
IPS(Interpreter for Process Structures) for mbed
Dependencies: ConfigFile FATFileSystem mbed
BaseIPS.cpp
00001 // BaseIPS.cpp 2015/5/22 00002 #include <algorithm> 00003 #include "mbed.h" 00004 #include "BaseIPS.h" 00005 00006 #ifndef NO_MATH 00007 #include <math.h> 00008 #define Round(x) ( (x>=0) ? (int)(x+0.5) : (int)(x-0.5) ) 00009 #endif 00010 00011 BaseIPS::BaseIPS() { 00012 PS = 0xfff8; 00013 RS = 0x4f8; 00014 depth=0; 00015 idle=0; 00016 input_ptr = 512; 00017 cycle = 0; 00018 inputfile = NULL; 00019 } 00020 00021 u16 BaseIPS::peek(u16 a) { 00022 return peekB(a) | peekB(a + 1)<<8; 00023 } 00024 00025 void BaseIPS::poke(u16 a, u16 w) { 00026 pokeB(a, w & 0xff); 00027 pokeB(a + 1, w>>8); 00028 } 00029 00030 u32 BaseIPS::peek32(u16 a) { 00031 return peekB(a) | peekB(a + 1)<<8 | peekB(a + 2)<<16 | peekB(a + 3)<<24; 00032 } 00033 00034 00035 void BaseIPS::push_ps(u16 w) { 00036 PS -= 2; 00037 poke(PS, w); 00038 } 00039 00040 u16 BaseIPS::pull_ps() { 00041 PS += 2; 00042 return peek(PS - 2); 00043 } 00044 00045 void BaseIPS::push_rs(u16 w) { 00046 RS -= 2; 00047 poke(RS, w); 00048 } 00049 00050 u16 BaseIPS::pull_rs() { 00051 RS += 2; 00052 return peek(RS - 2); 00053 } 00054 00055 void BaseIPS::c_rumpelstilzchen(void) { // 0 00056 } 00057 00058 void BaseIPS::c_defex(void) { // 1 00059 push_rs(PPC); 00060 PPC = HP; 00061 depth++; 00062 } 00063 00064 void BaseIPS::c_consex(void) { // 2 00065 push_ps(peek(HP)); 00066 } 00067 00068 void BaseIPS::c_varex(void) { // 3 00069 push_ps(HP); 00070 } 00071 00072 void BaseIPS::c_retex(void) { // 4 00073 depth--; 00074 PPC = pull_rs(); 00075 } 00076 00077 void BaseIPS::c_get(void) { // 5 00078 poke(PS, peek(peek(PS))); 00079 } 00080 00081 void BaseIPS::c_getB(void) { // 6 00082 poke(PS, peekB(peek(PS))); 00083 } 00084 00085 void BaseIPS::c_put(void) { // 7 00086 u16 a = pull_ps(); 00087 u16 v = pull_ps(); 00088 if (peek(a) != v) idle=0; 00089 poke(a, v); 00090 } 00091 00092 void BaseIPS::c_putB(void) { // 8 00093 u16 a = pull_ps(); 00094 u16 v = pull_ps(); 00095 if (peekB(a) != v) idle=0; 00096 pokeB(a, v); 00097 idle=0; 00098 } 00099 00100 void BaseIPS::c_1bliteral(void) { // 9 00101 push_ps(peekB(PPC)); 00102 PPC++; 00103 } 00104 00105 void BaseIPS::c_2bliteral(void) { // 10 00106 push_ps(peek(PPC)); 00107 PPC += 2; 00108 } 00109 00110 void BaseIPS::c_bronz(void) { // 11 00111 if ((pull_ps() & 1) == 0) PPC=peek(PPC); 00112 else PPC += 2; 00113 } 00114 00115 void BaseIPS::c_jump(void) { // 12 00116 PPC = peek(PPC); 00117 } 00118 00119 void BaseIPS::c_weg(void) { // 13 00120 PS += 2; 00121 } 00122 00123 void BaseIPS::c_pweg(void) { // 14 00124 PS += 4; 00125 } 00126 00127 void BaseIPS::c_plus(void) { // 15 00128 s16 v = pull_ps(); 00129 poke(PS, peek(PS) + v); 00130 } 00131 00132 void BaseIPS::c_minus(void) { // 16 00133 s16 v = pull_ps(); 00134 poke(PS, peek(PS) - v); 00135 } 00136 00137 void