Simple USBHost library for Nucleo F446RE/F411RE/F401RE FRDM-KL46Z/KL25Z/F64F LPC4088/LPC1768
Dependents: F401RE-BTstack_example F401RE-USBHostMSD_HelloWorld
Fork of KL46Z-USBHost by
USBEndpoint.h
00001 /* mbed USBHost Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #pragma once 00018 #include "FunctionPointer.h" 00019 #include "USBHostTypes.h" 00020 #include "USBDeviceConnected.h" 00021 00022 class USBDeviceConnected; 00023 00024 /** 00025 * USBEndpoint class 00026 */ 00027 class USBEndpoint { 00028 public: 00029 /** 00030 * Constructor 00031 */ 00032 USBEndpoint(USBDeviceConnected* _dev) { 00033 init(CONTROL_ENDPOINT, IN, 8, 0); 00034 dev = _dev; 00035 pData = NULL; 00036 } 00037 00038 /** 00039 * Initialize an endpoint 00040 * 00041 * @param type endpoint type 00042 * @param dir endpoint direction 00043 * @param size endpoint size 00044 * @param ep_number endpoint number 00045 */ 00046 void init(ENDPOINT_TYPE _type, ENDPOINT_DIRECTION _dir, uint32_t size, uint8_t ep_number) { 00047 setState(USB_TYPE_FREE); 00048 setType(_type); 00049 dir = _dir; 00050 MaxPacketSize = size; 00051 address = ep_number; 00052 data01_toggle = DATA0; 00053 } 00054 00055 void ohci_init(uint8_t frameCount, uint8_t queueLimit) { 00056 ohci.frameCount = frameCount; 00057 ohci.queueLimit = queueLimit; 00058 } 00059 00060 /** 00061 * Attach a member function to call when a transfer is finished 00062 * 00063 * @param tptr pointer to the object to call the member function on 00064 * @param mptr pointer to the member function to be called 00065 */ 00066 template<typename T> 00067 inline void attach(T* tptr, void (T::*mptr)(void)) { 00068 if((mptr != NULL) && (tptr != NULL)) { 00069 rx.attach(tptr, mptr); 00070 } 00071 } 00072 00073 /** 00074 * Attach a callback called when a transfer is finished 00075 * 00076 * @param fptr function pointer 00077 */ 00078 inline void attach(void (*fptr)(void)) { 00079 if(fptr != NULL) { 00080 rx.attach(fptr); 00081 } 00082 } 00083 00084 /** 00085 * Call the handler associted to the end of a transfer 00086 */ 00087 inline void call() { 00088 rx.call(); 00089 }; 00090 00091 void setType(ENDPOINT_TYPE _type) { type = _type; } 00092 void setState(USB_TYPE st) { state = st; } 00093 void setDevice(USBDeviceConnected* _dev) { dev = _dev; } 00094 void setBuffer(uint8_t* buf, int size) { buf_start = buf, buf_size = size; } 00095 void setLengthTransferred(int len) { transferred = len; }; 00096 void setAddress(int addr) { address = addr; } 00097 void setSize(int size) { MaxPacketSize = size; } 00098 void setData01(uint8_t data01) { data01_toggle = data01; } 00099 void setNextEndpoint(USBEndpoint* ep) { nextEp = ep; }; 00100 template<class T> 00101 void setHALData(T data) { pData = data; } 00102 00103 USBDeviceConnected* getDevice() { return dev; } 00104 ENDPOINT_TYPE getType() { return type; }; 00105 USB_TYPE getState() { return state; } 00106 int getLengthTransferred() { return transferred; } 00107 uint8_t *getBufStart() { return buf_start; } 00108 int getBufSize() { return buf_size; } 00109 uint8_t getAddress(){ return address; }; 00110 int getSize() { return MaxPacketSize; } 00111 ENDPOINT_DIRECTION getDir() { return dir; } 00112 uint8_t getData01() { return data01_toggle; } 00113 void toggleData01() { 00114 data01_toggle = (data01_toggle == DATA0) ? DATA1 : DATA0; 00115 } 00116 USBEndpoint* nextEndpoint() { return nextEp; }; 00117 template<class T> 00118 T getHALData() { return reinterpret_cast<T>(pData); } 00119 00120 struct { 00121 uint8_t queueLimit; 00122 uint8_t frameCount; // 1-8 00123 } ohci; 00124 private: 00125 USBEndpoint(){} 00126 ENDPOINT_TYPE type; 00127 USB_TYPE state; 00128 ENDPOINT_DIRECTION dir; 00129 USBDeviceConnected* dev; 00130 uint8_t data01_toggle; // DATA0,DATA1 00131 uint8_t address; 00132 int transferred; 00133 uint8_t * buf_start; 00134 int buf_size; 00135 FunctionPointer rx; 00136 int MaxPacketSize; 00137 USBEndpoint* nextEp; 00138 void* pData; 00139 }; 00140 00141 class EndpointQueue { 00142 public: 00143 EndpointQueue():head(NULL),tail(NULL) {} 00144 void push(USBEndpoint* ep) { 00145 if (head) { 00146 tail->setNextEndpoint(ep); 00147 } else { 00148 head = ep; 00149 } 00150 tail = ep; 00151 ep->setNextEndpoint(NULL); 00152 } 00153 USBEndpoint* pop() { 00154 USBEndpoint* ep = head; 00155 if (ep) { 00156 head = ep->nextEndpoint(); 00157 } 00158 return ep; 00159 } 00160 bool empty() { return head == NULL; } 00161 00162 private: 00163 USBEndpoint* head; 00164 USBEndpoint* tail; 00165 }; 00166 00167
Generated on Wed Jul 13 2022 05:41:27 by
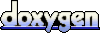