
BTstack Bluetooth stack
Embed:
(wiki syntax)
Show/hide line numbers
hci.c
00001 /* 00002 * Copyright (C) 2009-2012 by Matthias Ringwald 00003 * 00004 * Redistribution and use in source and binary forms, with or without 00005 * modification, are permitted provided that the following conditions 00006 * are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright 00009 * notice, this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright 00011 * notice, this list of conditions and the following disclaimer in the 00012 * documentation and/or other materials provided with the distribution. 00013 * 3. Neither the name of the copyright holders nor the names of 00014 * contributors may be used to endorse or promote products derived 00015 * from this software without specific prior written permission. 00016 * 4. Any redistribution, use, or modification is done solely for 00017 * personal benefit and not for any commercial purpose or for 00018 * monetary gain. 00019 * 00020 * THIS SOFTWARE IS PROVIDED BY MATTHIAS RINGWALD AND CONTRIBUTORS 00021 * ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00022 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS 00023 * FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL MATTHIAS 00024 * RINGWALD OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00025 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, 00026 * BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS 00027 * OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED 00028 * AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF 00030 * THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF 00031 * SUCH DAMAGE. 00032 * 00033 * Please inquire about commercial licensing options at btstack@ringwald.ch 00034 * 00035 */ 00036 00037 /* 00038 * hci.c 00039 * 00040 * Created by Matthias Ringwald on 4/29/09. 00041 * 00042 */ 00043 00044 #include "config.h" 00045 00046 #include "hci.h" 00047 00048 #include <stdarg.h> 00049 #include <string.h> 00050 #include <stdio.h> 00051 00052 #ifndef EMBEDDED 00053 #include <unistd.h> // gethostbyname 00054 #include <btstack/version.h> 00055 #endif 00056 00057 #include "btstack_memory.h" 00058 #include "debug.h" 00059 #include "hci_dump.h" 00060 00061 #include <btstack/hci_cmds.h> 00062 00063 #define HCI_CONNECTION_TIMEOUT_MS 10000 00064 00065 #ifdef USE_BLUETOOL 00066 #include "bt_control_iphone.h" 00067 #endif 00068 00069 static void hci_update_scan_enable(void); 00070 00071 // the STACK is here 00072 static hci_stack_t hci_stack; 00073 00074 /** 00075 * get connection for a given handle 00076 * 00077 * @return connection OR NULL, if not found 00078 */ 00079 hci_connection_t * connection_for_handle(hci_con_handle_t con_handle){ 00080 linked_item_t *it; 00081 for (it = (linked_item_t *) hci_stack.connections; it ; it = it->next){ 00082 if ( ((hci_connection_t *) it)->con_handle == con_handle){ 00083 return (hci_connection_t *) it; 00084 } 00085 } 00086 return NULL; 00087 } 00088 00089 static void hci_connection_timeout_handler(timer_source_t *timer){ 00090 hci_connection_t * connection = (hci_connection_t *) linked_item_get_user(&timer->item); 00091 #ifdef HAVE_TIME 00092 struct timeval tv; 00093 gettimeofday(&tv, NULL); 00094 if (tv.tv_sec >= connection->timestamp.tv_sec + HCI_CONNECTION_TIMEOUT_MS/1000) { 00095 // connections might be timed out 00096 hci_emit_l2cap_check_timeout(connection); 00097 } 00098 #endif 00099 #ifdef HAVE_TICK 00100 if (embedded_get_ticks() > connection->timestamp + embedded_ticks_for_ms(HCI_CONNECTION_TIMEOUT_MS)){ 00101 // connections might be timed out 00102 hci_emit_l2cap_check_timeout(connection); 00103 } 00104 #endif 00105 run_loop_set_timer(timer, HCI_CONNECTION_TIMEOUT_MS); 00106 run_loop_add_timer(timer); 00107 } 00108 00109 static void hci_connection_timestamp(hci_connection_t *connection){ 00110 #ifdef HAVE_TIME 00111 gettimeofday(&connection->timestamp, NULL); 00112 #endif 00113 #ifdef HAVE_TICK 00114 connection->timestamp = embedded_get_ticks(); 00115 #endif 00116 } 00117 00118 /** 00119 * create connection for given address 00120 * 00121 * @return connection OR NULL, if no memory left 00122 */ 00123 static hci_connection_t * create_connection_for_addr(bd_addr_t addr){ 00124 hci_connection_t * conn = (hci_connection_t *) btstack_memory_hci_connection_get(); 00125 if (!conn) return NULL; 00126 BD_ADDR_COPY(conn->address, addr); 00127 conn->con_handle = 0xffff; 00128 conn->authentication_flags = AUTH_FLAGS_NONE; 00129 linked_item_set_user(&conn->timeout.item, conn); 00130 conn->timeout.process = hci_connection_timeout_handler; 00131 hci_connection_timestamp(conn); 00132 conn->acl_recombination_length = 0; 00133 conn->acl_recombination_pos = 0; 00134 conn->num_acl_packets_sent = 0; 00135 linked_list_add(&hci_stack.connections, (linked_item_t *) conn); 00136 return conn; 00137 } 00138 00139 /** 00140 * get connection for given address 00141 * 00142 * @return connection OR NULL, if not found 00143 */ 00144 static hci_connection_t * connection_for_address(bd_addr_t address){ 00145 linked_item_t *it; 00146 for (it = (linked_item_t *) hci_stack.connections; it ; it = it->next){ 00147 if ( ! BD_ADDR_CMP( ((hci_connection_t *) it)->address, address) ){ 00148 return (hci_connection_t *) it; 00149 } 00150 } 00151 return NULL; 00152 } 00153 00154 inline static void connectionSetAuthenticationFlags(hci_connection_t * conn, hci_authentication_flags_t flags){ 00155 conn->authentication_flags = (hci_authentication_flags_t)(conn->authentication_flags | flags); 00156 } 00157 00158 inline static void connectionClearAuthenticationFlags(hci_connection_t * conn, hci_authentication_flags_t flags){ 00159 conn->authentication_flags = (hci_authentication_flags_t)(conn->authentication_flags & ~flags); 00160 } 00161 00162 00163 /** 00164 * add authentication flags and reset timer 00165 */ 00166 static void