092 * returns value read from device 00093 */ 00094 int write(int value); 00095 }; 00096 00097 #endif // SWSPI_H
Generated on Tue Jul 12 2022 19:39:32 by
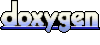