Simple USBHost library for LPC4088. Backward compatibility of official-USBHost.
Dependencies: FATFileSystem mbed-rtos
BaseUsbHostLib.cpp
00001 // BaseUsbHostLib.cpp 2014/4/22 00002 #include "USBHost.h" 00003 //#define DEBUG 00004 #include "BaseUsbHostDebug.h" 00005 #define TEST 00006 #include "BaseUsbHostTest.h" 00007 00008 //#define DBG_USE_POSIX_MEMALIGN 00009 00010 #ifdef DBG_USE_POSIX_MEMALIGN 00011 void* usb_ram_malloc(size_t size, int aligment) 00012 { 00013 TEST_ASSERT(aligment >= 4); 00014 TEST_ASSERT(!(aligment & 3)); 00015 void* p; 00016 if (posix_memalign(&p, aligment, size) == 0) { 00017 return p; 00018 } 00019 return NULL; 00020 } 00021 00022 void usb_ram_free(void* p) 00023 { 00024 free(p); 00025 } 00026 #else 00027 00028 #define CHUNK_SIZE 64 00029 00030 #if defined(TARGET_LPC1768) 00031 #define USB_RAM_BASE 0x2007C000 00032 #define USB_RAM_SIZE 0x4000 00033 #define BLOCK_COUNT (USB_RAM_SIZE/CHUNK_SIZE) 00034 #elif defined(TARGET_LPC4088) 00035 #define USB_RAM_BASE 0x20000000 00036 #define USB_RAM_SIZE 0x4000 00037 #define BLOCK_COUNT (USB_RAM_SIZE/CHUNK_SIZE) 00038 #else 00039 #error "target error" 00040 #endif 00041 00042 static uint8_t* ram = NULL; 00043 static uint8_t* map; 00044 00045 static void usb_ram_init() 00046 { 00047 USB_INFO("USB_RAM: 0x%p(%d)", USB_RAM_BASE, USB_RAM_SIZE); 00048 ram = (uint8_t*)USB_RAM_BASE; 00049 TEST_ASSERT((int)ram%256 == 0); 00050 map = (uint8_t*)malloc(BLOCK_COUNT); 00051 TEST_ASSERT(map); 00052 memset(map, 0, BLOCK_COUNT); 00053 } 00054 00055 // first fit malloc 00056 void* usb_ram_malloc(size_t size, int aligment) 00057 { 00058 TEST_ASSERT(aligment >= 4); 00059 TEST_ASSERT(!(aligment & 3)); 00060 if (ram == NULL) { 00061 usb_ram_init(); 00062 } 00063 int needs = (size+CHUNK_SIZE-1)/CHUNK_SIZE; 00064 void* p = NULL; 00065 for(int idx = 0; idx < BLOCK_COUNT;) { 00066 bool found = true; 00067 for(int i = 0; i < needs; i++) { 00068 int block = map[idx + i]; 00069 if (block != 0) { 00070 idx += block; 00071 found = false; 00072 break; 00073 } 00074 } 00075 if (!found) { 00076 continue; 00077 } 00078 p = ram+idx*CHUNK_SIZE; 00079 if ((int)p % aligment) { 00080 idx++; 00081 continue; 00082 } 00083 TEST_ASSERT((idx + needs) <= BLOCK_COUNT); 00084 for(int i = 0; i < needs; i++) { 00085 map[idx + i] = needs - i; 00086 } 00087 break; 00088 } 00089 TEST_ASSERT(p); 00090 return p; 00091 } 00092 00093 void usb_ram_free(void* p) 00094 { 00095 TEST_ASSERT(p >= ram); 00096 TEST_ASSERT(p < (ram+CHUNK_SIZE*BLOCK_COUNT)); 00097 int idx = ((int)p-(int)ram)/CHUNK_SIZE; 00098 int block = map[idx]; 00099 TEST_ASSERT(block >= 1); 00100 for(int i =0; i < block; i++) { 00101 map[idx + i] = 0; 00102 } 00103 } 00104 00105 #endif // DBG_USE_POSIX_MEMALIGN 00106 00107
Generated on Tue Jul 12 2022 22:44:17 by
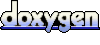