
BTstack Bluetooth stack
Embed:
(wiki syntax)
Show/hide line numbers
sdp.h
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 #pragma once 00037 00038 #include <stdint.h> 00039 #include <btstack/linked_list.h> 00040 00041 #include "config.h" 00042 00043 typedef enum { 00044 SDP_ErrorResponse = 1, 00045 SDP_ServiceSearchRequest, 00046 SDP_ServiceSearchResponse, 00047 SDP_ServiceAttributeRequest, 00048 SDP_ServiceAttributeResponse, 00049 SDP_ServiceSearchAttributeRequest, 00050 SDP_ServiceSearchAttributeResponse 00051 } SDP_PDU_ID_t; 00052 00053 // service record 00054 // -- uses user_data field for actual 00055 typedef struct { 00056 // linked list - assert: first field 00057 linked_item_t item; 00058 00059 // client connection 00060 void * connection; 00061 00062 // data is contained in same memory 00063 uint32_t service_record_handle; 00064 uint8_t service_record[0]; 00065 } service_record_item_t; 00066 00067 00068 void sdp_init(void); 00069 00070 void sdp_register_packet_handler(void (*handler)(void * connection, uint8_t packet_type, 00071 uint16_t channel, uint8_t *packet, uint16_t size)); 00072 00073 #ifdef EMBEDDED 00074 // register service record internally - the normal version creates a copy of the record 00075 // pre: AttributeIDs are in ascending order => ServiceRecordHandle is first attribute if present 00076 // @returns ServiceRecordHandle or 0 if registration failed 00077 uint32_t sdp_register_service_internal(void *connection, service_record_item_t * record_item); 00078 #else 00079 // register service record internally - this special version doesn't copy the record, it cannot be freeed 00080 // pre: AttributeIDs are in ascending order 00081 // pre: ServiceRecordHandle is first attribute and valid 00082 // pre: record 00083 // @returns ServiceRecordHandle or 0 if registration failed 00084 uint32_t sdp_register_service_internal(void *connection, uint8_t * service_record); 00085 #endif 00086 00087 // unregister service record internally 00088 void sdp_unregister_service_internal(void *connection, uint32_t service_record_handle); 00089 00090 // 00091 void sdp_unregister_services_for_connection(void *connection); 00092 00093 // 00094 int sdp_handle_service_search_request(uint8_t * packet, uint16_t remote_mtu); 00095 int sdp_handle_service_attribute_request(uint8_t * packet, uint16_t remote_mtu); 00096 int sdp_handle_service_search_attribute_request(uint8_t * packet, uint16_t remote_mtu);
Generated on Tue Jul 12 2022 15:55:29 by
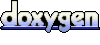