
thread based dc motor direction
Dependencies: Motor mbed-rtos mbed
Fork of rtos_basic by
main.cpp
00001 #include "mbed.h" 00002 #include "Motor.h" 00003 #include "rtos.h" 00004 00005 AnalogIn inputx(p20); // input pins 20,19,18 for x,y,z axis respectively. 00006 AnalogIn inputy(p19); 00007 DigitalOut led1(LED1); 00008 DigitalOut led2(LED2); 00009 DigitalOut led3(LED3); 00010 Motor m1(p23,p5,p6); // pwm, fwd, rev 00011 //Motor m2(p22,p11,p12); // pwm,fwd,rev 00012 00013 Serial pc(USBTX,USBRX); //Serial class for transmission of serial data 00014 Thread thread; 00015 00016 void led2_thread() { 00017 //while (true) { 00018 00019 led2 = !led2; 00020 pc.printf("thread2 executed"); 00021 Thread::wait(500); 00022 00023 //} 00024 00025 } 00026 00027 void led1_thread() { 00028 // while(true) { 00029 00030 led1 = !led1; 00031 pc.printf("thread1 executed"); 00032 00033 Thread::wait(500); 00034 00035 // } 00036 } 00037 00038 void led3_thread() { 00039 //while (true) { 00040 pc.printf("invalid"); 00041 led3 = !led3; 00042 //m2.speed(0); 00043 Thread::wait(500); 00044 // } 00045 } 00046 00047 00048 int main() 00049 { 00050 pc.baud(9600); // fixing a constant baud rate of 9600 bps at which mbed will interact with computer 00051 float cal_x,cal_y; //caliberation variables 00052 int x,y; // variables for x,y,z axes 00053 int i=100; 00054 while(i--) //small timed loop to caliberate the accelerometer with currentl position of the accelerometer 00055 { 00056 cal_x=inputx*100; 00057 cal_y=inputy*100; 00058 00059 } 00060 while(true) 00061 { 00062 Thread::wait(500); 00063 x=4*(cal_x - inputx*100); 00064 y=4*(cal_y - inputy*100); 00065 00066 00067 y=(y-30); 00068 pc.printf("x=%d,y=%d *",x,y); 00069 pc.printf("\n"); 00070 00071 if(x >= 0 && y >=0) 00072 { 00073 pc.printf("\n Anticlockwise\n"); 00074 for (float s= 1.0; s < 10.0 ; s += 0.01) 00075 m1.speed(s); // clockwise 00076 thread.start(led1_thread); 00077 } 00078 00079 00080 else if (x <= 0 && y <=0) 00081 { 00082 pc.printf("\n Clockwise \n"); 00083 for(float s= -1.0; s > -10.0 ; s += -0.01) 00084 m1.speed(s); 00085 00086 // anticlockwise //Anticlockwise 00087 // led1_thread(); 00088 thread.start(led2_thread); 00089 } 00090 00091 else if((x<=0 && y>=0)|| (x>=0 && y<=0)) 00092 { 00093 pc.printf(""); 00094 m1.speed(0); //stop 00095 thread.start(led3_thread); 00096 } 00097 00098 Thread::wait(500); 00099 00100 //passing the caliberated x,y,z values to the computer. 00101 } 00102 00103 }
Generated on Tue Aug 2 2022 03:57:18 by
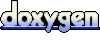