
BTstack Bluetooth stack
Embed:
(wiki syntax)
Show/hide line numbers
USBHostBTstack.h
00001 #include "USBHostConf.h" 00002 #include "USBHost.h" 00003 #pragma once 00004 00005 #define TEST_ASSERT(A) while(!(A)){fprintf(stderr,"\n\n%s@%d %s ASSERT!\n\n",__PRETTY_FUNCTION__,__LINE__,#A);exit(1);}; 00006 00007 struct Packet { 00008 uint8_t type; 00009 uint8_t* buf; 00010 uint16_t len; 00011 }; 00012 00013 /** 00014 * A class to communicate a BTstack 00015 */ 00016 class USBHostBTstack : public IUSBEnumerator { 00017 public: 00018 /** 00019 * Constructor 00020 * 00021 */ 00022 USBHostBTstack(); 00023 00024 /** 00025 * Check if a BTstack device is connected 00026 * 00027 * @return true if a BTstack device is connected 00028 */ 00029 bool connected(); 00030 00031 /** 00032 * Try to connect to a BTstack device 00033 * 00034 * @return true if connection was successful 00035 */ 00036 bool connect(); 00037 00038 int open(); 00039 int send_packet(uint8_t packet_type, uint8_t* packet, int size); 00040 void register_packet_handler( void (*pMethod)(uint8_t, uint8_t*, uint16_t)); 00041 void poll(); 00042 00043 protected: 00044 //From IUSBEnumerator 00045 virtual void setVidPid(uint16_t vid, uint16_t pid); 00046 virtual bool parseInterface(uint8_t intf_nb, uint8_t intf_class, uint8_t intf_subclass, uint8_t intf_protocol); //Must return true if the interface should be parsed 00047 virtual bool useEndpoint(uint8_t intf_nb, ENDPOINT_TYPE type, ENDPOINT_DIRECTION dir); //Must return true if the endpoint will be used 00048 00049 private: 00050 USBHost * host; 00051 USBDeviceConnected * dev; 00052 bool dev_connected; 00053 uint8_t int_report[64]; 00054 uint8_t bulk_report[64]; 00055 USBEndpoint * int_in; 00056 USBEndpoint * bulk_in; 00057 USBEndpoint * bulk_out; 00058 bool ep_int_in; 00059 bool ep_bulk_in; 00060 bool ep_bulk_out; 00061 00062 bool btstack_device_found; 00063 int btstack_intf; 00064 void (*m_pCb)(uint8_t, uint8_t*, uint16_t); 00065 Mail<Packet, 2> mail_box; 00066 void int_rxHandler(); 00067 void bulk_rxHandler(); 00068 void init(); 00069 }; 00070 00071 void _debug_bytes(const char* pretty, int line, const char* s, uint8_t* buf, int len);
Generated on Tue Jul 12 2022 15:55:29 by
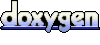