Project Autus - Automated Plant Chamber
Fork of keypad_test by
Embed:
(wiki syntax)
Show/hide line numbers
OneWireThermometer.h
00001 /* 00002 * OneWireThermometer. Base class for Maxim One-Wire Thermometers. 00003 * Uses the OneWireCRC library. 00004 * 00005 * Copyright (C) <2010> Petras Saduikis <petras@petras.co.uk> 00006 * 00007 * This file is part of OneWireThermometer. 00008 * 00009 * OneWireThermometer is free software: you can redistribute it and/or modify 00010 * it under the terms of the GNU General Public License as published by 00011 * the Free Software Foundation, either version 3 of the License, or 00012 * (at your option) any later version. 00013 * 00014 * OneWireThermometer is distributed in the hope that it will be useful, 00015 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00016 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00017 * GNU General Public License for more details. 00018 * 00019 * You should have received a copy of the GNU General Public License 00020 * along with OneWireThermometer. If not, see <http://www.gnu.org/licenses/>. 00021 */ 00022 00023 #ifndef SNATCH59_ONEWIRETHERMOMETER_H 00024 #define SNATCH59_ONEWIRETHERMOMETER_H 00025 00026 #include <mbed.h> 00027 #include "OneWireCRC.h" 00028 #include "OneWireDefs.h" 00029 00030 typedef unsigned char BYTE; // something a byte wide 00031 typedef unsigned char DEVADDRESS[8]; // stores the address of one device 00032 00033 class OneWireThermometer 00034 { 00035 public: 00036 OneWireThermometer(PinName pin, int device_id); 00037 00038 bool initialize(); 00039 float readTemperature(int device); 00040 virtual void setResolution(eResolution resln) = 0; 00041 int getDeviceCount(); 00042 00043 protected: 00044 OneWireCRC oneWire; 00045 const int deviceId; 00046 00047 eResolution resolution; 00048 BYTE address[8]; 00049 DEVADDRESS devices[10]; 00050 int deviceCount; 00051 00052 void resetAndAddress(); 00053 bool readAndValidateData(BYTE* data); 00054 virtual float calculateTemperature(BYTE* data) = 0; // device specific 00055 }; 00056 00057 #endif
Generated on Tue Jul 12 2022 21:16:07 by
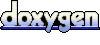