BaseIPS::c_dup(void) { // 17 00138 push_ps(peek(PS)); 00139 } 00140 00141 void BaseIPS::c_pdup(void) { // 18 00142 push_ps(peek(PS + 2)); 00143 push_ps(peek(PS + 2)); 00144 } 00145 00146 void BaseIPS::c_vert(void) { // 19 00147 s16 h=peek(PS); 00148 poke(PS, peek(PS + 2)); 00149 poke(PS + 2, h); 00150 } 00151 00152 void BaseIPS::c_zwo(void) { // 20 00153 push_ps(peek(PS+2)); 00154 } 00155 00156 void BaseIPS::c_rdu(void) { // 21 00157 s16 h=peek(PS); 00158 poke(PS, peek(PS+2)); 00159 poke(PS+2, peek(PS+4)); 00160 poke(PS+4, h); 00161 } 00162 00163 void BaseIPS::c_rdo(void) { // 22 00164 s16 h=peek(PS+4); 00165 poke(PS+4, peek(PS+2)); 00166 poke(PS+2, peek(PS)); 00167 poke(PS, h); 00168 } 00169 00170 void BaseIPS::c_index(void) { // 23 00171 push_ps(peek(RS)); 00172 } 00173 00174 void BaseIPS::c_s_to_r(void) { // 24 00175 push_rs(pull_ps()); 00176 } 00177 00178 void BaseIPS::c_r_to_s(void) { // 25 00179 push_ps(pull_rs()); 00180 } 00181 00182 void BaseIPS::c_eqz(void) { // 26 00183 poke(PS,(peek(PS)==0)); 00184 } 00185 00186 void BaseIPS::c_gz(void) { // 27 00187 poke(PS,((s16)peek(PS)>0)); 00188 } 00189 00190 void BaseIPS::c_lz(void) { // 28 00191 poke(PS,((s16)peek(PS)<0)); 00192 } 00193 00194 void BaseIPS::c_geu(void) { // 29 00195 u16 a,b; 00196 b=pull_ps(); 00197 a=peek(PS); 00198 poke(PS,a>=b); 00199 } 00200 00201 void BaseIPS::c_f_vergl(void) { // 30 00202 int t; 00203 u16 n,a1,a2; 00204 n=pull_ps(); 00205 a2=pull_ps(); 00206 a1=peek(PS); 00207 t=1; 00208 do { 00209 int b; 00210 b=(s16)peekB(a1++)-(s16)peekB(a2++); 00211 if (b>0) t=2; 00212 else if (b<0) t=0; 00213 n=(n-1)&0xff; 00214 } while (n); 00215 poke(PS,t); 00216 } 00217 00218 void BaseIPS::c_nicht(void) { // 31 00219 poke(PS, 0xffff^peek(PS)); 00220 } 00221 00222 void BaseIPS::c_und(void) { // 32 00223 u16 v = pull_ps(); 00224 poke(PS, v & peek(PS)); 00225 } 00226 00227 void BaseIPS::c_oder(void) { // 33 00228 u16 v = pull_ps(); 00229 poke(PS, v | peek(PS)); 00230 } 00231 00232 void BaseIPS::c_exo(void) { // 34 00233 u16 v = pull_ps(); 00234 poke(PS, v ^ peek(PS)); 00235 } 00236 00237 void BaseIPS::c_bit(void) { // 35 00238 poke(PS, 1<<peek(PS)); 00239 } 00240 00241 void BaseIPS::c_cbit(void) { // 36 00242 int a = pull_ps(); 00243 int b = (pull_ps())&0x07; 00244 pokeB(a, peekB(a)&~(1<<b) ); 00245 } 00246 00247 void BaseIPS::c_sbit(void) { // 37 00248 int a,b; 00249 a=pull_ps(); 00250 b=(pull_ps())&0x07; 00251 pokeB(a, peekB(a)|(1<<b) ); 00252 } 00253 00254 void BaseIPS::c_tbit(void) { // 38 00255 int a,b; 00256 a=pull_ps(); 00257 b=(peek(PS))&0x07; 00258 if (peekB(a)&(1<<b)) poke(PS,1); 00259 else poke(PS,0); 00260 } 00261 00262 void BaseIPS::loop_sharedcode(int i) { 00263 int l; 00264 l=(s16)peek(RS); 00265 if (i<=l) { 00266 push_rs(i); 00267 PPC=peek(PPC); 00268 } else { 00269 pull_rs(); 00270 PPC+=2; 00271 } 00272 } 00273 00274 void BaseIPS::c_jeex(void) { // 39 00275 PPC=peek(PPC); 