
BTstack Bluetooth stack
Embed:
(wiki syntax)
Show/hide line numbers
bd_addr.cpp
00001 #include "mbed.h" 00002 #include "bd_addr.h" 00003 bd_addr::bd_addr() 00004 { 00005 00006 } 00007 00008 bd_addr::bd_addr(char* s) 00009 { 00010 for(int i = 0; i <= 5; i++) { 00011 ad[i] = strtol(s+i*3, NULL, 16); 00012 } 00013 } 00014 00015 uint8_t* bd_addr::data(uint8_t* addr) 00016 { 00017 if (addr != NULL) { 00018 ad[5] = addr[0]; 00019 ad[4] = addr[1]; 00020 ad[3] = addr[2]; 00021 ad[2] = addr[3]; 00022 ad[1] = addr[4]; 00023 ad[0] = addr[5]; 00024 } 00025 return ad; 00026 } 00027 00028 char* bd_addr::to_str() 00029 { 00030 snprintf(ad_str, sizeof(ad_str), "%02X:%02X:%02X:%02X:%02X:%02X", 00031 ad[0], ad[1], ad[2], ad[3], ad[4], ad[5]); 00032 return ad_str; 00033 }
Generated on Tue Jul 12 2022 15:55:29 by
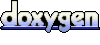