
School project.
Dependencies: Timezone NTPClient BSP_DISCO_F746NG Grove_temperature
setup_functions.h
00001 /** 00002 @file setup_functions.h 00003 @author Tu Tri Huynh 00004 @date January 13, 2021 00005 @brief Functions used for initial setup of the unit, specifically to set the building and room where unit installation is planned. 00006 */ 00007 00008 /** 00009 Declaration of the function (prototype) to allow other functions to call it 00010 @date 1/13/2021 00011 */ 00012 void setup_set_room_and_building(); 00013 00014 /** 00015 Prompt user for confirmation if happy with inputted information 00016 1/13/2021 00017 */ 00018 void setup_confirm() 00019 { 00020 char answer; 00021 printf("Type y for Yes, n to redo: "); 00022 scanf("%1s",&answer); 00023 if (answer == 'y' || answer == 'Y') 00024 { 00025 printf("Setup successfully completed.\n"); 00026 } 00027 else 00028 { 00029 /// This will empty the buffer and will avoid already inputted data to be used in scanf etc. 00030 int c; 00031 do { 00032 c = getchar(); 00033 } while (c != EOF && c != '\n'); 00034 00035 setup_set_room_and_building(); 00036 } 00037 /** 00038 printf("setup_confirm function end\n"); 00039 */ 00040 } 00041 00042 /** 00043 Show inputted information, and call setup_confirm to ask for confirmation. 00044 1/13/2021 00045 */ 00046 void setup_show_settings() 00047 { 00048 printf("Is this correct?\n"); 00049 printf("----------------------------\n"); 00050 printf("Building: %s\n", building); 00051 printf("Room: %s\n", room); 00052 printf("----------------------------\n"); 00053 setup_confirm(); 00054 } 00055 00056 /** 00057 Function to set building and room. Will call setup_show_settings to show what has been inputted. 00058 1/13/2021 00059 */ 00060 void setup_set_room_and_building() 00061 { 00062 char temp; 00063 /** 1/14/2021 00064 When using %s, string will be terminated when white space is found. 00065 Therefore, set it to read white spaces with %[^\n] 00066 Also, changed wording to reduce "please" sentences.*/ 00067 /** 00068 printf("Please set the building (maximum 30 characters): "); 00069 scanf("%30s", building); 00070 printf("Please set the room (maximum 30 characters): "); 00071 scanf("%30s", room); */ 00072 printf("Enter name of building (maximum 30 characters): "); 00073 scanf("%[^\n]", building); 00074 printf("Enter name of room (maximum 30 characters): "); 00075 /// temp statement to clear buffer 00076 scanf("%c", &temp); 00077 scanf("%[^\n]", room); 00078 00079 setup_show_settings(); 00080 } 00081 00082 /** 00083 Function to run the initial setup process. 00084 1/13/2021 00085 */ 00086 void setup_run_setup() 00087 { 00088 printf("\n*********************************************************\n"); 00089 printf("Welcome to the Light Control System initial setup process\n"); 00090 printf("Please set the units' location...\n\n"); 00091 setup_set_room_and_building(); 00092 }
Generated on Fri Jul 15 2022 03:26:25 by
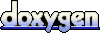