Fork of NetServicesMin with some warnings removed
Fork of NetServicesMin by
DNSRequest.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** \file 00025 DNS Request header file 00026 */ 00027 00028 #ifndef DNSREQUEST_H 00029 #define DNSREQUEST_H 00030 00031 #include "core/net.h" 00032 #include "core/ipaddr.h" 00033 #include "core/host.h" 00034 //Essentially it is a safe interface to NetDnsRequest 00035 #include "if/net/netdnsrequest.h" 00036 00037 ///DNS Request error codes 00038 enum DNSRequestErr 00039 { 00040 __DNS_MIN = -0xFFFF, 00041 DNS_SETUP, ///<DNSRequest not properly configured 00042 DNS_IF, ///<Interface has problems, does not exist or is not initialized 00043 DNS_MEM, ///<Not enough mem 00044 DNS_INUSE, ///<Interface / Port is in use 00045 DNS_PROCESSING, ///<Request has not completed 00046 //... 00047 DNS_OK = 0 ///<Success 00048 }; 00049 00050 ///DNS Request Result Events 00051 enum DNSReply 00052 { 00053 DNS_PRTCL, 00054 DNS_NOTFOUND, ///Hostname is unknown 00055 DNS_ERROR, ///Problem with DNS Service 00056 //... 00057 DNS_FOUND, 00058 }; 00059 00060 //class NetDnsRequest; 00061 //enum NetDnsReply; 00062 00063 ///This is a simple DNS Request class 00064 /** 00065 This class exposes an API to deal with DNS Requests 00066 */ 00067 class DNSRequest 00068 { 00069 public: 00070 ///Creates a new request 00071 DNSRequest(); 00072 00073 ///Terminates and closes request 00074 ~DNSRequest(); 00075 00076 ///Resolves an hostname 00077 /** 00078 @param hostname : hostname to resolve 00079 */ 00080 DNSRequestErr resolve(const char* hostname); 00081 00082 ///Resolves an hostname 00083 /** 00084 @param host : hostname to resolve, the result will be stored in the IpAddr field of this object 00085 */ 00086 DNSRequestErr resolve(Host* pHost); 00087 00088 ///Setups callback 00089 /** 00090 The callback function will be called on result. 00091 @param pMethod : callback function 00092 */ 00093 void setOnReply( void (*pMethod)(DNSReply) ); 00094 00095 class CDummy; 00096 ///Setups callback 00097 /** 00098 The callback function will be called on result. 00099 @param pItem : instance of class on which to execute the callback method 00100 @param pMethod : callback method 00101 */ 00102 template<class T> 00103 void setOnReply( T* pItem, void (T::*pMethod)(DNSReply) ) 00104 { 00105 m_pCbItem = (CDummy*) pItem; 00106 m_pCbMeth = (void (CDummy::*)(DNSReply)) pMethod; 00107 } 00108 00109 ///Gets IP address once it has been resolved 00110 /** 00111 @param pIp : pointer to an IpAddr instance in which to store the resolved IP address 00112 */ 00113 DNSRequestErr getResult(IpAddr* pIp); 00114 00115 ///Closes DNS Request before completion 00116 DNSRequestErr close(); 00117 00118 protected: 00119 void onNetDnsReply(NetDnsReply r); 00120 DNSRequestErr checkInst(); 00121 00122 private: 00123 NetDnsRequest* m_pNetDnsRequest; 00124 00125 CDummy* m_pCbItem; 00126 void (CDummy::*m_pCbMeth)(DNSReply); 00127 00128 void (*m_pCb)(DNSReply); 00129 00130 }; 00131 00132 #endif
Generated on Wed Jul 13 2022 04:33:03 by
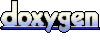