
School project.
Dependencies: Timezone NTPClient BSP_DISCO_F746NG Grove_temperature
helper_functions.h
00001 /** 00002 @file helper_functions.h 00003 @author Tu Tri Huynh 00004 @date January 14, 2021 00005 @brief Functions to help with general tasks. 00006 */ 00007 00008 /** 00009 This function returns the percentage of AnalogIn sensor read values that by default are floating point numbers between 0.00 to 1.00. 00010 @param read_value Float value from the read() function in analog units 00011 @return whole number that represents percentage. 00012 1/14/2021 00013 */ 00014 int helper_get_sensor_read_in_percent(float read_value) 00015 { 00016 return (int)(read_value*100); 00017 } 00018 00019 /** 00020 This function returns a rounded float value, used for temperature readings. 00021 @param temp Temperature value to be rounded 00022 */ 00023 float helper_round_temperature(float temp) 00024 { 00025 return floorf(temp * 100)/100; 00026 } 00027 00028 /** 00029 This function converts Celsius temperature to Fahrenheit. 00030 @param temp Celsius value 00031 */ 00032 float helper_convert_celsius_to_fahrenheit(float temp) 00033 { 00034 float fahrenheit = ((temp * 9)/5)+32; 00035 return fahrenheit; 00036 } 00037 00038 /** 00039 This function reverses the bool value. 00040 @@param value Bool value to reverse. 00041 1/18/2021 00042 */ 00043 bool helper_reverse_bool(bool value) 00044 { 00045 return !value; 00046 }
Generated on Fri Jul 15 2022 03:26:25 by
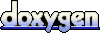