
School project.
Dependencies: Timezone NTPClient BSP_DISCO_F746NG Grove_temperature
lcd_functions.h
00001 /** 00002 @file lcd_functions.h 00003 @author Tu Tri Huynh 00004 @date January 13, 2021 00005 @brief This file contains functions for the LCD display. The display is visually divided into four equal parts to output different things. 00006 @section Useful information 00007 LCD size is (480, 272) 00008 Available font sizes are Font8, Font12, Font16, Font20 or Font24 00009 */ 00010 00011 /** 00012 This function will initialize the LCD display for use 00013 1/13/2021 00014 */ 00015 void lcd_initialize() 00016 { 00017 BSP_LCD_Init(); 00018 BSP_LCD_LayerDefaultInit(LTDC_ACTIVE_LAYER, LCD_FB_START_ADDRESS); 00019 BSP_LCD_SelectLayer(LTDC_ACTIVE_LAYER); 00020 } 00021 00022 00023 00024 /** 00025 This function will run a screen in LCD display that tells user to configure the unit 00026 1/13/2021 00027 */ 00028 void lcd_show_setup_screen() 00029 { 00030 /// Clears the LCD with specified color 00031 BSP_LCD_Clear(LCD_COLOR_BLACK); 00032 BSP_LCD_SetBackColor(LCD_COLOR_BLACK); 00033 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00034 BSP_LCD_SetFont(&Font24); 00035 BSP_LCD_DisplayStringAt(0, 50, (uint8_t *)"SETUP", CENTER_MODE); 00036 BSP_LCD_SetFont(&Font16); 00037 BSP_LCD_DisplayStringAt(0, 200, (uint8_t *)"Please configure the unit", CENTER_MODE); 00038 } 00039 00040 /** 00041 This function will run the startup screen with project- and developername 00042 1/13/2021 00043 */ 00044 void lcd_show_startup_screen() 00045 { 00046 BSP_LCD_Clear(LCD_COLOR_BLACK); 00047 BSP_LCD_SetBackColor(LCD_COLOR_BLACK); 00048 BSP_LCD_SetTextColor(LCD_COLOR_ORANGE); 00049 BSP_LCD_DisplayStringAt(0, 100, (uint8_t *)"Light Control System", CENTER_MODE); 00050 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00051 BSP_LCD_DisplayStringAt(0, 200, (uint8_t *)"by Tu Tri Huynh", CENTER_MODE); 00052 HAL_Delay(3000); 00053 } 00054 00055 /** 00056 Sets up the upper left part of screen 00057 1/18/2021 00058 */ 00059 void lcd_upper_left() 00060 { 00061 BSP_LCD_SetTextColor(LCD_COLOR_ORANGE); 00062 BSP_LCD_FillRect(0, 0, 240, 136); 00063 } 00064 00065 /** 00066 Update the content of the upper left part of display. 00067 1/18/2021 00068 */ 00069 void lcd_update_upper_left(char current_time[]) 00070 { 00071 BSP_LCD_SetBackColor(LCD_COLOR_ORANGE); 00072 BSP_LCD_SetTextColor(LCD_COLOR_BLUE); 00073 BSP_LCD_SetFont(&Font24); 00074 BSP_LCD_DisplayStringAt(50, 55, (uint8_t *)current_time, LEFT_MODE); 00075 BSP_LCD_SetTextColor(LCD_COLOR_ORANGE); 00076 /// Used to hide some weird artifacts in time string 00077 BSP_LCD_FillRect(185, 52, 30, 30); 00078 } 00079 00080 /** 00081 Sets up the upper right part of display 00082 1/18/2021 00083 */ 00084 void lcd_upper_right() 00085 { 00086 BSP_LCD_SetBackColor(LCD_COLOR_WHITE); 00087 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00088 BSP_LCD_FillRect(240, 0, 240, 136); 00089 BSP_LCD_SetBackColor(LCD_COLOR_WHITE); 00090 BSP_LCD_SetTextColor(LCD_COLOR_DARKYELLOW); 00091 BSP_LCD_SetFont(&Font20); 00092 BSP_LCD_DisplayStringAt(5, 16, (uint8_t *)"Light settings", RIGHT_MODE); 00093 } 00094 00095 /** 00096 Update the content of the upper right part of display. 