00276 push_rs(pull_ps()); 00277 loop_sharedcode((s16)pull_ps()); 00278 } 00279 00280 void BaseIPS::c_loopex(void) { // 40 00281 loop_sharedcode((s16)(pull_rs()+1)); 00282 } 00283 00284 void BaseIPS::c_plusloopex(void) { // 41 00285 int i; 00286 i=(s16)(pull_rs()); 00287 loop_sharedcode(i+(s16)pull_rs()); 00288 } 00289 00290 void BaseIPS::c_fieldtrans(void) { // 42 00291 /* note: cannot call memcpy() here, because memcpy()'s behaviour is not defined when source and destination areas overlap */ 00292 /* memmove() cannot be used either, because its behaviour for overlap is different from what IPS expects */ 00293 int n,d,s,b; 00294 n=pull_ps(); 00295 d=pull_ps(); 00296 s=pull_ps(); 00297 do { 00298 b=peekB(s++); 00299 pokeB(d++,b); 00300 n=(n-1)&0xff; 00301 } while (n); 00302 if (d<=0x400) redraw=1; 00303 idle=0; 00304 } 00305 00306 void BaseIPS::c_pmul(void) { // 43 00307 u32 c = pull_ps() * pull_ps(); 00308 push_ps((s16)(c&0xffff)); 00309 push_ps(c>>16); 00310 } 00311 00312 void BaseIPS::c_pdiv(void) { // 44 00313 u32 q,n; 00314 u16 d,nh,nl,r; 00315 d=pull_ps(); 00316 nh=pull_ps(); 00317 nl=pull_ps(); 00318 n=(nh<<16)+nl; 00319 if (d==0) { q=0xffff; r=0; } 00320 else { 00321 q=n/d; 00322 r=n%d; 00323 if (q>=0x10000) { q=0xffff; r=0; } 00324 } 00325 push_ps(q); 00326 push_ps(r); 00327 } 00328 00329 void BaseIPS::c_tue(void) { // 45 00330 HP = pull_ps(); 00331 } 00332 00333 void BaseIPS::c_polyname(void) { // 46 00334 u32 x,p,d; 00335 d=pull_ps(); 00336 x=pull_ps(); 00337 p=pull_ps(); 00338 x= x | (p&0xff00) | ((p&0xff)<<16); 00339 p = d ^ x ^ (x>>1) ^ (x>>2) ^ (x>>7); 00340 x = x ^ ((p&0xff)<<24); 00341 x >>= 7; 00342 push_ps( (x&0xff00) | ((x>>16)&0xff) ); 00343 push_ps( (x&0xff) ); 00344 } 00345 00346 void BaseIPS::c_scode(void) { // 47 00347 int i; 00348 u32 nd; 00349 int offs=peek(a_Os); 00350 i=peek(a_NDptr); 00351 nd=peek32(i); 00352 i=peek(a_P3); 00353 while (i) { 00354 i+=offs; 00355 if ( ( (peek32(i) ^ nd) & 0xffffff3f) ==0) { 00356 push_ps(i+6); 00357 return; 00358 } 00359 i=peek(i+4); 00360 } 00361 push_ps(0); 00362 } 00363 00364 void BaseIPS::c_cscan(void) { // 48 00365 int a; 00366 int pi,pe; 00367 int comment=0; 00368 pi=peek(a_PI); 00369 pe=peek(a_PE); 00370 00371 a=pull_ps(); 00372 if (a==1) { 00373 while (pi<=pe) { 00374 if (comment) { 00375 if (peekB(pi)==')') comment=0; 00376 } else { 00377 if (peekB(pi)=='(') comment=1; 00378 else if (peekB(pi)!=' ') { 00379 poke(a_PI,pi); 00380 push_ps(1); 00381 return; 00382 } 00383 } 00384 pi++; 00385 } 00386 push_ps(0); 00387 poke(a_P2,1); 00388 return; 00389 } else { 00390 while (pi<=pe) { 00391 if (peekB(pi)==' ') { 00392 break; 00393 } 00394 pi++; 00395 } 00396 poke(a_PI,pi); 00397 push_ps(0); 00398 } 00399 } 00400 00401 void BaseIPS::c_chs(void) { // 49 00402 poke(PS,-(s16)peek(PS)); 00403 } 00404 00405 void BaseIPS::c_cyc2(void) { // 50 00406 u32 ch,a,crcc; 00407 int i; 00408 