_Very_ simple wrapper to a WiFly module connected via serial port.
Embed:
(wiki syntax)
Show/hide line numbers
wifly.cpp
00001 // 00002 // Wrapper for the WiFly module, using the serial port 00003 // 00004 // Copyright 2010 Pulse-Robotics, Inc. 00005 // John Sosoka, Tyler Wilson 00006 // 00007 00008 #include "wifly.h" 00009 00010 00011 WiFly::WiFly(PinName tx, PinName rx) 00012 : port(0) 00013 { 00014 port = new Serial(tx, rx); 00015 00016 if (port) 00017 { 00018 port->baud(9600); 00019 } 00020 } 00021 00022 void WiFly::commandMode() const 00023 { 00024 if (port) 00025 { 00026 port->printf("$$$"); 00027 // wait for 250ms 00028 00029 char result[4]; 00030 port->scanf("%c%c%c", result[0], result[1], result[2]); 00031 result[3] = 0; 00032 00033 if (strcmp(result, "CMD") != 0) 00034 { 00035 // not the expected reply 00036 } 00037 } 00038 } 00039 00040 void WiFly::dataMode() const 00041 { 00042 if (port) 00043 { 00044 port->printf("exit\r"); 00045 00046 char result[5]; 00047 port->scanf("%c%c%c%c", result[0], result[1], result[2], result[3]); 00048 result[4] = 0; 00049 00050 if (strcmp(result, "EXIT") != 0) 00051 { 00052 // not the expected reply 00053 } 00054 } 00055 } 00056 00057 00058 // Serial methods 00059 void WiFly::baud(int baudrate) 00060 { 00061 port->baud(baudrate); 00062 } 00063 00064 int WiFly::getc() 00065 { 00066 return port->getc(); 00067 } 00068 00069 void WiFly::putc(int c) 00070 { 00071 port->putc(c); 00072 } 00073 00074 int WiFly::readable() 00075 { 00076 return port->readable(); 00077 } 00078
Generated on Sun Jul 17 2022 22:34:36 by
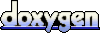