hci_add_connection_flags_for_flipped_bd_addr(uint8_t *bd_addr, hci_authentication_flags_t flags){ 00167 bd_addr_t addr; 00168 bt_flip_addr(addr, *(bd_addr_t *) bd_addr); 00169 hci_connection_t * conn = connection_for_address(addr); 00170 if (conn) { 00171 connectionSetAuthenticationFlags(conn, flags); 00172 hci_connection_timestamp(conn); 00173 } 00174 } 00175 00176 int hci_authentication_active_for_handle(hci_con_handle_t handle){ 00177 hci_connection_t * conn = connection_for_handle(handle); 00178 if (!conn) return 0; 00179 if (!conn->authentication_flags) return 0; 00180 if (conn->authentication_flags & SENT_LINK_KEY_REPLY) return 0; 00181 if (conn->authentication_flags & RECV_LINK_KEY_NOTIFICATION) return 0; 00182 return 1; 00183 } 00184 00185 void hci_drop_link_key_for_bd_addr(bd_addr_t *addr){ 00186 if (hci_stack.remote_device_db) { 00187 hci_stack.remote_device_db->delete_link_key(addr); 00188 } 00189 } 00190 00191 00192 /** 00193 * count connections 00194 */ 00195 static int nr_hci_connections(void){ 00196 int count = 0; 00197 linked_item_t *it; 00198 for (it = (linked_item_t *) hci_stack.connections; it ; it = it->next, count++); 00199 return count; 00200 } 00201 00202 /** 00203 * Dummy handler called by HCI 00204 */ 00205 static void dummy_handler(uint8_t packet_type, uint8_t *packet, uint16_t size){ 00206 } 00207 00208 uint8_t hci_number_outgoing_packets(hci_con_handle_t handle){ 00209 hci_connection_t * connection = connection_for_handle(handle); 00210 if (!connection) { 00211 log_error("hci_number_outgoing_packets connectino for handle %u does not exist!\n", handle); 00212 return 0; 00213 } 00214 return connection->num_acl_packets_sent; 00215 } 00216 00217 uint8_t hci_number_free_acl_slots(){ 00218 uint8_t free_slots = hci_stack.total_num_acl_packets; 00219 linked_item_t *it; 00220 for (it = (linked_item_t *) hci_stack.connections; it ; it = it->next){ 00221 hci_connection_t * connection = (hci_connection_t *) it; 00222 if (free_slots < connection->num_acl_packets_sent) { 00223 log_error("hci_number_free_acl_slots: sum of outgoing packets > total acl packets!\n"); 00224 return 0; 00225 } 00226 free_slots -= connection->num_acl_packets_sent; 00227 } 00228 return free_slots; 00229 } 00230 00231 int hci_can_send_packet_now(uint8_t packet_type){ 00232 00233 // check for async hci transport implementations 00234 if (hci_stack.hci_transport->can_send_packet_now){ 00235 if (!hci_stack.hci_transport->can_send_packet_now(packet_type)){ 00236 return 0; 00237 } 00238 } 00239 00240 // check regular Bluetooth flow control 00241 switch (packet_type) { 00242 case HCI_ACL_DATA_PACKET: 00243 return hci_number_free_acl_slots(); 00244 case HCI_COMMAND_DATA_PACKET: 00245 return hci_stack.num_cmd_packets; 00246 default: 00247 return 0; 00248 } 00249 } 00250 00251 int hci_send_acl_packet(uint8_t *packet, int size){ 00252 00253 // check for free places on BT module 00254 if (!hci_number_free_acl_slots()) return BTSTACK_ACL_BUFFERS_FULL; 00255 00256 hci_con_handle_t con_handle = READ_ACL_CONNECTION_HANDLE(packet); 00257 hci_connection_t *connection = connection_for_handle( con_handle); 00258 if (!connection) return 0; 00259 hci_connection_timestamp(connection); 00260 00261 // count packet 00262 connection->num_acl_packets_sent++; 00263 // log_info("hci_send_acl_packet - handle %u, sent %u\n", connection->con_handle, connection->num_acl_packets_sent); 00264 00265 // send packet 00266 int err = hci_stack.hci_transport->send_packet(HCI_ACL_DATA_PACKET, packet, size); 00267 00268 return err; 00269 } 00270 00271 static void acl_handler(uint8_t *packet, int size){ 00272 00273 // get info 00274 hci_con_handle_t con_handle = READ_ACL_CONNECTION_HANDLE(packet); 00275 hci_connection_t *conn = connection_for_handle(con_handle); 00276 uint8_t acl_flags = READ_ACL_FLAGS(packet); 00277 uint16_t acl_length = READ_ACL_LENGTH(packet); 00278 00279 // ignore non-registered handle 00280 if (!conn){ 00281 log_error( "hci.c: acl_handler called with non-registered handle %u!\n" , con_handle); 00282 return; 00283 } 00284 00285 // update idle timestamp 00286 hci_connection_timestamp(conn); 00287 00288 // handle different packet types 00289 switch (acl_flags & 0x03) { 00290 00291 case 0x01: // continuation fragment 00292 00293 // sanity check 00294 if (conn->acl_recombination_pos == 0) { 00295 log_error( "ACL Cont Fragment but no first fragment for handle 0x%02x\n", con_handle); 00296 return; 00297 } 00298 00299 // append fragment payload (header already stored) 00300 memcpy(&conn->acl_recombination_buffer[conn->acl_recombination_pos], &packet[4], acl_length ); 00301 conn->acl_recombination_pos += acl_length; 00302 00303 // log_error( "ACL Cont Fragment: acl_len %u, combined_len %u, l2cap_len %u\n", acl_length, 00304 // conn->acl_recombination_pos, conn->acl_recombination_length); 00305 00306 // forward complete L2CAP packet if complete. 00307 if (conn->acl_recombination_pos >= conn->acl_recombination_length + 4 + 4){ // pos already incl. ACL header 00308 00309 hci_stack.packet_handler(HCI_ACL_DATA_PACKET, conn->acl_recombination_buffer, conn->acl_recombination_pos); 00310 // reset recombination buffer 00311 conn->acl_recombination_length = 0; 00312 conn->acl_recombination_pos = 0; 00313 } 00314 break; 00315 00316 case 0x02: { // first fragment 00317 00318 // sanity check 00319 if (conn->acl_recombination_pos) { 00320 log_error( "ACL First Fragment but data in buffer for handle 0x%02x\n", con_handle); 00321 return; 00322 } 00323 00324 // peek into L2CAP packet! 