
Test program with networking part in RTOS-thread
Dependencies: mbed mbed-rtos EthernetInterface
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "cmsis_os.h" 00004 00005 DigitalOut led1(LED1); 00006 DigitalOut led2(LED2); 00007 DigitalOut led3(LED3); 00008 00009 void led2_thread(void const *argument) { 00010 while (true) { 00011 led2 = !led2; 00012 osDelay(1000); 00013 } 00014 } 00015 void net_thread(void const *argument) { 00016 EthernetInterface eth; 00017 eth.init(); //Use DHCP 00018 eth.connect(); 00019 printf("IP Address is %s\n", eth.getIPAddress()); 00020 TCPSocket sock; 00021 while (true) { 00022 led3 = !led3; 00023 sock.connect("mbed.org", 80); 00024 00025 char http_cmd[] = "GET /media/uploads/donatien/hello.txt HTTP/1.1\r\nHost: %s\r\n\r\n"; 00026 sock.send(http_cmd, sizeof(http_cmd) - 1, 3000); 00027 00028 char in_buf[256]; 00029 bool firstIteration = true; 00030 int ret; 00031 do { 00032 ret = sock.receive(in_buf, 255, firstIteration?3000:0); 00033 in_buf[ret] = '\0'; 00034 00035 printf("Received %d chars from server: %s\n", ret, in_buf); 00036 firstIteration = false; 00037 } while ( ret > 0 ); 00038 00039 sock.close(); 00040 00041 //eth.disconnect(); 00042 00043 led3 = !led3; 00044 osDelay(500); 00045 } 00046 } 00047 00048 osThreadDef(led2_thread, osPriorityNormal, DEFAULT_STACK_SIZE); 00049 osThreadDef(net_thread, osPriorityNormal, DEFAULT_STACK_SIZE); 00050 00051 int main() { 00052 osThreadCreate(osThread(led2_thread), NULL); 00053 00054 osThreadCreate(osThread(net_thread), NULL); 00055 00056 while (true) { 00057 led1 = !led1; 00058 osDelay(500); 00059 } 00060 } 00061
Generated on Mon Jul 18 2022 22:22:25 by
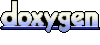