
Arianna autonomous DAQ firmware
Dependencies: mbed SDFileSystemFilinfo AriSnProtocol NetServicesMin AriSnComm MODSERIAL PowerControlClkPatch DS1820OW
SnStatusFrame.h
00001 #ifndef SN_SnStatusFrame 00002 #define SN_SnStatusFrame 00003 00004 #include <stdint.h> 00005 00006 #include "SnBitUtils.h" 00007 #include "SnCommWin.h" 00008 #include "SnConfigFrame.h" 00009 #include "SnEventFrame.h" 00010 #include "SnTempFrame.h" 00011 #include "SnSDUtils.h" 00012 #include "Watchdog.h" 00013 00014 //#define ST_DEBUG 00015 00016 struct SnStatusFrame { 00017 // 00018 // A simple struct (everything public) to function like namespace. 00019 // The contents of the status frame are sent from here, in order to 00020 // help make sure the status frame is the same for each comm method. 00021 // 00022 // No member variables are used in order to preserve memory on the mbed. 00023 // (i.e. no actual status frame middle-man object exists) 00024 // 00025 00026 static const uint8_t kIOVers; // MUST BE INCREASED if bytes written/read change!! 00027 00028 static const uint32_t kMaxSizeOfV1 = 00029 1u + sizeof(uint64_t) 00030 + (sizeof(uint32_t)*3u) + (sizeof(uint16_t)) 00031 + (sizeof(uint8_t)*3u) + SnConfigFrame::kConfLblLen 00032 + SnEventFrame::kMaxSizeOfV1; 00033 static const uint32_t kMaxSizeOfV2 = 00034 sizeof(uint64_t) + (3u*sizeof(uint32_t)) + (2u*sizeof(uint16_t)) 00035 + (3u*sizeof(uint8_t)) + (2u*sizeof(float)) 00036 + SnConfigFrame::kConfLblLen; 00037 static const uint32_t kMaxSizeOfV3 = 00038 kMaxSizeOfV2 + sizeof(uint32_t) + sizeof(float); 00039 static const uint32_t kMaxSizeOfV4 = kMaxSizeOfV3 - sizeof(uint32_t); 00040 static const uint32_t kMaxSizeOfV5 = kMaxSizeOfV4 + sizeof(uint8_t); 00041 static const uint32_t kMaxSizeOfV6 = kMaxSizeOfV5 + sizeof(uint8_t) + sizeof(uint32_t); // iov and power on time 00042 static const uint32_t kMaxSizeOfV7 = kMaxSizeOfV6 + sizeof(float); // temperature 00043 static const uint32_t kMaxSizeOfV8 = kMaxSizeOfV7 + sizeof(uint8_t); 00044 static const uint32_t kMaxSizeOfV9 = kMaxSizeOfV8; // V9 just marks that no event is automatically sent along 00045 static const uint32_t kMaxSizeOfV10 = kMaxSizeOfV9 00046 - (2u*sizeof(float)) // remove thmrate, evtrate 00047 + (2u*sizeof(uint32_t)) // add num thm trigs, num saved evts 00048 + sizeof(float); // add seq livetime 00049 static const uint32_t kMaxSizeOf = kMaxSizeOfV1; 00050 00051 00052 public: 00053 static bool fgRecalcFiles; 00054 private: 00055 static float fgFreeMB; 00056 00057 public: 00058 static 00059 float GetFreeMB() { return fgFreeMB; } 00060 00061 static 00062 uint32_t GetMaxSizeOf(const uint8_t rv) { 00063 if (rv==1) { 00064 return kMaxSizeOfV1; 00065 } else if (rv==2) { 00066 return kMaxSizeOfV2; 00067 } else if (rv==3) { 00068 return kMaxSizeOfV3; 00069 } else if (rv==4) { 00070 return kMaxSizeOfV4; 00071 } else if (rv==5) { 00072 return kMaxSizeOfV5; 00073 } else if (rv==6) { 00074 return kMaxSizeOfV6; 00075 } else if (rv==7) { 00076 return kMaxSizeOfV7; 00077 } else if (rv==8) { 00078 return kMaxSizeOfV8; 00079 } else if (rv==9) { 00080 return kMaxSizeOfV9; 00081 } else if (rv==10) { 00082 return kMaxSizeOfV10; 00083 } else { 00084 return kMaxSizeOf; 00085 } 00086 } 00087 00088 template<class T> 00089 static 00090 SnCommWin::ECommWinResult WriteTo(T& x, 00091 const SnConfigFrame& conf, 00092 