00325 uint16_t l2cap_length = READ_L2CAP_LENGTH( packet ); 00326 00327 // log_error( "ACL First Fragment: acl_len %u, l2cap_len %u\n", acl_length, l2cap_length); 00328 00329 // compare fragment size to L2CAP packet size 00330 if (acl_length >= l2cap_length + 4){ 00331 00332 // forward fragment as L2CAP packet 00333 hci_stack.packet_handler(HCI_ACL_DATA_PACKET, packet, acl_length + 4); 00334 00335 } else { 00336 // store first fragment and tweak acl length for complete package 00337 memcpy(conn->acl_recombination_buffer, packet, acl_length + 4); 00338 conn->acl_recombination_pos = acl_length + 4; 00339 conn->acl_recombination_length = l2cap_length; 00340 bt_store_16(conn->acl_recombination_buffer, 2, l2cap_length +4); 00341 } 00342 break; 00343 00344 } 00345 default: 00346 log_error( "hci.c: acl_handler called with invalid packet boundary flags %u\n", acl_flags & 0x03); 00347 return; 00348 } 00349 00350 // execute main loop 00351 hci_run(); 00352 } 00353 00354 static void hci_shutdown_connection(hci_connection_t *conn){ 00355 log_info("Connection closed: handle %u, %s\n", conn->con_handle, bd_addr_to_str(conn->address)); 00356 00357 // cancel all l2cap connections 00358 hci_emit_disconnection_complete(conn->con_handle, 0x16); // terminated by local host 00359 00360 run_loop_remove_timer(&conn->timeout); 00361 00362 linked_list_remove(&hci_stack.connections, (linked_item_t *) conn); 00363 btstack_memory_hci_connection_free( conn ); 00364 00365 // now it's gone 00366 hci_emit_nr_connections_changed(); 00367 } 00368 00369 static const uint16_t packet_type_sizes[] = { 00370 0, HCI_ACL_2DH1_SIZE, HCI_ACL_3DH1_SIZE, HCI_ACL_DM1_SIZE, 00371 HCI_ACL_DH1_SIZE, 0, 0, 0, 00372 HCI_ACL_2DH3_SIZE, HCI_ACL_3DH3_SIZE, HCI_ACL_DM3_SIZE, HCI_ACL_DH3_SIZE, 00373 HCI_ACL_2DH5_SIZE, HCI_ACL_3DH5_SIZE, HCI_ACL_DM5_SIZE, HCI_ACL_DH5_SIZE 00374 }; 00375 00376 static uint16_t hci_acl_packet_types_for_buffer_size(uint16_t buffer_size){ 00377 uint16_t packet_types = 0; 00378 int i; 00379 for (i=0;i<16;i++){ 00380 if (packet_type_sizes[i] == 0) continue; 00381 if (packet_type_sizes[i] <= buffer_size){ 00382 packet_types |= 1 << i; 00383 } 00384 } 00385 // flip bits for "may not be used" 00386 packet_types ^= 0x3306; 00387 return packet_types; 00388 } 00389 00390 uint16_t hci_usable_acl_packet_types(void){ 00391 return hci_stack.packet_types; 00392 } 00393 00394 uint8_t* hci_get_outgoing_acl_packet_buffer(void){ 00395 // hci packet buffer is >= acl data packet length 00396 return hci_stack.hci_packet_buffer; 00397 } 00398 00399 uint16_t hci_max_acl_data_packet_length(){ 00400 return hci_stack.acl_data_packet_length; 00401 } 00402 00403 // avoid huge local variables 00404 #ifndef EMBEDDED 00405 static device_name_t device_name; 00406 #endif 00407 static void event_handler(uint8_t *packet, int size){ 00408 bd_addr_t addr; 00409 uint8_t link_type; 00410 hci_con_handle_t handle; 00411 hci_connection_t * conn; 00412 int i; 00413 00414 // printf("HCI:EVENT:%02x\n", packet[0]); 00415 00416 switch (packet[0]) { 00417 00418 case HCI_EVENT_COMMAND_COMPLETE: 00419 // get num cmd packets 00420 // log_info("HCI_EVENT_COMMAND_COMPLETE cmds old %u - new %u\n", hci_stack.num_cmd_packets, packet[2]); 00421 hci_stack.num_cmd_packets = packet[2]; 00422 00423 if (COMMAND_COMPLETE_EVENT(packet, hci_read_buffer_size)){ 00424 // from offset 5 00425 // status 00426 // "The HC_ACL_Data_Packet_Length return parameter will be used to determine the size of the L2CAP segments contained in ACL Data Packets" 00427 hci_stack.acl_data_packet_length = READ_BT_16(packet, 6); 00428 // ignore: SCO data packet len (8) 00429 hci_stack.total_num_acl_packets = packet[9]; 00430 // ignore: total num SCO packets 00431 if (hci_stack.state == HCI_STATE_INITIALIZING){ 00432 // determine usable ACL payload size 00433 if (HCI_ACL_PAYLOAD_SIZE < hci_stack.acl_data_packet_length){ 00434 hci_stack.acl_data_packet_length = HCI_ACL_PAYLOAD_SIZE; 00435 } 00436 // determine usable ACL packet types 00437 hci_stack.packet_types = hci_acl_packet_types_for_buffer_size(hci_stack.acl_data_packet_length); 00438 00439 log_error("hci_read_buffer_size: used size %u, count %u, packet types %04x\n", 00440 hci_stack.acl_data_packet_length, hci_stack.total_num_acl_packets, hci_stack.packet_types); 00441 } 00442 } 00443 // Dump local address 00444 if (COMMAND_COMPLETE_EVENT(packet, hci_read_bd_addr)) { 00445 bd_addr_t addr; 00446 bt_flip_addr(addr, &packet[OFFSET_OF_DATA_IN_COMMAND_COMPLETE + 1]); 00447 log_info("Local Address, Status: 0x%02x: Addr: %s\n", 00448 packet[OFFSET_OF_DATA_IN_COMMAND_COMPLETE], bd_addr_to_str(addr)); 00449 } 00450 if (COMMAND_COMPLETE_EVENT(packet, hci_write_scan_enable)){ 00451 hci_emit_discoverable_enabled(hci_stack.discoverable); 00452 } 00453 break; 00454 00455 case HCI_EVENT_COMMAND_STATUS: 00456 // get num cmd packets 00457 // log_info("HCI_EVENT_COMMAND_STATUS cmds - old %u - new %u\n", hci_stack.num_cmd_packets, packet[3]); 00458 hci_stack.num_cmd_packets = packet[3]; 00459 break; 00460 00461 case HCI_EVENT_NUMBER_OF_COMPLETED_PACKETS: 00462 for (i=0; i<packet[2];i++){ 00463 handle = READ_BT_16(packet, 3 + 2*i); 00464 uint16_t num_packets = READ_BT_16(packet, 3 + packet[2]*2 + 2*i); 00465 conn = connection_for_handle(handle); 00466 if (!conn){ 00467 log_error("hci_number_completed_packet lists unused con handle %u\n", handle); 00468 continue; 00469 } 00470 conn->num_acl_packets_sent -= num_packets; 00471 // log_info("hci_number_completed_packet %u processed for handle %u, outstanding %u\n", num_packets, handle, conn->num_acl_packets_sent); 00472 } 00473 break; 00474 00475 case HCI_EVENT_CONNECTION_REQUEST: 00476 bt_flip_addr(addr, &packet[2]); 00477 // TODO: eval COD 8-10 00478 link_type = packet[11]; 00479 log_info("Connection_incoming: %s, type %u\n", bd_addr_to_str(addr), link_type); 00480 if (link_type == 1) { // ACL 00481 conn = connection_for_address(addr); 00482 if (!conn) { 00483 conn = create_connection_for_addr(addr); 00484 } 00485 if (!conn) { 00486 // CONNECTION REJECTED DUE TO LIMITED RESOURCES (0X0D) 00487 hci_stack.decline_reason = 0x0d; 00488 BD_ADDR_COPY(hci_stack.decline_addr, addr); 00489 break; 00490 } 00491 conn->state = RECEIVED_CONNECTION_REQUEST; 