Simple USBHost library for LPC4088. Backward compatibility of official-USBHost.
Dependencies: FATFileSystem mbed-rtos
USBEndpoint.cpp
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #include "USBHost.h" 00018 #include "BaseUsbHostDebug.h" 00019 #include "USBEndpoint.h" 00020 #include "BaseUsbHostTest.h" 00021 00022 HCTD* USBEndpoint::get_queue_HCTD(uint32_t millisec) 00023 { 00024 for(int i = 0; i < 16; i++) { 00025 osEvent evt = m_queue.get(millisec); 00026 if (evt.status == osEventMessage) { 00027 HCTD* td = reinterpret_cast<HCTD*>(evt.value.p); 00028 TEST_ASSERT(td); 00029 uint8_t cc = td->ConditionCode(); 00030 if (cc != 0) { 00031 m_ConditionCode = cc; 00032 DBG_TD(td); 00033 } 00034 return td; 00035 } else if (evt.status == osOK) { 00036 continue; 00037 } else if (evt.status == osEventTimeout) { 00038 return NULL; 00039 } else { 00040 DBG("evt.status: %02x\n", evt.status); 00041 TEST_ASSERT(evt.status == osEventMessage); 00042 } 00043 } 00044 return NULL; 00045 } 00046 00047
Generated on Tue Jul 12 2022 22:44:17 by
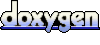