00409 ch=pull_ps(); 00410 a=pull_ps(); 00411 crcc=(a>>8)|((a&0xff)<<8); 00412 00413 ch<<=8; 00414 for (i=0;i<=7;i++) { 00415 //int test; 00416 //test=(ch^crcc)&0x8000; 00417 crcc<<=1; 00418 ch<<=1; 00419 if ((ch^crcc)&0x10000) crcc^=0x1021; 00420 } 00421 00422 push_ps( ((crcc&0xff)<<8) | ((crcc>>8)&0xff) ); 00423 } 00424 00425 void BaseIPS::c_close(void) { // 51 00426 if (inputfile) { 00427 file_close(inputfile); 00428 inputfile = NULL; 00429 } 00430 } 00431 00432 void BaseIPS::c_open(void) { // 52 00433 if (inputfile) { 00434 file_close(inputfile); 00435 } 00436 size_t namelen = pull_ps(); 00437 int namestart = pull_ps(); 00438 char filename[256]; 00439 namelen = std::min(namelen, sizeof(filename)-1); 00440 for(size_t i = 0; i < namelen; i++) { 00441 filename[i] = peekB(namestart + i); 00442 } 00443 filename[namelen] = '\0'; 00444 inputfile = file_open(filename); 00445 push_ps(inputfile != NULL ? 1 : 0); 00446 } 00447 00448 void BaseIPS::c_oscli(void) { // 53 00449 error("OSCLI not implemented!"); 00450 } 00451 00452 void BaseIPS::c_load(void) { // 54 00453 error("LOAD not implemented!"); 00454 } 00455 00456 void BaseIPS::c_save(void) { // 55 00457 int namelen = pull_ps(); 00458 int namestart = pull_ps(); 00459 int end = pull_ps(); 00460 int start = pull_ps(); 00461 00462 char filename[256]; 00463 if (namelen > 255) namelen = 255; 00464 for(int i = 0; i < namelen; i++) { 00465 filename[i] = peekB(namestart + i); 00466 } 00467 filename[namelen] = 0; 00468 00469 void* fh = file_open(filename, "wb"); 00470 if (fh == NULL) { 00471 push_ps(0); 00472 return; 00473 } 00474 for(int i = start; i < end; i++) { 00475 file_putc(peekB(i), fh); 00476 } 00477 file_close(fh); 00478 push_ps(1); 00479 } 00480 00481 void BaseIPS::c_setkbptr(void) { // 56 00482 input_ptr = pull_ps() & 0x3ff; 00483 } 00484 00485 void BaseIPS::c_getPS(void) { // 57 00486 push_ps(PS); 00487 } 00488 00489 void BaseIPS::c_setPS(void) { // 58 00490 PS=pull_ps(); 00491 } 00492 00493 #ifndef NO_MATH 00494 void BaseIPS::c_rp_code(void) { // 59 00495 double theta,x,y,r; 00496 pull_ps(); 00497 y=(s16)pull_ps(); 00498 x=(s16)pull_ps(); 00499 theta=((s16)pull_ps())/10430.38; /* convert to radians */ 00500 theta+=atan2(y,x); 00501 r=sqrt(x*x+y*y)*1.6468; 00502 push_ps(Round(theta*10430.38)); 00503 push_ps((int)(r+0.5)); 00504 push_ps(0); 00505 } 00506 00507 void BaseIPS::c_tr_code(void) { // 60 00508 double theta,x,y,r; 00509 pull_ps(); 00510 y=(s16)pull_ps(); 00511 x=(s16)pull_ps(); 00512 theta=((s16)pull_ps())/10430.38; /* convert to radians */ 00513 theta+=atan2(y,x); 00514 r=sqrt(x*x+y*y)*1.6468; 00515 push_ps(0); 00516 push_ps(Round(r*cos(theta))); 00517 push_ps(Round(r*sin(theta))); 00518 } 00519 #else 00520 void BaseIPS::c_rp_code(void) { 00521 error("c_rp_code not implemented!\n"); 00522 } 00523 void BaseIPS::c_tr_code(void) { 00524 error("c_tr_code not implemented!