Simple USBHost library for Nucleo F446RE/F411RE/F401RE FRDM-KL46Z/KL25Z/F64F LPC4088/LPC1768
Dependents: F401RE-BTstack_example F401RE-USBHostMSD_HelloWorld
Fork of KL46Z-USBHost by
BaseUvc.cpp
00001 // BaseUvc.cpp 00002 #include "USBHostConf.h" 00003 #include "USBHost.h" 00004 #include "BaseUvc.h" 00005 00006 void BaseUvc::poll() { 00007 uint8_t buf[ep_iso_in->getSize()]; 00008 int result = host->isochronousReadNB(ep_iso_in, buf, sizeof(buf)); 00009 if (result >= 0) { 00010 const uint16_t frame = 0; 00011 onResult(frame, buf, ep_iso_in->getLengthTransferred()); 00012 } 00013 } 00014 00015 USB_TYPE BaseUvc::Control(int req, int cs, int index, uint8_t* buf, int size) { 00016 if (req == SET_CUR) { 00017 return host->controlWrite(dev, 00018 USB_HOST_TO_DEVICE | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_INTERFACE, 00019 req, cs<<8, index, buf, size); 00020 } 00021 return host->controlRead(dev, 00022 USB_DEVICE_TO_HOST | USB_REQUEST_TYPE_CLASS | USB_RECIPIENT_INTERFACE, 00023 req, cs<<8, index, buf, size); 00024 } 00025 00026 USB_TYPE BaseUvc::setInterfaceAlternate(uint8_t intf, uint8_t alt) { 00027 return host->controlWrite(dev, USB_HOST_TO_DEVICE | USB_RECIPIENT_INTERFACE, 00028 SET_INTERFACE, alt, intf, NULL, 0); 00029 } 00030 00031 void BaseUvc::onResult(uint16_t frame, uint8_t* buf, int len) 00032 { 00033 if(m_pCbItem && m_pCbMeth) 00034 (m_pCbItem->*m_pCbMeth)(frame, buf, len); 00035 else if(m_pCb) 00036 m_pCb(frame, buf, len); 00037 } 00038 00039 void BaseUvc::setOnResult( void (*pMethod)(uint16_t, uint8_t*, int) ) 00040 { 00041 m_pCb = pMethod; 00042 m_pCbItem = NULL; 00043 m_pCbMeth = NULL; 00044 } 00045 00046 void BaseUvc::clearOnResult() 00047 { 00048 m_pCb = NULL; 00049 m_pCbItem = NULL; 00050 m_pCbMeth = NULL; 00051 } 00052
Generated on Wed Jul 13 2022 05:41:27 by
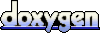