
ICRS Eurobot 2013
Dependencies: mbed mbed-rtos Servo QEI
coprocserial.cpp
00001 #include "Kalman.h" 00002 #include "mbed.h" 00003 #include "globals.h" 00004 00005 Serial coprocserial(NC, P_SERIAL_RX); 00006 00007 //DigitalOut OLED1(LED1); 00008 DigitalOut OLED2(LED2); 00009 00010 // bytes packing 00011 typedef union { 00012 00013 struct _data{ 00014 unsigned char sync[3]; 00015 unsigned char ID; 00016 float value; 00017 float variance; 00018 } data; 00019 00020 unsigned char type_char[12]; 00021 } bytepack_t; 00022 00023 bytepack_t incbuff; 00024 00025 volatile int buffprintflag = 0; 00026 void printbuff(){ 00027 while(!buffprintflag); 00028 buffprintflag = 0; 00029 for(int i = 0; i < 9; i++){ 00030 printf("%x ", incbuff.type_char[i]); 00031 } 00032 printf("\r\n"); 00033 } 00034 00035 void procserial(){ 00036 00037 //Fetch the byte in a "clear interrupt" sense 00038 unsigned char c = LPC_UART1->RBR; 00039 00040 //OLED1 = !OLED1; 00041 00042 static int ctr = 0; 00043 00044 00045 if (ctr < 3){ 00046 if (c == 0xFF) 00047 ctr++; 00048 else 00049 ctr = 0; 00050 } else { 00051 incbuff.type_char[ctr] = c; 00052 if (++ctr == 12){ 00053 ctr = 0; 00054 00055 OLED2 = !OLED2; 00056 buffprintflag = 1; 00057 00058 //runupdate 00059 Kalman::runupdate((Kalman::measurement_t)incbuff.data.ID, incbuff.data.value, incbuff.data.variance); 00060 } 00061 } 00062 00063 } 00064 00065 void InitSerial(){ 00066 00067 coprocserial.baud(115200); 00068 00069 printf("attachserial\r\n"); 00070 coprocserial.attach(procserial); 00071 }
Generated on Wed Jul 13 2022 18:28:36 by
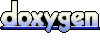