\n"); 00525 } 00526 #endif 00527 00528 void BaseIPS::c_swap3(void) { // 61 00529 u16 h; 00530 h=peek(PS+6); poke(PS+6,peek(PS)); poke(PS,h); 00531 h=peek(PS+8); poke(PS+8,peek(PS+2)); poke(PS+2,h); 00532 h=peek(PS+10); poke(PS+10,peek(PS+4)); poke(PS+4,h); 00533 } 00534 00535 void BaseIPS::c_defchar(void) { // 62 00536 error("DEFCHAR not implemented!\n"); 00537 } 00538 00539 void BaseIPS::c_pplus(void) { // 63 00540 u32 h=pull_ps(); 00541 u32 b=(h<<16)+pull_ps(); 00542 h=pull_ps(); 00543 u32 a=(h<<16)+pull_ps(); 00544 u32 c=a+b; 00545 push_ps(c&0xffff); 00546 push_ps(c>>16); 00547 } 00548 00549 void BaseIPS::c_pminus(void) { // 64 00550 u32 h = pull_ps(); 00551 u32 b = (h<<16)+pull_ps(); 00552 h = pull_ps(); 00553 u32 a = (h<<16)+pull_ps(); 00554 u32 c = a - b; 00555 push_ps(c&0xffff); 00556 push_ps(c>>16); 00557 } 00558 00559 void BaseIPS::do_20ms(void) { 00560 pokeB(UHR, peekB(UHR) + 2); // 20ms 00561 if (peekB(UHR) == 100) { 00562 pokeB(UHR, 0); 00563 pokeB(UHR+1, peekB(UHR+1) + 1); 00564 if (peekB(UHR+1) == 60) { 00565 pokeB(UHR+1, 0); 00566 pokeB(UHR+2, peekB(UHR+2) + 1); 00567 if (peekB(UHR+2) == 60) { 00568 pokeB(UHR+2, 0); 00569 pokeB(UHR+3, peekB(UHR+3) + 1); 00570 if (peekB(UHR+3) == 24) { 00571 pokeB(UHR+3, 0); 00572 poke(UHR+4, peek(UHR+4) + 1); 00573 } 00574 } 00575 } 00576 } 00577 00578 const int sus[4]={SU0,SU1,SU2,SU3}; 00579 for (int i = 0; i < 4; i++) { 00580 int su=sus[i]; 00581 if ((peekB(su)&1) == 0) { 00582 if (peekB(su) != 0) { 00583 pokeB(su, peekB(su) - 2); 00584 } else { 00585 if (peekB(su+1) != 0) { 00586 pokeB(su, 98); 00587 pokeB(su+1, peekB(su+1) - 1); 00588 } else { 00589 if (peekB(su+2) != 0) { 00590 pokeB(su+1, 59); 00591 pokeB(su+2, peekB(su+2) - 1); 00592 } else { 00593 if (peekB(su+3) != 0) { 00594 pokeB(su+2, 255); 00595 pokeB(su+3, peekB(su+3) - 1); 00596 } else { 00597 pokeB(su, 1); 00598 } 00599 } 00600 } 00601 } 00602 } 00603 } 00604 } 00605 00606 void BaseIPS::read_inputfile(void) { 00607 for(int i = 0x200; i < 0x400; i++) { 00608 pokeB(i, ' '); 00609 } 00610 00611 do { 00612 int ch = file_getc(inputfile); 00613 if (ch<1) { 00614 if (input_ptr == peek(a_PI)) { 00615 file_close(inputfile); 00616 inputfile = NULL; 00617 pokeB(LOADFLAG, 0); 00618 input_ptr = 0x200; 00619 } 00620 break; 00621 } 00622 if (ch=='\r') { 00623 /* ignore \r, we expect at least also a \n as end-of-line */ 00624 } else if (ch=='\n') { 00625 input_ptr=(input_ptr&0xffc0)+64; 00626 } else { 00627 pokeB(input_ptr++,ch); 00628 } 00629 } while (input_ptr<1024 && inputfile); /* 1024 = TVE */ 00630 00631 if (input_ptr!