00492 hci_run(); 00493 } else { 00494 // SYNCHRONOUS CONNECTION LIMIT TO A DEVICE EXCEEDED (0X0A) 00495 hci_stack.decline_reason = 0x0a; 00496 BD_ADDR_COPY(hci_stack.decline_addr, addr); 00497 } 00498 break; 00499 00500 case HCI_EVENT_CONNECTION_COMPLETE: 00501 // Connection management 00502 bt_flip_addr(addr, &packet[5]); 00503 log_info("Connection_complete (status=%u) %s\n", packet[2], bd_addr_to_str(addr)); 00504 conn = connection_for_address(addr); 00505 if (conn) { 00506 if (!packet[2]){ 00507 conn->state = OPEN; 00508 conn->con_handle = READ_BT_16(packet, 3); 00509 00510 // restart timer 00511 run_loop_set_timer(&conn->timeout, HCI_CONNECTION_TIMEOUT_MS); 00512 run_loop_add_timer(&conn->timeout); 00513 00514 log_info("New connection: handle %u, %s\n", conn->con_handle, bd_addr_to_str(conn->address)); 00515 00516 hci_emit_nr_connections_changed(); 00517 } else { 00518 // connection failed, remove entry 00519 linked_list_remove(&hci_stack.connections, (linked_item_t *) conn); 00520 btstack_memory_hci_connection_free( conn ); 00521 00522 // if authentication error, also delete link key 00523 if (packet[2] == 0x05) { 00524 hci_drop_link_key_for_bd_addr(&addr); 00525 } 00526 } 00527 } 00528 break; 00529 00530 case HCI_EVENT_LINK_KEY_REQUEST: 00531 log_info("HCI_EVENT_LINK_KEY_REQUEST\n"); 00532 hci_add_connection_flags_for_flipped_bd_addr(&packet[2], RECV_LINK_KEY_REQUEST); 00533 if (!hci_stack.remote_device_db) break; 00534 hci_add_connection_flags_for_flipped_bd_addr(&packet[2], HANDLE_LINK_KEY_REQUEST); 00535 hci_run(); 00536 // request handled by hci_run() as HANDLE_LINK_KEY_REQUEST gets set 00537 return; 00538 00539 case HCI_EVENT_LINK_KEY_NOTIFICATION: 00540 hci_add_connection_flags_for_flipped_bd_addr(&packet[2], RECV_LINK_KEY_NOTIFICATION); 00541 if (!hci_stack.remote_device_db) break; 00542 bt_flip_addr(addr, &packet[2]); 00543 hci_stack.remote_device_db->put_link_key(&addr, (link_key_t *) &packet[8]); 00544 // still forward event to allow dismiss of pairing dialog 00545 break; 00546 00547 case HCI_EVENT_PIN_CODE_REQUEST: 00548 hci_add_connection_flags_for_flipped_bd_addr(&packet[2], RECV_PIN_CODE_REQUEST); 00549 // PIN CODE REQUEST means the link key request didn't succee -> delete stored link key 00550 if (!hci_stack.remote_device_db) break; 00551 bt_flip_addr(addr, &packet[2]); 00552 hci_stack.remote_device_db->delete_link_key(&addr); 00553 break; 00554 00555 #ifndef EMBEDDED 00556 case HCI_EVENT_REMOTE_NAME_REQUEST_COMPLETE: 00557 if (!hci_stack.remote_device_db) break; 00558 if (packet[2]) break; // status not ok 00559 bt_flip_addr(addr, &packet[3]); 00560 // fix for invalid remote names - terminate on 0xff 00561 for (i=0; i<248;i++){ 00562 if (packet[9+i] == 0xff){ 00563 packet[9+i] = 0; 00564 break; 00565 } 00566 } 00567 memset(&device_name, 0, sizeof(device_name_t)); 00568 strncpy((char*) device_name, (char*) &packet[9], 248); 00569 hci_stack.remote_device_db->put_name(&addr, &device_name); 00570 break; 00571 00572 case HCI_EVENT_INQUIRY_RESULT: 00573 case HCI_EVENT_INQUIRY_RESULT_WITH_RSSI: 00574 if (!hci_stack.remote_device_db) break; 00575 // first send inq result packet 00576 hci_stack.packet_handler(HCI_EVENT_PACKET, packet, size); 00577 // then send cached remote names 00578 for (i=0; i<packet[2];i++){ 00579 bt_flip_addr(addr, &packet[3+i*6]); 00580 if (hci_stack.remote_device_db->get_name(&addr, &device_name)){ 00581 hci_emit_remote_name_cached(&addr, &device_name); 00582 } 00583 } 00584 return; 00585 #endif 00586 00587 case HCI_EVENT_DISCONNECTION_COMPLETE: 00588 if (!packet[2]){ 00589 handle = READ_BT_16(packet, 3); 00590 hci_connection_t * conn = connection_for_handle(handle); 00591 if (conn) { 00592 hci_shutdown_connection(conn); 00593 } 00594 } 00595 break; 00596 00597 case HCI_EVENT_HARDWARE_ERROR: 00598 if(hci_stack.control->hw_error){ 00599 (*hci_stack.control->hw_error)(); 00600 } 00601 break; 00602 00603 #ifdef HAVE_BLE 00604 case HCI_EVENT_LE_META: 00605 switch (packet[2]) { 00606 case HCI_SUBEVENT_LE_CONNECTION_COMPLETE: 00607 // Connection management 00608 bt_flip_addr(addr, &packet[8]); 00609 log_info("LE Connection_complete (status=%u) %s\n", packet[3], bd_addr_to_str(addr)); 00610 // LE connections are auto-accepted, so just create a connection if there isn't one already 00611 conn = connection_for_address(addr); 00612 if (packet[3]){ 00613 if (conn){ 00614 // outgoing connection failed, remove entry 00615 linked_list_remove(&hci_stack.connections, (linked_item_t *) conn); 00616 btstack_memory_hci_connection_free( conn ); 00617 00618 } 00619 // if authentication error, also delete link key 00620 if (packet[3] == 0x05) { 00621 hci_drop_link_key_for_bd_addr(&addr); 00622 } 00623 break; 00624 } 00625 if (!conn){ 00626 conn = create_connection_for_addr(addr); 00627 } 00628 if (!conn){ 00629 // no memory 00630 break; 00631 } 00632 00633 conn->state = OPEN; 00634 conn->con_handle = READ_BT_16(packet, 4); 00635 00636 // TODO: store - role, peer address type, conn_interval, conn_latency, supervision timeout, master clock 00637 00638 // restart timer 00639 // run_loop_set_timer(&conn->timeout, HCI_CONNECTION_TIMEOUT_MS); 00640 // run_loop_add_timer(&conn->timeout); 00641 00642 log_info("New connection: handle %u, %s\n", conn->con_handle, bd_addr_to_str(conn->address)); 00643 00644 hci_emit_nr_connections_changed(); 00645 break; 00646 00647 default: 00648 break; 00649 } 00650 break; 00651 #endif 00652 00653 default: 00654 break; 00655 } 00656 00657 // handle BT initialization 00658 if (hci_stack.state == HCI_STATE_INITIALIZING){ 00659 // handle H4 synchronization loss on restart 00660 // if (hci_stack.substate == 1 && packet[0] == HCI_EVENT_HARDWARE_ERROR){ 00661 // hci_stack.substate = 0; 00662 // } 00663 // handle normal init sequence 00664 if (hci_stack.substate % 2){ 00665 // odd: waiting for event 00666 if (packet[0] == HCI_EVENT_COMMAND_COMPLETE){ 