const uint16_t seq, 00093 const uint32_t numThmTrigs, 00094 const uint32_t numSavedEvts, 00095 const float seqlive, 00096 const uint32_t powerOnTime, 00097 const SnTempFrame& temper, 00098 const uint8_t loseLSB, 00099 const uint8_t loseMSB, 00100 const uint16_t wvBase) { 00101 // expect 'x' to be a MODSERIAL& or a char const* 00102 00103 const uint32_t llen = strlen(conf.GetLabel()); 00104 00105 // if anything about these writes changes, update kIOVers and SizeOf 00106 x = SnBitUtils::WriteTo(x, SnStatusFrame::kIOVers); 00107 x = SnBitUtils::WriteTo(x, conf.GetMacAddress()); 00108 x = SnBitUtils::WriteTo(x, llen); 00109 x = SnBitUtils::WriteTo(x, conf.GetLabel(), llen); 00110 x = SnBitUtils::WriteTo(x, conf.GetRun()); 00111 x = SnBitUtils::WriteTo(x, seq); 00112 x = SnBitUtils::WriteTo(x, static_cast<uint32_t>(time(0))); 00113 x = SnBitUtils::WriteTo(x, loseLSB); 00114 x = SnBitUtils::WriteTo(x, loseMSB); 00115 x = SnBitUtils::WriteTo(x, wvBase); 00116 // x = SnBitUtils::WriteTo(x, thmrate); 00117 // x = SnBitUtils::WriteTo(x, evtrate); 00118 // file info 00119 if (fgRecalcFiles) { 00120 /* 00121 #ifdef ST_DEBUG 00122 printf("Calling GetDirProps for %s\r\n",SnSDUtils::kSDdir); 00123 #endif 00124 SnSDUtils::GetDirProps(SnSDUtils::kSDdir, fgNfiles, fgTotKbytes); 00125 fgTotKbytes /= 1e3; // KB 00126 #ifdef ST_DEBUG 00127 printf("nfiles=%u, tb=%g kb\r\n",fgNfiles,fgTotKbytes); 00128 #endif 00129 */ 00130 fgFreeMB = SnSDUtils::GetFreeBytes() / 1048576.0; 00131 #ifdef ST_DEBUG 00132 printf("fgFreeMB = %g\r\n",fgFreeMB); 00133 #endif 00134 fgRecalcFiles = false; 00135 } 00136 x = SnBitUtils::WriteTo(x, fgFreeMB); 00137 00138 // watchdog reset bit cleared after status received successfully, not here 00139 const uint8_t wdreset = Watchdog::didWatchdogReset(); 00140 x = SnBitUtils::WriteTo(x, wdreset); 00141 00142 // the config i/o version we expect (might be needed to send a new config to the station) 00143 x = SnBitUtils::WriteTo(x, conf.kIOVers); 00144 00145 x = SnBitUtils::WriteTo(x, powerOnTime); 00146 x = SnBitUtils::WriteTo(x, temper.GetTemperature()); 00147 x = SnBitUtils::WriteTo(x, static_cast<uint8_t>(SnSDUtils::IsInitOk())); 00148 00149 // send number of thm trigs, num saved events and livetime 00150 x = SnBitUtils::WriteTo(x, numThmTrigs); 00151 x = SnBitUtils::WriteTo(x, numSavedEvts); 00152 x = SnBitUtils::WriteTo(x, seqlive); 00153 00154 return SnCommWin::kOkMsgSent; 00155 } 00156 00157 static 00158 uint32_t SizeOf(const uint8_t rv, const uint32_t confLblLen, 00159 const uint8_t loseLSB, const uint8_t loseMSB) { 00160 // number of bytes read/written during i/o 00161 const uint32_t maxsize = GetMaxSizeOf(rv); 00162 const uint32_t msz = maxsize - SnConfigFrame::kConfLblLen 00163 + confLblLen; 00164 if (rv==1) { 00165 if ((loseLSB==0) && (loseMSB==0)) { 00166 return msz; 00167 } else { 00168 return msz - maxsize 00169 + SnEventFrame::SizeOf(SnEventFrame::kIOVers, 00170 loseLSB, loseMSB); 00171 } 00172 } else { 00173 return msz; 00174 } 00175 } 00176 00177 static 00178 uint32_t SizeOf(const uint8_t rv, const SnConfigFrame& conf) { 00179 return SizeOf(rv, conf.GetLabelStrLen(), 00180 conf.GetWvLoseLSB(), conf.GetWvLoseMSB()); 00181 } 00182 00183 }; 00184 00185 #endif // SN_SnStatusFrame
Generated on Thu Jul 14 2022 21:01:57 by
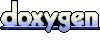