
ISP example program.
Dependencies: SLCD mbed USBLocalFileSystem
BaseLpcIsp.h
00001 #pragma once 00002 00003 #include "mbed.h" 00004 #include <stdarg.h> 00005 #include "mystring.h" 00006 00007 #define LF '\n' 00008 #define CRLF "\r\n" 00009 00010 #define LED_TX 0 00011 00012 class BaseLpcIsp { 00013 public: 00014 BaseLpcIsp(PinName txd, PinName rxd, PinName reset, PinName isp): 00015 _target(txd, rxd),_nreset(reset),_nisp(isp) { 00016 _wpos = _rpos = 0; 00017 _target.attach<BaseLpcIsp>(this, &BaseLpcIsp::callback); 00018 }; 00019 void Reset(bool isp); 00020 bool Sync(); 00021 bool FlashWrite(const char* filename); 00022 00023 void baud(int baudrate) { _target.baud(baudrate); } 00024 int putc(int c) { return _target.putc(c); } 00025 int readable() { return (_wpos != _rpos) ? 1 : 0; } 00026 int getc() { 00027 if (readable()) { 00028 int c = _buf[_rpos++]; 00029 if (_rpos >= sizeof(_buf)) { 00030 _rpos = 0; 00031 } 00032 return c; 00033 } 00034 return -1; 00035 } 00036 int puts(const char *str) { 00037 while(*str) { 00038 putc(*str++); 00039 } 00040 return 0; 00041 } 00042 00043 protected: 00044 uint32_t part_code; 00045 int chunk_size; 00046 int sector_size; 00047 bool plan_binary; 00048 virtual void infoLED(int select, int value) {} 00049 virtual void infoSLCD(const char* s) {} 00050 00051 private: 00052 void isp_error(int line) { 00053 char buf[5]; 00054 snprintf(buf, sizeof(buf), "E%3d", line); 00055 infoSLCD(buf); 00056 } 00057 bool _cmd(const char* format, ...); 00058 void sendln(const char* s); 00059 bool waitln(const char* s); 00060 bool recvln(char* buf, int size); 00061 bool recvln(mystring& s); 00062 bool _write_to_ram(int addr, uint8_t* buf, int size); 00063 bool _patch(int addr, uint8_t* buf, int size); 00064 RawSerial _target; 00065 char _buf[64]; 00066 __IO int _wpos; 00067 int _rpos; 00068 void callback() { 00069 int c = _target.getc(); 00070 _buf[_wpos++] = c; 00071 if (_wpos >= sizeof(_buf)) { 00072 _wpos = 0; 00073 } 00074 } 00075 uint8_t tx_sw; 00076 DigitalOut _nreset; 00077 DigitalOut _nisp; 00078 };
Generated on Sun Jul 24 2022 14:33:07 by
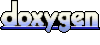