00667 hci_stack.substate++; 00668 } 00669 } 00670 } 00671 00672 // help with BT sleep 00673 if (hci_stack.state == HCI_STATE_FALLING_ASLEEP 00674 && hci_stack.substate == 1 00675 && COMMAND_COMPLETE_EVENT(packet, hci_write_scan_enable)){ 00676 hci_stack.substate++; 00677 } 00678 00679 hci_stack.packet_handler(HCI_EVENT_PACKET, packet, size); 00680 00681 // execute main loop 00682 hci_run(); 00683 } 00684 00685 void packet_handler(uint8_t packet_type, uint8_t *packet, uint16_t size){ 00686 switch (packet_type) { 00687 case HCI_EVENT_PACKET: 00688 event_handler(packet, size); 00689 break; 00690 case HCI_ACL_DATA_PACKET: 00691 acl_handler(packet, size); 00692 break; 00693 default: 00694 break; 00695 } 00696 } 00697 00698 /** Register HCI packet handlers */ 00699 void hci_register_packet_handler(void (*handler)(uint8_t packet_type, uint8_t *packet, uint16_t size)){ 00700 hci_stack.packet_handler = handler; 00701 } 00702 00703 void hci_init(hci_transport_t *transport, void *config, bt_control_t *control, remote_device_db_t const* remote_device_db){ 00704 00705 // reference to use transport layer implementation 00706 hci_stack.hci_transport = transport; 00707 00708 // references to used control implementation 00709 hci_stack.control = control; 00710 00711 // reference to used config 00712 hci_stack.config = config; 00713 00714 // no connections yet 00715 hci_stack.connections = NULL; 00716 hci_stack.discoverable = 0; 00717 hci_stack.connectable = 0; 00718 00719 // no pending cmds 00720 hci_stack.decline_reason = 0; 00721 hci_stack.new_scan_enable_value = 0xff; 00722 00723 // higher level handler 00724 hci_stack.packet_handler = dummy_handler; 00725 00726 // store and open remote device db 00727 hci_stack.remote_device_db = remote_device_db; 00728 if (hci_stack.remote_device_db) { 00729 hci_stack.remote_device_db->open(); 00730 } 00731 00732 // max acl payload size defined in config.h 00733 hci_stack.acl_data_packet_length = HCI_ACL_PAYLOAD_SIZE; 00734 00735 // register packet handlers with transport 00736 transport->register_packet_handler(&packet_handler); 00737 00738 hci_stack.state = HCI_STATE_OFF; 00739 } 00740 00741 void hci_close(){ 00742 // close remote device db 00743 if (hci_stack.remote_device_db) { 00744 hci_stack.remote_device_db->close(); 00745 } 00746 while (hci_stack.connections) { 00747 hci_shutdown_connection((hci_connection_t *) hci_stack.connections); 00748 } 00749 hci_power_control(HCI_POWER_OFF); 00750 } 00751 00752 // State-Module-Driver overview 00753 // state module low-level 00754 // HCI_STATE_OFF off close 00755 // HCI_STATE_INITIALIZING, on open 00756 // HCI_STATE_WORKING, on open 00757 // HCI_STATE_HALTING, on open 00758 // HCI_STATE_SLEEPING, off/sleep close 00759 // HCI_STATE_FALLING_ASLEEP on open 00760 00761 static int hci_power_control_on(void){ 00762 00763 // power on 00764 int err = 0; 00765 if (hci_stack.control && hci_stack.control->on){ 00766 err = (*hci_stack.control->on)(hci_stack.config); 00767 } 00768 if (err){ 00769 log_error( "POWER_ON failed\n"); 00770 hci_emit_hci_open_failed(); 00771 return err; 00772 } 00773 00774 // open low-level device 00775 err = hci_stack.hci_transport->open(hci_stack.config); 00776 if (err){ 00777 log_error( "HCI_INIT failed, turning Bluetooth off again\n"); 00778 if (hci_stack.control && hci_stack.control->off){ 00779 (*hci_stack.control->off)(hci_stack.config); 00780 } 00781 hci_emit_hci_open_failed(); 00782 return err; 00783 } 00784 return 0; 00785 } 00786 00787 static void hci_power_control_off(void){ 00788 00789 log_info("hci_power_control_off\n"); 00790 00791 // close low-level device 00792 hci_stack.hci_transport->close(hci_stack.config); 00793 00794 log_info("hci_power_control_off - hci_transport closed\n"); 00795 00796 // power off 00797 if (hci_stack.control && hci_stack.control->off){ 00798 (*hci_stack.control->off)(hci_stack.config); 00799 } 00800 00801 log_info("hci_power_control_off - control closed\n"); 00802 00803 hci_stack.state = HCI_STATE_OFF; 00804 } 00805 00806 static void hci_power_control_sleep(void){ 00807 00808 log_info("hci_power_control_sleep\n"); 00809 00810 #if 0 00811 // don't close serial port during sleep 00812 00813 // close low-level device 00814 hci_stack.hci_transport->close(hci_stack.config); 00815 #endif 00816 00817 // sleep mode 00818 if (hci_stack.control && hci_stack.control->sleep){ 00819 (*hci_stack.control->sleep)(hci_stack.config); 00820 } 00821 00822 hci_stack.state = HCI_STATE_SLEEPING; 00823 } 00824 00825 static int hci_power_control_wake(void){ 00826 00827 log_info("hci_power_control_wake\n"); 00828 00829 // wake on 00830 if (hci_stack.control && hci_stack.control->wake){ 00831 (*hci_stack.control->wake)(hci_stack.config); 00832 } 00833 00834 #if 0 00835 // open low-level device 00836 int err = hci_stack.hci_transport->open(hci_stack.config); 00837 if (err){ 00838 log_error( "HCI_INIT failed, turning Bluetooth off again\n"); 00839 if (hci_stack.control && hci_stack.control->off){ 00840 (*hci_stack.control->off)(hci_stack.config); 00841 } 00842 hci_emit_hci_open_failed(); 00843 return err; 00844 } 00845 #endif 00846 00847 return 0; 00848 } 00849 00850 00851 int hci_power_control(HCI_POWER_MODE power_mode){ 00852 00853 log_info("hci_power_control: %u, current mode %u\n", power_mode, hci_stack.state); 00854 00855 int err = 0; 00856 switch (hci_stack.state){ 00857 00858 case HCI_STATE_OFF: 00859 switch (power_mode){ 00860 case HCI_POWER_ON: 00861 err = hci_power_control_on(); 00862 if (err) return err; 00863 // set up state machine 00864 hci_stack.num_cmd_packets = 1; // assume that one cmd can be sent 00865 hci_stack.state = HCI_STATE_INITIALIZING; 00866 hci_stack.substate = 0; 00867 break; 00868 case HCI_POWER_OFF: 00869 // do nothing 00870 break; 00871 case HCI_POWER_SLEEP: 00872 // do nothing (with SLEEP == OFF) 00873 break; 00874 } 00875 