
ICRS Eurobot 2013
Dependencies: mbed mbed-rtos Servo QEI
Extremum.h
00001 /* 00002 * Tiny Vector Matrix Library 00003 * Dense Vector Matrix Libary of Tiny size using Expression Templates 00004 * 00005 * Copyright (C) 2001 - 2007 Olaf Petzold <opetzold@users.sourceforge.net> 00006 * 00007 * This library is free software; you can redistribute it and/or 00008 * modify it under the terms of the GNU Lesser General Public 00009 * License as published by the Free Software Foundation; either 00010 * version 2.1 of the License, or (at your option) any later version. 00011 * 00012 * This library is distributed in the hope that it will be useful, 00013 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00014 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00015 * Lesser General Public License for more details. 00016 * 00017 * You should have received a copy of the GNU Lesser General Public 00018 * License along with this library; if not, write to the Free Software 00019 * Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA 00020 * 00021 * $Id: Extremum.h,v 1.10 2007-06-23 15:58:58 opetzold Exp $ 00022 */ 00023 00024 #ifndef TVMET_EXTREMUM_H 00025 #define TVMET_EXTREMUM_H 00026 00027 namespace tvmet { 00028 00029 00030 /** 00031 * \class matrix_tag Extremum.h "tvmet/Extremum.h" 00032 * \brief For use with Extremum to simplify max handling. 00033 * This allows the min/max functions to return an Extremum object. 00034 */ 00035 struct matrix_tag { }; 00036 00037 00038 /** 00039 * \class vector_tag Extremum.h "tvmet/Extremum.h" 00040 * \brief For use with Extremum to simplify max handling. 00041 * This allows the min/max functions to return an Extremum object. 00042 */ 00043 struct vector_tag { }; 00044 00045 00046 /** 00047 * \class Extremum Extremum.h "tvmet/Extremum.h" 00048 * \brief Generell class for storing extremums determined by min/max. 00049 */ 00050 template<class T1, class T2, class Tag> 00051 class Extremum { }; 00052 00053 00054 /** 00055 * \class Extremum<T1, T2, vector_tag> Extremum.h "tvmet/Extremum.h" 00056 * \brief Partial specialzed for vectors to store extremums by value and index. 00057 */ 00058 template<class T1, class T2> 00059 class Extremum<T1, T2, vector_tag> 00060 { 00061 public: 00062 typedef T1 value_type; 00063 typedef T2 index_type; 00064 00065 public: 00066 Extremum(value_type value, index_type index) 00067 : m_value(value), m_index(index) { } 00068 value_type value() const { return m_value; } 00069 index_type index() const { return m_index; } 00070 00071 private: 00072 value_type m_value; 00073 index_type m_index; 00074 }; 00075 00076 00077 /** 00078 * \class Extremum<T1, T2, matrix_tag> Extremum.h "tvmet/Extremum.h" 00079 * \brief Partial specialzed for matrix to store extremums by value, row and column. 00080 */ 00081 template<class T1, class T2> 00082 class Extremum<T1, T2, matrix_tag> 00083 { 00084 public: 00085 typedef T1 value_type; 00086 typedef T2 index_type; 00087 00088 public: 00089 Extremum(value_type value, index_type row, index_type col) 00090 : m_value(value), m_row(row), m_col(col) { } 00091 value_type value() const { return m_value; } 00092 index_type row() const { return m_row; } 00093 index_type col() const { return m_col; } 00094 00095 private: 00096 value_type m_value; 00097 index_type m_row, m_col; 00098 }; 00099 00100 00101 } // namespace tvmet 00102 00103 #endif // TVMET_EXTREMUM_H 00104 00105 // Local Variables: 00106 // mode:C++ 00107 // tab-width:8 00108 // End:
Generated on Wed Jul 13 2022 18:28:36 by
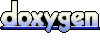