00097 @param light_reading The intensity level of registered light in percentage 00098 @param rotary_reading The current position of rotary sensor in percentage 00099 1/18/2021 00100 */ 00101 void lcd_update_upper_right(int light_reading, int rotary_reading) 00102 { 00103 BSP_LCD_SetBackColor(LCD_COLOR_WHITE); 00104 BSP_LCD_SetTextColor(LCD_COLOR_DARKYELLOW); 00105 BSP_LCD_SetFont(&Font16); 00106 char light_to_lcd[30]; 00107 char rotary_to_lcd[30]; 00108 sprintf(light_to_lcd, "Light intensity: %3i%%", light_reading); 00109 sprintf(rotary_to_lcd, "Rotary turned: %3i%%", rotary_reading); 00110 00111 BSP_LCD_DisplayStringAt(0, 46, (uint8_t *)light_to_lcd, RIGHT_MODE); 00112 BSP_LCD_DisplayStringAt(0, 70, (uint8_t *)rotary_to_lcd, RIGHT_MODE); 00113 } 00114 00115 /** 00116 Sets up the lower left part of display 00117 1/13/2021 00118 */ 00119 void lcd_lower_left() 00120 { 00121 BSP_LCD_SetBackColor(LCD_COLOR_DARKMAGENTA); 00122 BSP_LCD_SetTextColor(LCD_COLOR_DARKMAGENTA); 00123 BSP_LCD_FillRect(0, 136, 240, 136); 00124 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00125 BSP_LCD_SetFont(&Font20); 00126 BSP_LCD_DisplayStringAt(5, 160, (uint8_t *)"Location", LEFT_MODE); 00127 } 00128 00129 /** 00130 Updates the content of the lower left area of the display 00131 @param building[] Name of the building 00132 @param room[] Name of the room 00133 1/18/2021 00134 */ 00135 void lcd_update_lower_left(char building[], char room[]) 00136 { 00137 BSP_LCD_SetBackColor(LCD_COLOR_DARKMAGENTA); 00138 BSP_LCD_SetTextColor(LCD_COLOR_WHITE); 00139 BSP_LCD_SetFont(&Font16); 00140 BSP_LCD_DisplayStringAt(35, 200, (uint8_t *)building, LEFT_MODE); 00141 BSP_LCD_DisplayStringAt(35, 220, (uint8_t *)room, LEFT_MODE); 00142 } 00143 00144 /** 00145 Sets up the lower right part of display 00146 1/18/2021 00147 */ 00148 void lcd_lower_right() 00149 { 00150 BSP_LCD_SetTextColor(LCD_COLOR_CYAN); 00151 BSP_LCD_FillRect(240, 136, 240, 136); 00152 BSP_LCD_SetBackColor(LCD_COLOR_CYAN); 00153 BSP_LCD_SetTextColor(LCD_COLOR_ORANGE); 00154 BSP_LCD_SetFont(&Font20); 00155 BSP_LCD_DisplayStringAt(5, 160, (uint8_t *)"Temperature", RIGHT_MODE); 00156 BSP_LCD_SetFont(&Font16); 00157 } 00158 00159 /** Update the content of the lower right part of display. 00160 @param temp The temperature reading from the thermal sensor 00161 @param is_fahrenheit The bool value to determine if temperature unit should switch to fahrenheit or not 00162 1/18/2021 00163 */ 00164 void lcd_update_lower_right(float temp, bool is_fahrenheit) 00165 { 00166 char temp_text[30]; 00167 if (is_fahrenheit == true) 00168 { 00169 float fahrenheit = helper_convert_celsius_to_fahrenheit(temp); 00170 sprintf(temp_text, "%3.0f F", fahrenheit); 00171 } 00172 else 00173 { 00174 sprintf(temp_text, "%2.0f C", temp); 00175 } 00176 BSP_LCD_SetBackColor(LCD_COLOR_CYAN); 00177 BSP_LCD_SetTextColor(LCD_COLOR_ORANGE); 00178 BSP_LCD_SetFont(&Font24); 00179 BSP_LCD_DisplayStringAt(35, 210, (uint8_t *)temp_text, RIGHT_MODE); 00180 BSP_LCD_DrawCircle(421, 214, 3); 00181 BSP_LCD_SetFont(&Font16); 00182 } 00183 00184 /** 00185 This function will show the default main screen on display 00186 1/19/2021 00187 */ 00188 void lcd_show_main_screen() 00189 { 00190 BSP_LCD_Clear(LCD_COLOR_DARKBLUE); // Clear with color 00191 lcd_upper_left(); 00192 lcd_lower_left(); 00193 lcd_lower_right(); 00194 lcd_upper_right(); 00195 } 00196 00197 /** 00198 This function will print LCD size in console terminal 00199 1/13/2021 00200 */ 00201 void lcd_get_screen_size() 00202 { 00203 printf("LCD X size : %zu\n\r",BSP_LCD_GetXSize()); //result: 480 00204 printf("LCD Y size : %zu\n\r",BSP_LCD_GetYSize()); //result: 272 00205 }
Generated on Fri Jul 15 2022 03:26:25 by
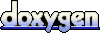