break; 00876 00877 case HCI_STATE_INITIALIZING: 00878 switch (power_mode){ 00879 case HCI_POWER_ON: 00880 // do nothing 00881 break; 00882 case HCI_POWER_OFF: 00883 // no connections yet, just turn it off 00884 hci_power_control_off(); 00885 break; 00886 case HCI_POWER_SLEEP: 00887 // no connections yet, just turn it off 00888 hci_power_control_sleep(); 00889 break; 00890 } 00891 break; 00892 00893 case HCI_STATE_WORKING: 00894 switch (power_mode){ 00895 case HCI_POWER_ON: 00896 // do nothing 00897 break; 00898 case HCI_POWER_OFF: 00899 // see hci_run 00900 hci_stack.state = HCI_STATE_HALTING; 00901 break; 00902 case HCI_POWER_SLEEP: 00903 // see hci_run 00904 hci_stack.state = HCI_STATE_FALLING_ASLEEP; 00905 hci_stack.substate = 0; 00906 break; 00907 } 00908 break; 00909 00910 case HCI_STATE_HALTING: 00911 switch (power_mode){ 00912 case HCI_POWER_ON: 00913 // set up state machine 00914 hci_stack.state = HCI_STATE_INITIALIZING; 00915 hci_stack.substate = 0; 00916 break; 00917 case HCI_POWER_OFF: 00918 // do nothing 00919 break; 00920 case HCI_POWER_SLEEP: 00921 // see hci_run 00922 hci_stack.state = HCI_STATE_FALLING_ASLEEP; 00923 hci_stack.substate = 0; 00924 break; 00925 } 00926 break; 00927 00928 case HCI_STATE_FALLING_ASLEEP: 00929 switch (power_mode){ 00930 case HCI_POWER_ON: 00931 00932 #if defined(USE_POWERMANAGEMENT) && defined(USE_BLUETOOL) 00933 // nothing to do, if H4 supports power management 00934 if (bt_control_iphone_power_management_enabled()){ 00935 hci_stack.state = HCI_STATE_INITIALIZING; 00936 hci_stack.substate = 6; 00937 break; 00938 } 00939 #endif 00940 // set up state machine 00941 hci_stack.num_cmd_packets = 1; // assume that one cmd can be sent 00942 hci_stack.state = HCI_STATE_INITIALIZING; 00943 hci_stack.substate = 0; 00944 break; 00945 case HCI_POWER_OFF: 00946 // see hci_run 00947 hci_stack.state = HCI_STATE_HALTING; 00948 break; 00949 case HCI_POWER_SLEEP: 00950 // do nothing 00951 break; 00952 } 00953 break; 00954 00955 case HCI_STATE_SLEEPING: 00956 switch (power_mode){ 00957 case HCI_POWER_ON: 00958 00959 #if defined(USE_POWERMANAGEMENT) && defined(USE_BLUETOOL) 00960 // nothing to do, if H4 supports power management 00961 if (bt_control_iphone_power_management_enabled()){ 00962 hci_stack.state = HCI_STATE_INITIALIZING; 00963 hci_stack.substate = 6; 00964 hci_update_scan_enable(); 00965 break; 00966 } 00967 #endif 00968 err = hci_power_control_wake(); 00969 if (err) return err; 00970 // set up state machine 00971 hci_stack.num_cmd_packets = 1; // assume that one cmd can be sent 00972 hci_stack.state = HCI_STATE_INITIALIZING; 00973 hci_stack.substate = 0; 00974 break; 00975 case HCI_POWER_OFF: 00976 hci_stack.state = HCI_STATE_HALTING; 00977 break; 00978 case HCI_POWER_SLEEP: 00979 // do nothing 00980 break; 00981 } 00982 break; 00983 } 00984 00985 // create internal event 00986 hci_emit_state(); 00987 00988 // trigger next/first action 00989 hci_run(); 00990 00991 return 0; 00992 } 00993 00994 static void hci_update_scan_enable(void){ 00995 // 2 = page scan, 1 = inq scan 00996 hci_stack.new_scan_enable_value = hci_stack.connectable << 1 | hci_stack.discoverable; 00997 hci_run(); 00998 } 00999 01000 void hci_discoverable_control(uint8_t enable){ 01001 if (enable) enable = 1; // normalize argument 01002 01003 if (hci_stack.discoverable == enable){ 01004 hci_emit_discoverable_enabled(hci_stack.discoverable); 01005 return; 01006 } 01007 01008 hci_stack.discoverable = enable; 01009 hci_update_scan_enable(); 01010 } 01011 01012 void hci_connectable_control(uint8_t enable){ 01013 if (enable) enable = 1; // normalize argument 01014 01015 // don't emit event 01016 if (hci_stack.connectable == enable) return; 01017 01018 hci_stack.connectable = enable; 01019 hci_update_scan_enable(); 01020 } 01021 01022 void hci_run(){ 01023 01024 hci_connection_t * connection; 01025 linked_item_t * it; 01026 01027 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01028 01029 // global/non-connection oriented commands 01030 01031 // decline incoming connections 01032 if (hci_stack.decline_reason){ 01033 uint8_t reason = hci_stack.decline_reason; 01034 hci_stack.decline_reason = 0; 01035 hci_send_cmd(&hci_reject_connection_request, hci_stack.decline_addr, reason); 01036 } 01037 01038 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01039 01040 // send scan enable 01041 if (hci_stack.new_scan_enable_value != 0xff){ 01042 hci_send_cmd(&hci_write_scan_enable, hci_stack.new_scan_enable_value); 01043 hci_stack.new_scan_enable_value = 0xff; 01044 } 01045 01046 // send pending HCI commands 01047 for (it = (linked_item_t *) hci_stack.connections; it ; it = it->next){ 01048 01049 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01050 01051 connection = (hci_connection_t *) it; 01052 01053 if (connection->state == RECEIVED_CONNECTION_REQUEST){ 01054 log_info("sending hci_accept_connection_request\n"); 01055 hci_send_cmd(&hci_accept_connection_request, connection->address, 1); 01056 connection->state = ACCEPTED_CONNECTION_REQUEST; 01057 } 01058 01059 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01060 01061 if (connection->authentication_flags & HANDLE_LINK_KEY_REQUEST){ 01062 link_key_t link_key; 01063 log_info("responding to link key request\n"); 01064 if (hci_stack.remote_device_db->get_link_key( &connection->address, &link_key)){ 01065 hci_send_cmd(&hci_link_key_request_reply, connection->address, &link_key); 01066 } else { 01067 hci_send_cmd(&hci_link_key_request_negative_reply, connection->address); 01068 } 01069 connectionClearAuthenticationFlags(connection, HANDLE_LINK_KEY_REQUEST); 01070 } 01071 } 01072 01073 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01074 01075 switch (hci_stack.state){ 01076 case HCI_STATE_INITIALIZING: 01077 // log_info("hci_init: substate %u\n", hci_stack.substate); 01078 if (hci_stack.substate % 2) { 01079 // odd: waiting for command completion 01080 return; 01081 } 01082 switch (hci_stack.substate >> 1){ 01083 case 0: // RESET 01084 hci_send_cmd(&hci_reset); 01085 if (hci_stack.config == 0 || ((hci_uart_config_t *)hci_stack.config)->baudrate_main == 0){ 01086 // skip baud change 01087 hci_stack.substate = 4; // >> 1 = 2 01088 } 01089 break; 01090 case 1: // SEND BAUD CHANGE 01091 hci_stack.control->baudrate_cmd(hci_stack.config, ((hci_uart_config_t *)hci_stack.config)->baudrate_main, hci_stack.hci_packet_buffer); 01092 hci_send_cmd_packet(hci_stack.hci_packet_buffer, 3 + hci_stack.hci_packet_buffer[2]); 01093 break; 01094 case 2: // LOCAL BAUD CHANGE 01095 hci_stack.hci_transport->set_baudrate(((hci_uart_config_t *)hci_stack.config)->baudrate_main); 01096 hci_stack.substate += 2; 01097 // break missing here for fall through 01098 01099 case 3: 01100 // custom initialization 01101 if (hci_stack.control && hci_stack.control->next_cmd){ 01102 int valid_cmd = (*hci_stack.control->next_cmd)(hci_stack.config, hci_stack.hci_packet_buffer); 01103 if (valid_cmd){ 01104 int size = 3 + hci_stack.hci_packet_buffer[2]; 01105 hci_stack.hci_transport->send_packet(HCI_COMMAND_DATA_PACKET, hci_stack.hci_packet_buffer, size); 01106 hci_stack.substate = 4; // more init commands 01107 break; 01108 } 01109 log_info("hci_run: init script done\n\r"); 01110 } 01111 // otherwise continue 01112 hci_send_cmd(&hci_read_bd_addr); 01113 break; 01114 case 4: 01115 hci_send_cmd(&hci_read_buffer_size); 01116 break; 01117 case 5: 01118 // ca. 15 sec 01119 hci_send_cmd(&hci_write_page_timeout, 0x6000); 01120 break; 01121 case 6: 01122 hci_send_cmd(&hci_write_scan_enable, (hci_stack.connectable << 1) | hci_stack.discoverable); // page scan 01123 break; 01124 case 7: 01125 #ifndef EMBEDDED 01126 { 01127 char hostname[30]; 01128 gethostname(hostname, 30); 01129 hostname[29] = '\0'; 01130 hci_send_cmd(&hci_write_local_name, hostname); 01131 break; 01132 } 01133 case 8: 01134 #ifdef USE_BLUETOOL 01135 hci_send_cmd(&hci_write_class_of_device, 0x007a020c); // Smartphone 01136 break; 01137 01138 case 9: 01139 #endif 01140 #endif 01141 // done. 01142 hci_stack.state = HCI_STATE_WORKING; 01143 hci_emit_state(); 01144 break; 01145 default: 01146 break; 01147 } 01148 hci_stack.substate++; 01149 break; 01150 01151 case HCI_STATE_HALTING: 01152 01153 log_info("HCI_STATE_HALTING\n"); 01154 // close all open connections 01155 connection = (hci_connection_t *) hci_stack.connections; 01156 if (connection){ 01157 01158 // send disconnect 01159 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01160 01161 log_info("HCI_STATE_HALTING, connection %p, handle %u\n", connection, (uint16_t)connection->con_handle); 01162 hci_send_cmd(&hci_disconnect, connection->con_handle, 0x13); // remote closed connection 01163 01164 // send disconnected event right away - causes higher layer connections to get closed, too. 01165 hci_shutdown_connection(connection); 01166 return; 01167 } 01168 log_info("HCI_STATE_HALTING, calling off\n"); 01169 01170 // switch mode 01171 hci_power_control_off(); 01172 01173 log_info("HCI_STATE_HALTING, emitting state\n"); 01174 hci_emit_state(); 01175 log_info("HCI_STATE_HALTING, done\n"); 01176 break; 01177 01178 case HCI_STATE_FALLING_ASLEEP: 01179 switch(hci_stack.substate) { 01180 case 0: 01181 log_info("HCI_STATE_FALLING_ASLEEP\n"); 01182 // close all open connections 01183 connection = (hci_connection_t *) hci_stack.connections; 01184 01185 #if defined(USE_POWERMANAGEMENT) && defined(USE_BLUETOOL) 01186 // don't close connections, if H4 supports power management 01187 if (bt_control_iphone_power_management_enabled()){ 01188 connection = NULL; 01189 } 01190 #endif 01191 if (connection){ 01192 01193 // send disconnect 01194 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01195 01196 log_info("HCI_STATE_FALLING_ASLEEP, connection %p, handle %u\n", connection, (uint16_t)connection->con_handle); 01197 hci_send_cmd(&hci_disconnect, connection->con_handle, 0x13); // remote closed connection 01198 01199 // send disconnected event right away - causes higher layer connections to get closed, too. 01200 hci_shutdown_connection(connection); 01201 return; 01202 } 01203 01204 // disable page and inquiry scan 01205 if (!hci_can_send_packet_now(HCI_COMMAND_DATA_PACKET)) return; 01206 01207 log_info("HCI_STATE_HALTING, disabling inq cans\n"); 01208 hci_send_cmd(&hci_write_scan_enable, hci_stack.connectable << 1); // drop inquiry scan but keep page scan 01209 01210 // continue in next sub state 01211 hci_stack.substate++; 01212 break; 01213 case 1: 01214 // wait for command complete "hci_write_scan_enable" in event_handler(); 01215 break; 01216 case 2: 01217 log_info("HCI_STATE_HALTING, calling sleep\n"); 01218 #if defined(USE_POWERMANAGEMENT) && defined(USE_BLUETOOL) 01219 // don't actually go to sleep, if H4 supports power management 01220 if (bt_control_iphone_power_management_enabled()){ 01221 // SLEEP MODE reached 01222 hci_stack.state = HCI_STATE_SLEEPING; 01223 hci_emit_state(); 01224 break; 01225 } 01226 #endif 01227 // switch mode 01228 hci_power_control_sleep(); // changes hci_stack.state to SLEEP 01229 hci_emit_state(); 01230 break; 01231 01232 default: 01233 break; 01234 } 01235 break; 01236 01237 default: 01238 break; 01239 } 01240 } 01241 01242 int hci_send_cmd_packet(uint8_t *packet, int size){ 01243 bd_addr_t addr; 01244 hci_connection_t * conn; 01245 // house-keeping 01246 01247 // create_connection? 