=peek(a_PI)) { 00632 pokeB(READYFLAG,1); 00633 poke(a_PE,input_ptr-1); 00634 idle=0; 00635 } 00636 } 00637 00638 void BaseIPS::emulator() { 00639 PPC = 0x0400; 00640 input_ptr = peek(a_PI); 00641 while(1) { 00642 HP = peek(PPC); 00643 PPC += 2; 00644 exec: u16 CPC = peek(HP); 00645 HP += 2; 00646 trace(cycle++, PPC-2, HP-2, CPC, PS, RS); 00647 switch(CPC) { 00648 case 0: c_rumpelstilzchen(); break; 00649 case 1: c_defex(); break; 00650 case 2: c_consex(); break; 00651 case 3: c_varex(); break; 00652 case 4: c_retex(); break; 00653 case 5: c_get(); break; 00654 case 6: c_getB(); break; 00655 case 7: c_put(); break; 00656 case 8: c_putB(); break; 00657 case 9: c_1bliteral(); break; 00658 case 10: c_2bliteral(); break; 00659 case 11: c_bronz(); break; 00660 case 12: c_jump(); break; 00661 case 13: c_weg(); break; 00662 case 14: c_pweg(); break; 00663 case 15: c_plus(); break; 00664 case 16: c_minus(); break; 00665 case 17: c_dup(); break; 00666 case 18: c_pdup(); break; 00667 case 19: c_vert(); break; 00668 case 20: c_zwo(); break; 00669 case 21: c_rdu(); break; 00670 case 22: c_rdo(); break; 00671 case 23: c_index(); break; 00672 case 24: c_s_to_r(); break; 00673 case 25: c_r_to_s(); break; 00674 case 26: c_eqz(); break; 00675 case 27: c_gz(); break; 00676 case 28: c_lz(); break; 00677 case 29: c_geu(); break; 00678 case 30: c_f_vergl(); break; 00679 case 31: c_nicht(); break; 00680 case 32: c_und(); break; 00681 case 33: c_oder(); break; 00682 case 34: c_exo(); break; 00683 case 35: c_bit(); break; 00684 case 36: c_cbit(); break; 00685 case 37: c_sbit(); break; 00686 case 38: c_tbit(); break; 00687 case 39: c_jeex(); break; 00688 case 40: c_loopex(); break; 00689 case 41: c_plusloopex(); break; 00690 case 42: c_fieldtrans(); break; 00691 case 43: c_pmul(); break; 00692 case 44: c_pdiv(); break; 00693 case 45: c_tue(); goto exec; 00694 case 46: c_polyname(); break; 00695 case 47: c_scode(); break; 00696 case 48: c_cscan(); break; 00697 case 49: c_chs(); break; 00698 case 50: c_cyc2(); break; 00699 case 51: c_close(); break; 00700 case 52: c_open(); break; 00701 case 53: c_oscli(); break; 00702 case 54: c_load(); break; 00703 case 55: c_save(); break; 00704 case 56: c_setkbptr(); break; 00705 case 57: c_getPS(); break; 00706 case 58: c_setPS(); break; 00707 case 59: c_rp_code(); break; 00708 case 60: c_tr_code(); break; 00709 case 61: c_swap3(); break; 00710 case 62: c_defchar(); break; 00711 case 63: c_pplus(); break; 00712 case 64: c_pminus(); break; 00713 default: usercode(CPC); break; 00714 } 00715 do_io(); 00716 if (test_20ms()) { 00717 do_20ms(); 00718 } 00719 if (inputfile) { 00720 if (!(peekB(READYFLAG)&1) && (peekB(LOADFLAG)&1)) { 00721 read_inputfile(); 00722 } 00723 } 00724 } 00725 } 00726 00727 void BaseIPS::load_image(const u8* image, int size) { 00728 for(int i = 0; i < size; i++) { 00729 pokeB(i, *image++); 00730 } 00731 } 00732 00733 void BaseIPS::command(const char* s) { 00734 if (s) { 00735 int cmd_len = strlen(s); 00736 for(int i = 0; i < cmd_len; i++) { 00737 pokeB(i + 64*8, *s++); 00738 } 00739 poke(a_PE, 0x200 + cmd_len); 00740 pokeB(READYFLAG, 1); 00741 } 00742 } 00743 00744
Generated on Fri Jul 15 2022 06:25:55 by
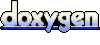