
BaseUsbHost example program
Dependencies: BaseUsbHost FATFileSystem mbed mbed-rtos
MyThread.h
00001 // MyThread.h 2012/12/9 00002 #ifndef MY_THREAD_H 00003 #define MY_THREAD_H 00004 00005 #define MAGIC_WORD 0xE25A2EA5 00006 static void thread_handler(void const *argument); 00007 00008 class MyThread { 00009 public: 00010 MyThread() { 00011 m_stack_size = DEFAULT_STACK_SIZE; 00012 m_stack_pointer = NULL; 00013 } 00014 void set_stack(uint32_t stack_size=DEFAULT_STACK_SIZE, uint8_t* stack_pointer = NULL) { 00015 m_stack_size = stack_size; 00016 m_stack_pointer = stack_pointer; 00017 } 00018 virtual void run() = 0; 00019 Thread* start(osPriority priority=osPriorityNormal) { 00020 if (m_stack_pointer == NULL) { 00021 m_stack_pointer = reinterpret_cast<uint8_t*>(malloc(m_stack_size)); 00022 } 00023 for(int i = 0; i < m_stack_size-64; i += 4) { 00024 *reinterpret_cast<uint32_t*>(m_stack_pointer+i) = MAGIC_WORD; 00025 } 00026 return th = new Thread(thread_handler, this, priority, m_stack_size, m_stack_pointer); 00027 } 00028 00029 int stack_used() { 00030 int i; 00031 for(i = 0; i < m_stack_size; i += 4) { 00032 if(*reinterpret_cast<uint32_t*>(m_stack_pointer+i) != MAGIC_WORD) { 00033 break; 00034 } 00035 } 00036 return m_stack_size - i; 00037 } 00038 00039 int stack_size() { return m_stack_size; } 00040 protected: 00041 Thread* th; 00042 uint32_t m_stack_size; 00043 uint8_t* m_stack_pointer; 00044 }; 00045 static void thread_handler(void const *argument) { 00046 MyThread* th = (MyThread*)argument; 00047 if (th) { 00048 th->run(); 00049 } 00050 } 00051 00052 #endif //MY_THREAD_H
Generated on Wed Jul 13 2022 00:00:06 by
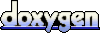