01248 if (IS_COMMAND(packet, hci_create_connection)){ 01249 bt_flip_addr(addr, &packet[3]); 01250 log_info("Create_connection to %s\n", bd_addr_to_str(addr)); 01251 conn = connection_for_address(addr); 01252 if (conn) { 01253 // if connection exists 01254 if (conn->state == OPEN) { 01255 // and OPEN, emit connection complete command 01256 hci_emit_connection_complete(conn, 0); 01257 } 01258 // otherwise, just ignore as it is already in the open process 01259 return 0; // don't sent packet to controller 01260 01261 } 01262 // create connection struct and register, state = SENT_CREATE_CONNECTION 01263 conn = create_connection_for_addr(addr); 01264 if (!conn){ 01265 // notify client that alloc failed 01266 hci_emit_connection_complete(conn, BTSTACK_MEMORY_ALLOC_FAILED); 01267 return 0; // don't sent packet to controller 01268 } 01269 conn->state = SENT_CREATE_CONNECTION; 01270 } 01271 01272 if (IS_COMMAND(packet, hci_link_key_request_reply)){ 01273 hci_add_connection_flags_for_flipped_bd_addr(&packet[3], SENT_LINK_KEY_REPLY); 01274 } 01275 if (IS_COMMAND(packet, hci_link_key_request_negative_reply)){ 01276 hci_add_connection_flags_for_flipped_bd_addr(&packet[3], SENT_LINK_KEY_NEGATIVE_REQUEST); 01277 } 01278 if (IS_COMMAND(packet, hci_pin_code_request_reply)){ 01279 hci_add_connection_flags_for_flipped_bd_addr(&packet[3], SENT_PIN_CODE_REPLY); 01280 } 01281 if (IS_COMMAND(packet, hci_pin_code_request_negative_reply)){ 01282 hci_add_connection_flags_for_flipped_bd_addr(&packet[3], SENT_PIN_CODE_NEGATIVE_REPLY); 01283 } 01284 01285 if (IS_COMMAND(packet, hci_delete_stored_link_key)){ 01286 if (hci_stack.remote_device_db){ 01287 bt_flip_addr(addr, &packet[3]); 01288 hci_stack.remote_device_db->delete_link_key(&addr); 01289 } 01290 } 01291 01292 hci_stack.num_cmd_packets--; 01293 return hci_stack.hci_transport->send_packet(HCI_COMMAND_DATA_PACKET, packet, size); 01294 } 01295 01296 /** 01297 * pre: numcmds >= 0 - it's allowed to send a command to the controller 01298 */ 01299 int hci_send_cmd(const hci_cmd_t *cmd, ...){ 01300 va_list argptr; 01301 va_start(argptr, cmd); 01302 uint16_t size = hci_create_cmd_internal(hci_stack.hci_packet_buffer, cmd, argptr); 01303 va_end(argptr); 01304 return hci_send_cmd_packet(hci_stack.hci_packet_buffer, size); 01305 } 01306 01307 // Create various non-HCI events. 01308 // TODO: generalize, use table similar to hci_create_command 01309 01310 void hci_emit_state(){ 01311 uint8_t event[3]; 01312 event[0] = BTSTACK_EVENT_STATE; 01313 event[1] = sizeof(event) - 2; 01314 event[2] = hci_stack.state; 01315 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01316 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01317 } 01318 01319 void hci_emit_connection_complete(hci_connection_t *conn, uint8_t status){ 01320 uint8_t event[13]; 01321 event[0] = HCI_EVENT_CONNECTION_COMPLETE; 01322 event[1] = sizeof(event) - 2; 01323 event[2] = status; 01324 bt_store_16(event, 3, conn->con_handle); 01325 bt_flip_addr(&event[5], conn->address); 01326 event[11] = 1; // ACL connection 01327 event[12] = 0; // encryption disabled 01328 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01329 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01330 } 01331 01332 void hci_emit_disconnection_complete(uint16_t handle, uint8_t reason){ 01333 uint8_t event[6]; 01334 event[0] = HCI_EVENT_DISCONNECTION_COMPLETE; 01335 event[1] = sizeof(event) - 2; 01336 event[2] = 0; // status = OK 01337 bt_store_16(event, 3, handle); 01338 event[5] = reason; 01339 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01340 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01341 } 01342 01343 void hci_emit_l2cap_check_timeout(hci_connection_t *conn){ 01344 uint8_t event[4]; 01345 event[0] = L2CAP_EVENT_TIMEOUT_CHECK; 01346 event[1] = sizeof(event) - 2; 01347 bt_store_16(event, 2, conn->con_handle); 01348 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01349 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01350 } 01351 01352 void hci_emit_nr_connections_changed(){ 01353 uint8_t event[3]; 01354 event[0] = BTSTACK_EVENT_NR_CONNECTIONS_CHANGED; 01355 event[1] = sizeof(event) - 2; 01356 event[2] = nr_hci_connections(); 01357 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01358 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01359 } 01360 01361 void hci_emit_hci_open_failed(){ 01362 uint8_t event[2]; 01363 event[0] = BTSTACK_EVENT_POWERON_FAILED; 01364 event[1] = sizeof(event) - 2; 01365 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01366 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01367 } 01368 01369 #ifndef EMBEDDED 01370 void hci_emit_btstack_version() { 01371 uint8_t event[6]; 01372 event[0] = BTSTACK_EVENT_VERSION; 01373 event[1] = sizeof(event) - 2; 01374 event[2] = BTSTACK_MAJOR; 01375 event[3] = BTSTACK_MINOR; 01376 bt_store_16(event, 4, BTSTACK_REVISION); 01377 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01378 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01379 } 01380 #endif 01381 01382 void hci_emit_system_bluetooth_enabled(uint8_t enabled){ 01383 uint8_t event[3]; 01384 event[0] = BTSTACK_EVENT_SYSTEM_BLUETOOTH_ENABLED; 01385 event[1] = sizeof(event) - 2; 01386 event[2] = enabled; 01387 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01388 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01389 } 01390 01391 void hci_emit_remote_name_cached(bd_addr_t *addr, device_name_t *name){ 01392 uint8_t event[2+1+6+248]; 01393 event[0] = BTSTACK_EVENT_REMOTE_NAME_CACHED; 01394 event[1] = sizeof(event) - 2; 01395 event[2] = 0; // just to be compatible with HCI_EVENT_REMOTE_NAME_REQUEST_COMPLETE 01396 bt_flip_addr(&event[3], *addr); 01397 memcpy(&event[9], name, 248); 01398 hci_dump_packet(HCI_EVENT_PACKET, 0, event, sizeof(event)); 01399 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01400 } 01401 01402 void hci_emit_discoverable_enabled(uint8_t enabled){ 01403 uint8_t event[3]; 01404 event[0] = BTSTACK_EVENT_DISCOVERABLE_ENABLED; 01405 event[1] = sizeof(event) - 2; 01406 event[2] = enabled; 01407 hci_dump_packet( HCI_EVENT_PACKET, 0, event, sizeof(event)); 01408 hci_stack.packet_handler(HCI_EVENT_PACKET, event, sizeof(event)); 01409 }
Generated on Tue Jul 12 2022 15:55:29 by
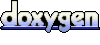