
School project.
Dependencies: Timezone NTPClient BSP_DISCO_F746NG Grove_temperature
main.cpp
00001 /** 00002 ****************************************************************************** 00003 @file main.cpp 00004 @author Tu Tri Huynh 00005 @date January 13, 2021 00006 @brief Light Control System to automatically dimmer or increase light levels 00007 @section Libraries used 00008 mbed-os version 6.6.0 00009 BSP_DISCO_F746NG, revision 13:85dbcff by Team ST 00010 Grove_temperature, v1.0.0 by Toyomasa Watarai 00011 NTPClient, revision 0:9282d46 by Zoltan Hudak 00012 Timezone, revision 0:38a95c8, originally by Jack Christensen and ported by Zoltan Hudak 00013 00014 @section Recommended hardware to run the program 00015 STM32F746G-DISCOVERY 00016 Grove Base Shield v2.1 00017 Grove Light Sensor v1.2 00018 Grove Rotary Angle Sensor v1.2 00019 Grove LED Socket Kit v1.5 00020 Grove LED diode 00021 Grove Button v1.2 00022 Grove Temperature Sensor v1.2 00023 ****************************************************************************** 00024 */ 00025 00026 #include "mbed.h" 00027 #include "Grove_temperature.h" 00028 #include "stm32746g_discovery_lcd.h" 00029 #include "EthernetInterface.h" 00030 #include "NTPClient.h" 00031 00032 AnalogIn light_sensor(A0); 00033 AnalogIn rotary(A1); 00034 DigitalIn button(D5); 00035 PwmOut led(D3); 00036 Grove_temperature temp(A2); 00037 EthernetInterface eth; 00038 NTPClient ntp(eth); 00039 /// Name of building to install the unit 00040 char building[31]; 00041 /// Name of room to install the unit 00042 char room[31]; 00043 00044 float light_sensor_reading; 00045 float rotary_reading; 00046 /// The percentage of light registered 00047 int light_intensity; 00048 /// The percentage of rotary turned 00049 int rotary_setting; 00050 00051 /// Used to determine if change in temperature has occured when new readings are performed 00052 int current_temp; 00053 /// Used to check if temperature unit should be switched or not 00054 bool switch_temp; 00055 bool is_fahrenheit = false; 00056 00057 00058 /*DigitalOut myled(LED1);*/ 00059 00060 #include "helper_functions.h" 00061 #include "setup_functions.h" 00062 #include "lcd_functions.h" 00063 #include "led_functions.h" 00064 #include "rotary_functions.h" 00065 #include "sc_functions.h" 00066 #include "button_functions.h" 00067 #include "main_functions.h" 00068 #include "time_functions.h" 00069 00070 00071 int main () 00072 { 00073 main_run_setup(); 00074 main_run_main_screen(); 00075 00076 Thread serial_communication; 00077 serial_communication.start(&sc_run_service); 00078 00079 Thread clock; 00080 clock.start(&time_update_current_time); 00081 00082 Thread button_check; 00083 button_check.start(&main_run_button_check); 00084 00085 while(1) 00086 { 00087 main_update_readings(); 00088 main_check_light_control(); 00089 00090 wait_us(1000000); 00091 00092 /** Code for testing temperature sensor 00093 1/18/2021 - Working 00094 */ 00095 /** 00096 printf("Current temperatur is: %2.2f\n", temp.getTemperature()); 00097 wait_us(1000000); 00098 */ 00099 00100 /** Code for testing that the rotary can control the LED light blinking 00101 1/14/2021 Working 00102 */ 00103 /** 00104 rotary_reading = rotary_reverse_read(rotary); 00105 printf("%1.2f\n",rotary_reading); 00106 led_set_blink_rate(rotary_reading); 00107 wait_us(2000000); 00108 */ 00109 00110 /** Code for testing if rotary has been turned or not 00111 1/14/2021 Working 00112 */ 00113 /** 00114 if (rotary_is_on(rotary)) 00115 { 00116 printf("Rotary is on\n"); 00117 } 00118 else 00119 { 00120 printf("Rotary is off\n"); 00121 } 00122 wait_us(1000000); 00123 */ 00124 00125 /** Code for testing sensor readings 00126 1/14/2021 Working 00127 */ 00128 /** 00129 light_sensor_reading = light_sensor.read(); 00130 rotary_reading = rotary.read(); 00131 printf("Light intensity: %i%%\n", helper_get_sensor_read_in_percent(light_sensor_reading)); 00132 printf("Rotary turned: %i%%\n", helper_get_sensor_read_in_percent(rotary_reading)); 00133 led_set_blink_rate(light_sensor_reading); 00134 wait_us(1000000); 00135 */ 00136 00137 /** 1/13/2021 Used to test the controller and make sure that it functions.*/ 00138 /** 00139 printf("This is a test.\n"); 00140 myled = 1; 00141 wait_us(1000000); 00142 myled = 0; 00143 wait_us(1000000); 00144 */ 00145 00146 /** 00147 printf("Mbed OS version: %d,%d,%d\n\n",MBED_MAJOR_VERSION, MBED_MINOR_VERSION, MBED_PATCH_VERSION); 00148 */ 00149 } 00150 }
Generated on Fri Jul 15 2022 03:26:25 by
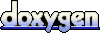