
BTstack Bluetooth stack
Embed:
(wiki syntax)
Show/hide line numbers
l2cap_signaling.c
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 /* 00038 * l2cap_signaling.h 00039 * 00040 * Created by Matthias Ringwald on 7/23/09. 00041 */ 00042 00043 #include "l2cap_signaling.h" 00044 00045 #include <string.h> 00046 00047 static char *l2cap_signaling_commands_format[] = { 00048 "D", // 0x01 command reject: reason {cmd not understood (0), sig MTU exceeded (2:max sig MTU), invalid CID (4:req CID)}, data len, data 00049 "22", // 0x02 connection request: PSM, Source CID 00050 "2222", // 0x03 connection response: Dest CID, Source CID, Result, Status 00051 "22D", // 0x04 config request: Dest CID, Flags, Configuration options 00052 "222D", // 0x05 config response: Source CID, Flags, Result, Configuration options 00053 "22", // 0x06 disconection request: Dest CID, Source CID 00054 "22", // 0x07 disconection response: Dest CID, Source CID 00055 "D", // 0x08 echo request: Data 00056 "D", // 0x09 echo response: Data 00057 "2", // 0x0a information request: InfoType {1=Connectionless MTU, 2=Extended features supported} 00058 "22D", // 0x0b information response: InfoType, Result, Data 00059 }; 00060 00061 uint8_t sig_seq_nr = 0xff; 00062 uint16_t source_cid = 0x40; 00063 00064 uint8_t l2cap_next_sig_id(void){ 00065 if (sig_seq_nr == 0xff) { 00066 sig_seq_nr = 1; 00067 } else { 00068 sig_seq_nr++; 00069 } 00070 return sig_seq_nr; 00071 } 00072 00073 uint16_t l2cap_next_local_cid(void){ 00074 return source_cid++; 00075 } 00076 00077 uint16_t l2cap_create_signaling_internal(uint8_t * acl_buffer, hci_con_handle_t handle, L2CAP_SIGNALING_COMMANDS cmd, uint8_t identifier, va_list argptr){ 00078 00079 // 0 - Connection handle : PB=10 : BC=00 00080 bt_store_16(acl_buffer, 0, handle | (2 << 12) | (0 << 14)); 00081 // 6 - L2CAP channel = 1 00082 bt_store_16(acl_buffer, 6, 1); 00083 // 8 - Code 00084 acl_buffer[8] = cmd; 00085 // 9 - id (!= 0 sequentially) 00086 acl_buffer[9] = identifier; 00087 00088 // 12 - L2CAP signaling parameters 00089 uint16_t pos = 12; 00090 const char *format = l2cap_signaling_commands_format[cmd-1]; 00091 uint16_t word; 00092 uint8_t * ptr; 00093 while (*format) { 00094 switch(*format) { 00095 case '1': // 8 bit value 00096 case '2': // 16 bit value 00097 word = va_arg(argptr, int); 00098 // minimal va_arg is int: 2 bytes on 8+16 bit CPUs 00099 acl_buffer[pos++] = word & 0xff; 00100 if (*format == '2') { 00101 acl_buffer[pos++] = word >> 8; 00102 } 00103 break; 00104 case 'D': // variable data. passed: len, ptr 00105 word = va_arg(argptr, int); 00106 ptr = va_arg(argptr, uint8_t *); 00107 memcpy(&acl_buffer[pos], ptr, word); 00108 pos += word; 00109 break; 00110 default: 00111 break; 00112 } 00113 format++; 00114 }; 00115 va_end(argptr); 00116 00117 // Fill in various length fields: it's the number of bytes following for ACL lenght and l2cap parameter length 00118 // - the l2cap payload length is counted after the following channel id (only payload) 00119 00120 // 2 - ACL length 00121 bt_store_16(acl_buffer, 2, pos - 4); 00122 // 4 - L2CAP packet length 00123 bt_store_16(acl_buffer, 4, pos - 6 - 2); 00124 // 10 - L2CAP signaling parameter length 00125 bt_store_16(acl_buffer, 10, pos - 12); 00126 00127 return pos; 00128 }
Generated on Tue Jul 12 2022 15:55:29 by
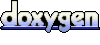